How to Sort a Collection Using Multiple Fields in Kotlin
- Generate a Kotlin Project
- Sort Collection Using Individual Properties
- Sort Collection Using Multiple Properties
- Conclusion
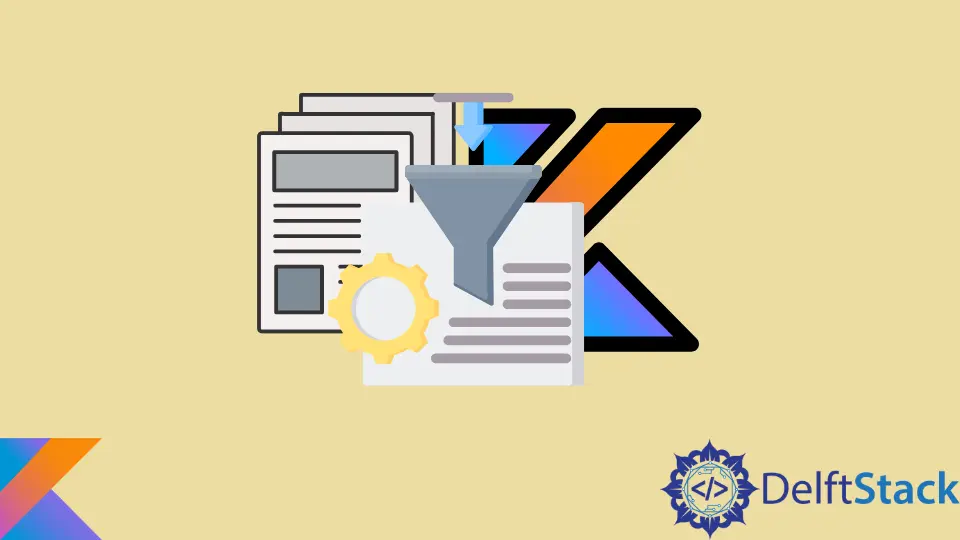
The Kotlin library provides different ways the developer can leverage based on the requirement. This tutorial will teach us how to sort a collection using individual and multiple fields.
Generate a Kotlin Project
Open IntelliJ development environment and select File > New > Project
. Enter the project name as kotlin-sortby
, select Kotlin
on the language section, and select Intellij
on the Build system
section.
Lastly, press the Create
button to generate the project. Create a file named Main.kt
under the src/main/kotlin
folder and copy and paste the following code into the file.
Code:
import java.time.Year
data class Book(val id: Int,
val name: String,
val yearPublished: Year)
fun fetchBooks(): ArrayList<Book>{
return arrayListOf(
Book(1,"Java Arrays", Year.of(1984)),
Book(2,"Python Arrays",Year.of(2021)),
Book(3,"Pascal for loop",Year.of(2022)),
Book(4,"Pascal Arrays",Year.of(2021)),
Book(5,"Java for loop",Year.of(2022))
)
}
In this code, we’ve created a method named fetchBooks()
that returns a List
of book objects created using the Book
data class. We use this list in our examples to see how we can sort the books based on different criteria.
Sort Collection Using Individual Properties
Copy and paste the following example codes into the Main.kt
file after the fetchBooks()
method.
Use the sortBy()
Method to Sort a Collection
In the following example, we’ll create a method named usingSortBy()
that uses the sortBy()
method to sort our previously created list using the yearPublished
field.
The sortBy()
method is an extension function of the MutableList
and does not return any value. The function has a crossinline
keyword before the selector function that is applied to it, which means that the selector function does not allow non-local returns.
Note that the selector function uses the Comparable
interface behind the scenes to sort the elements in the list.
Code:
fun usingSortBy(){
val books = fetchBooks()
books.sortBy(Book::yearPublished)
books.forEach(::println);
}
fun main(){
usingSortBy();
}
Output:
Book(id=1, name=Java Arrays, yearPublished=1984)
Book(id=2, name=Python Arrays, yearPublished=2021)
Book(id=4, name=Pascal Arrays, yearPublished=2021)
Book(id=3, name=Pascal for loop, yearPublished=2022)
Book(id=5, name=Java for loop, yearPublished=2022)
Use the sortedBy()
Method to Sort a Collection
The collections framework provides an alternative to the sortBy()
method that allows us to return a new list we could not get in this example. We can use the sortedBy()
method, an extension function of the Iterable
interface.
Code:
fun usingSortedBy(){
fetchBooks().sortedBy(Book::yearPublished)
.forEach(::println);
}
fun main(){
usingSortedBy();
}
Output:
Book(id=1, name=Java Arrays, yearPublished=1984)
Book(id=2, name=Python Arrays, yearPublished=2021)
Book(id=4, name=Pascal Arrays, yearPublished=2021)
Book(id=3, name=Pascal for loop, yearPublished=2022)
Book(id=5, name=Java for loop, yearPublished=2022)
The sortedBy()
method returns a list containing the elements sorted using the selector function, and we can invoke the forEach()
method on the returned list to log the objects to the console.
Sort Collection Using Multiple Properties
We’ve seen how to sort elements using a single field in previous examples. However, there are situations where the client might want to sort based on multiple fields in an application.
In this section, we will learn how to realize this functionality.
Use the sortWith()
Method to Sort a Collection
In this example, we’ve created a method named usingSortWith()
that uses the sortWith()
method to sort our list using the name
of the book followed by the year
of the book.
The sortWith()
method is an extension function of the MutableList
and accepts any argument that returns a Comparator
. Note that this method does not return any value.
Code:
fun usingSortWith(){
val books = fetchBooks();
books.sortWith(compareBy(Book::name, Book::yearPublished));
books.forEach(::println);
}
fun main(){
usingSortWith();
}
We have used the compareBy()
method as the argument for the sortWith()
method. The compareBy()
method returns a Comparator
and can be overloaded with more than one selector function to sort the list based on different properties.
The vararg
keyword added before the selector function definition indicates that we can pass more than one argument.
Output:
Book(id=1, name=Java Arrays, yearPublished=1984)
Book(id=5, name=Java for loop, yearPublished=2022)
Book(id=4, name=Pascal Arrays, yearPublished=2021)
Book(id=3, name=Pascal for loop, yearPublished=2022)
Book(id=2, name=Python Arrays, yearPublished=2021)
Use the sortedWith()
Method to Sort a Collection
The collections framework provides an alternative to the sortWith()
method that allows us to return a new list containing the sorted elements. We can use the sortedWith()
method to realize this functionality.
The sortedWith()
method is an extension function of the Iterable
interface and accepts any argument that returns a Comparator
.
Code:
fun usingSortedWith(){
fetchBooks().sortedWith(compareBy(Book::name, Book::yearPublished))
.forEach(::println);
}
fun main(){
usingSortedWith();
}
We have used the compareBy()
that returns a Comparator
as we have in the previous example and overloaded it to sort the list using name and year.
Output:
Book(id=1, name=Java Arrays, yearPublished=1984)
Book(id=5, name=Java for loop, yearPublished=2022)
Book(id=4, name=Pascal Arrays, yearPublished=2021)
Book(id=3, name=Pascal for loop, yearPublished=2022)
Book(id=2, name=Python Arrays, yearPublished=2021)
Conclusion
In this tutorial, we’ve learned different ways we can use to sort a list based on desired criteria. The approaches we have covered include using the sortBy()
method, using the sortedBy()
method, using the sortWith()
method, and using the sortedWith()
method.
Note that the other two sorting strategies allow us to sort a list in descending order, and they include sortByDescending()
and sortedByDescending()
.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub