How to Parse JSON String to Object in Kotlin
- Create a New Project and Add Dependencies
-
Parse JSON String to Object in Kotlin Using the
kotlinx.serialization
Library -
Parse JSON String to Object in Kotlin Using the
org.json
Library - Parse JSON String to Object in Kotlin Using the Gson Library
- Parse JSON String to Object in Kotlin Using the Jackson Library
- Conclusion
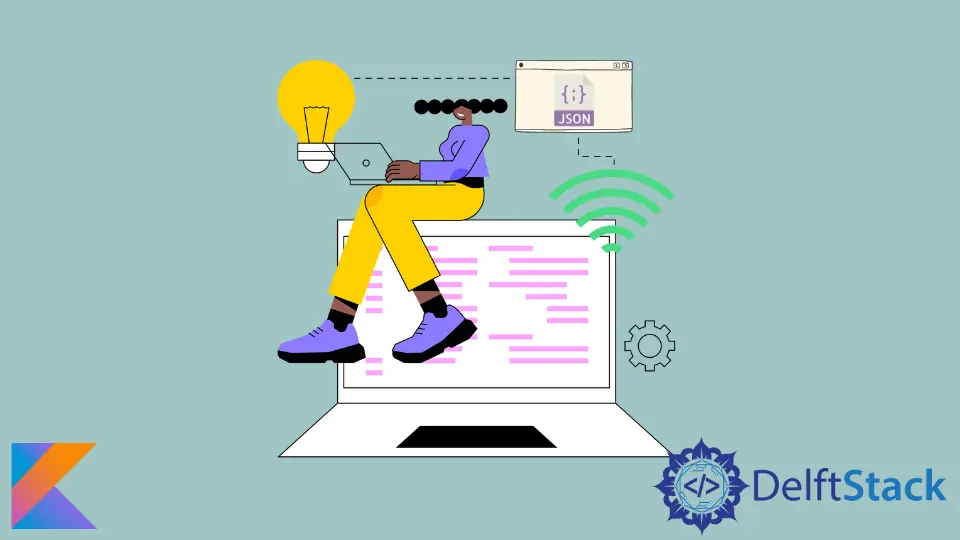
JSON stands for JavaScript Object Notation, a data interchange format used by client-server architectures to transfer data between computers or store data in a database.
The JSON data is consumed using REST APIs that issue HTTP requests to the servers to perform CRUD operations on the data stored in the database. For example, when we dynamically load data on a webpage or a mobile application, we usually retrieve this data from the database as JSON, parse the data into objects, and display each object variable to the view.
In this tutorial, we will learn the different ways we can use to parse JSON data to objects in Kotlin.
Create a New Project and Add Dependencies
To create a new project, go to Intellij and select File > New > Project
. On the window that opens, enter kotlinSerialization
as the project name, select Kotlin
in the Language section, and Gradle
in the Build System section.
Press the Create
button to generate a new project. Once the project is generated, go to the build.gradle.kts
file and ensure you have the following dependencies.
dependencies {
implementation ("com.fasterxml.jackson.module:jackson-module-kotlin:2.13.3")
implementation ("com.google.code.gson:gson:2.9.0")
implementation("org.json:json:20220320")
implementation("org.jetbrains.kotlinx:kotlinx-serialization-json:1.3.3")
testImplementation(kotlin("test"))
}
Each dependency provides APIs we use to create each implementation, so ensure you have all the dependencies to avoid the unresolved reference errors.
Parse JSON String to Object in Kotlin Using the kotlinx.serialization
Library
In this example, we need to ensure that we have the following plugins in the Plugins section of the build.gradle.kts
file.
plugins {
kotlin("jvm") version "1.7.0"
kotlin("plugin.serialization") version "1.7.0"
}
Create the folder structure com/serialize
under the kotlin
folder of the project where our Kotlin files will be created.
Create a file named UsingKotlinx.kt
under the serialize
folder, and copy and paste the following code into the file.
package com.serialize
import kotlinx.serialization.Serializable
import kotlinx.serialization.decodeFromString
import kotlinx.serialization.json.Json
val employeeJSON = """
{
"firstName": "john",
"lastName": "doe",
"email": "john@gmail.com"
}
""".trimIndent()
@Serializable
data class Employee(val firstName: String,
val lastName: String,
val email: String)
fun main(args: Array<String>){
//Decode employee from string
val employeeObject = Json.decodeFromString<Employee>(employeeJSON);
println(employeeObject);
}
In the above code, we have created a multiline text of our JSON object using Kotlin’s triple quoted text to represent our employee object. The trimIndent()
method removes a common indentation of all the input lines and removes the first and the last lines if they are blank.
We have then created an Employee
class and annotated it with the @Serializable
annotation. The Employee
class is a data class with the parameters firstName
, lastName
, and email
.
These parameters will store our data, hence the data
keyword. When we use a data class, we get some methods such as the toString()
out of the box without declaring them, and this is crucial when you want to log the data to the console.
The @Serializable
annotation makes the Employee
class serializable, which means we can change it to a format that can be stored in a database, a file, or transferred over the network.
To parse our JSON object to a Kotlin object, we invoke the decodeFromString()
generic method from Json
. Since we want to return an employee object, we pass the Employee
class to the type parameter and our JSON string as the method’s argument.
The print
statement logs the parsed object to the console. Run the code and note that the object is logged as a string to the console, as shown below.
Employee(firstName=john, lastName=doe, email=john@gmail.com)
Parse JSON String to Object in Kotlin Using the org.json
Library
In this example, we will utilize the JSONObject
API, which is provided by the org.json
library. Note that this library was included in our application when adding the dependencies.
Create a file named UsingJSONObject.kt
under the serialize
folder and copy and paste the following code into the file.
package com.serialize
import org.json.JSONObject
val usersArray = """
{
"User":[
{
"firstName": "john",
"lastName": "doe",
"email": "john@gmail.com"
},
{
"firstName": "mary",
"lastName": "public",
"email": "mary@gmail.com"
}
]
}
""".trimIndent()
class User{
var firstName: String? = null;
var lastName: String? = null;
var email: String? = null
override fun toString(): String {
return "User(firstName=$firstName, lastName=$lastName, email=$email)"
}
}
fun main() {
val userJsonObject =
JSONObject(usersArray)
val userObject = userJsonObject
.getJSONArray("User");
for (i in 0 until(userObject!!.length())){
val theUser = User();
val firstName = userObject
.getJSONObject(i)
.getString("firstName");
theUser.firstName = firstName
val lastName = userObject
.getJSONObject(i)
.getString("lastName")
theUser.lastName = lastName
val email = userObject
.getJSONObject(i)
.getString("email")
theUser.email = email;
println(theUser);
}
}
The first and the second steps are similar to the one we covered in the previous example, but this class creates an array of JSON objects and uses a normal Kotlin class. Since we are not using a data class in this example, we have to create our custom toString()
method using the fields firstName
, lastName
, and email
.
To retrieve the JSON array, we invoke the JSONObject()
constructor and pass the JSON string as the constructor’s argument. The constructor creates a JSON object from a string starting with {
and ending with }
; it throws a JSONException
if there is an error in the string.
Access to the JSON object allows us to retrieve any property we want by passing that property as an argument. To retrieve the array of Users, we invoke the getJSONArray()
method from the returned object and pass User
as the method’s argument.
The method returns a JSONArray
of users from the object.
Creating Kotlin objects from the JSONArray
is done manually using a loop. We retrieve each object from the array using the getJSONObject()
method by passing the object’s index as the method’s argument.
From this object, we retrieve each variable and assign it to a new Kotlin object created for each iteration. Run the application to verify it outputs the following string objects.
User(firstName=john, lastName=doe, email=john@gmail.com)
User(firstName=mary, lastName=public, email=mary@gmail.com)
Parse JSON String to Object in Kotlin Using the Gson Library
Create a UsingGson.kt
file under the serialize
folder and copy-paste the following code into the file.
package com.serialize
import com.google.gson.Gson
val customerObject = """
{
"firstName": "john",
"lastName": "doe",
"email": "john@gmail.com"
}
""".trimIndent()
class Customer{
private val firstName: String? = null;
private val lastName: String? = null;
private val email: String? = null;
override fun toString(): String {
return "Customer(firstName=$firstName, lastName=$lastName, email=$email)"
}
}
fun main() {
val theGson = Gson();
val customer = theGson
.fromJson(customerObject, Customer::class.java);
println(customer);
}
We are sure you have understood that the first step creates a JSON representation of our object, and the second step creates a class that will be used to create a Kotlin object from the string.
The main method invokes the Gson()
constructor to create a Gson object with the default configuration.
To map our JSON string to a Kotlin object, invoke the fromJson()
method from the Gson object and pass the JSON string as the method’s first argument and class
as the second parameter of the method.
Note that the fromJson()
method is generic and returns the type of object passed as the method’s second argument. The method is also overloaded to provide other implementations, such as reading the JSON object from a file.
Run the program and note that it logs the following string object to the console.
Customer(firstName=john, lastName=doe, email=john@gmail.com)
Parse JSON String to Object in Kotlin Using the Jackson Library
Create a file named UsingJackson.kt
under the serialize
folder and copy and paste the following code into the file.
package com.serialize
import com.fasterxml.jackson.module.kotlin.jacksonObjectMapper
val vehicleObject = """
{
"vehicleName": "Toyota",
"vehicleColor": "white",
"vehiclePrice": 10000
}
""".trimIndent()
data class Vehicle(private val vehicleName: String,
private val vehicleColor: String,
private val vehiclePrice: Int)
fun main() {
val jacksonMapper = jacksonObjectMapper();
val vehicle = jacksonMapper
.readValue(vehicleObject, Vehicle::class.java)
println(vehicle);
}
In this code, we have created a string representing our JSON object and a class that will be used to map the string to a Kotlin object. The properties created in the class must be the same as the keys in the JSON string.
To use the Jackson library, we invoke the jacksonObjectMapper()
in the main method to create an ObjectMapper
. Then, we call the readValue()
method of the ObjectMapper
and pass the JSON string as the first argument and the class
being mapped as the second argument.
The readValue()
method deserializes the JSON string and returns the type of object passed as the method’s second argument.
Run the code to verify it logs the following object as a string to the console.
Vehicle(vehicleName=Toyota, vehicleColor=white, vehiclePrice=10000)
Conclusion
In this tutorial, we have learned how to parse a JSON object to a Kotlin object. The approaches that we have covered include: using the kotlix.serialization
library, the org.json
library, the Gson
library, and the Jackson
library.
Note that these are not the only libraries we can use to realize the same objective, so feel free to implement your application with other libraries.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub