Kotlin Spread Operator
- Generate a Kotlin Project
- Pass Multiple Arguments in Kotlin
- Pass Multiple Arguments Using the Spread Operator in Kotlin
- Pass Multiple Arguments Using Primitive Type Array in Kotlin
- Conclusion
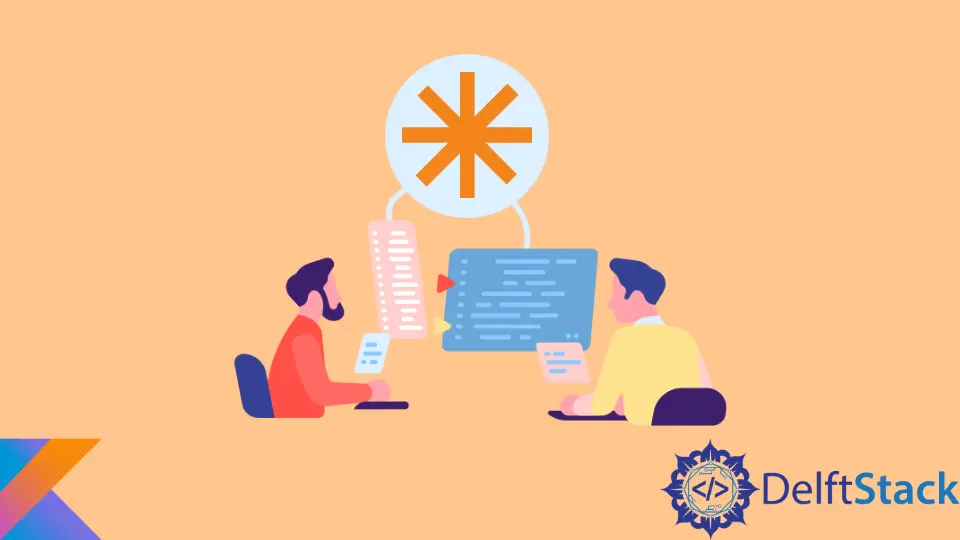
The spread operator (*
) is used in most programming languages such as Kotlin, TypeScript, JavaScript, and others.
The spread operator is used to pass multiple arguments in places where more than one argument is expected. In Kotlin, the spread operator can be used with function parameters prefixed with the vararg
keyword.
The vararg
keyword indicates that a function accepts a variable number of arguments and can be used with both generic and non-generic types. This tutorial will teach how the spread operator works and where it is used in Kotlin.
Generate a Kotlin Project
Open IntelliJ development environment and select File
> New
> Project
. Enter the project name as kotlin-spread-operator
, select Kotlin
on the Language
section, and select Intellij
on the Build system
section.
Finally, press the Create
button to generate the project. After the project has opened, create a file named Main.kt
under the src/main/kotlin
folder and copy and paste the following code into the file.
class House(
private val id: Int,
private val houseType: String,
private val houseLocation: String){
companion object{
fun <T> of(vararg houses: T): List<T>{
val theHouses = ArrayList<T>();
for (house in houses) {
theHouses.add(house);
}
return theHouses;
}
}
fun getId(): Int{
return this.id;
}
override fun toString(): String {
return "House(id=$id, houseType='$houseType', houseLocation='$houseLocation')"
}
}
In this code, we have created a class named House
with the properties id
, houseType
, and houseLocation
that must be provided when creating an object.
We have also defined three methods named of()
, getId()
, and toString()
. The of()
method is defined inside a companion object to indicate that it is a static method that can be invoked without creating any object.
The getId()
method returns the id
of the current object, and the toString()
method helps us to get a string representation of our object. We will use this class in the next sections to create instances that we will use in our examples.
Pass Multiple Arguments in Kotlin
Copy and paste the following code into the Main.kt
file after the House
class declaration.
fun multipleArgumentFunction(){
val bungalow = House(1,"Bungalow","Seattle");
val flat = House(2,"Flat","Texas")
val houses: List<House> = House.of(bungalow, flat);
houses.forEach(::println);
}
fun main(){
multipleArgumentFunction();
}
In this code, we have created a method that creates two house objects and returns a list of these objects by passing the objects as the arguments of the of()
method.
You might wonder why we passed two arguments while providing one parameter when defining the static of()
method. Recall that we mentioned a method that could accept more than one argument if it has the vararg
keyword.
Note that the static of()
method has the vararg
keyword, and thus it can accept more than one argument. Run this code and ensure the output is as shown below.
House(id=1, houseType='Bungalow', houseLocation='Seattle')
House(id=2, houseType='Flat', houseLocation='Texas')
Pass Multiple Arguments Using the Spread Operator in Kotlin
We have mentioned that a spread operator is used to pass multiple arguments where more than one argument is expected, and a spread operator is usually indicated using the *
operator, which is usually prefixed on the object containing the array of data.
Comment on the previous code and copy and paste the following code into the Main.kt
file after the comment.
fun multipleArgumentFunctionWithSpreadOperator(){
val bungalow = House(1,"Bungalow","Seattle");
val flat = House(2,"Flat","Texas")
val apartments: Array<House> = arrayOf(
House(3,"Apartment","San Francisco"),
House(4,"Apartment","New York"),
House(5,"Apartment","Florida")
);
val theHouses: List<House> = House.of(bungalow, flat, *apartments);
theHouses.forEach(::println);
}
fun main(){
multipleArgumentFunctionWithSpreadOperator();
}
This example builds up on the previous example, and in this code, we have added an Array
that contains three house objects.
The of()
method allows us to pass a variable number of arguments; we pass this array to the method using the spread operator.
Note that you can also pass other individual objects to the method.
We use the syntax *apartments
to pass the array to the method, and all the objects in the array are available to the code inside the method. Run the following code and ensure the output is as shown below.
House(id=1, houseType='Bungalow', houseLocation='Seattle')
House(id=2, houseType='Flat', houseLocation='Texas')
House(id=3, houseType='Apartment', houseLocation='San Francisco')
House(id=4, houseType='Apartment', houseLocation='New York')
House(id=5, houseType='Apartment', houseLocation='Florida')
Pass Multiple Arguments Using Primitive Type Array in Kotlin
Kotlin provides primitive type arrays that include IntArray
, ShortArray
, ByteArray
, LongArray
, and others.
If we are using these types in our application, we are prone to getting Type mismatch
errors, and this example shows how to solve this.
Comment on the previous code and copy and paste the following code into the Main.kt
file after the comment.
fun multipleArgumentFunctionWithTypedArray(){
val bungalow = House(1,"Bungalow","Seattle");
val flat = House(2,"Flat","Texas")
val apartments: Array<House> = arrayOf(
House(3,"Apartment","San Francisco"),
House(4,"Apartment","New York"),
House(5,"Apartment","Florida")
);
val apartmentCount: IntArray =
apartments.map(House::getId).toIntArray();
val totalHouses: List<Int> =
House.of(bungalow.getId(),flat.getId(), *apartmentCount.toTypedArray());
totalHouses.forEach(::println);
}
fun main(){
multipleArgumentFunctionWithTypedArray();
}
In this example, our objective is to return all the id
passed to the of()
method as a collection of integers List<Int>
.
The map()
method uses the array of house objects Array<House>
to return the primitive type array IntArray
using the toIntArray()
method.
To return all the id
of our objects, we have passed the id
of individual objects to the of()
method using the getId()
method.
We have passed the remaining object id
to the of()
method using a spread operator. However, we first converted the primitive type array IntArray
to a regular typed array Array<Int>
using the toTypedArray()
method to prevent the Type mismatch
error.
Run this code and ensure the output is as shown below.
1
2
3
4
5
Conclusion
In this tutorial, we have learned how to use the spread operator in Kotlin, and the topics we have covered include how to pass multiple arguments using the spread operator and how to pass multiple arguments using the spread operator. Finally, we covered how to pass multiple arguments using a primitive type array.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub