How to Get a Random Number in Kotlin
-
Use the
random()
Extension Function ofIntRange
in Kotlin -
Use
random()
With Range Inclusive in Kotlin -
Use
random()
With Range Exclusive in Kotlin -
Use the
shuffled()
Extension Function ofIterable
in Kotlin -
Use
ThreadLocalRandom
in Kotlin - Conclusion
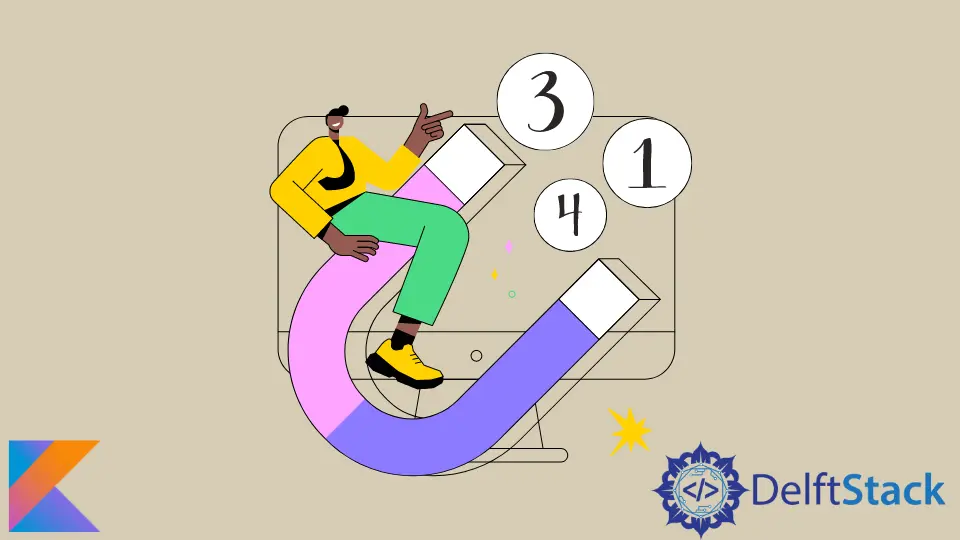
A random number is generated from a set of numbers using an algorithm that ensures each number has an equal probability of being generated.
We can generate different types of data types apart from numbers. For example, we can use the UUID.randomUUID()
method of java.util
to generate a random string.
This feature of allowing the developer to generate random strings is very important as it helps to generate unique identifiers for data, generate unique passwords, and generate an order tracking identifier in an eCommerce environment.
This tutorial will demonstrate how we can leverage to generate a random number using Kotlin.
Use the random()
Extension Function of IntRange
in Kotlin
An extension function is a way to add functionality to a class without modifying the existing code or using design patterns such as the decorator pattern.
Go to the IntelliJ development environment and select File
> New
> Project
. On the window that opens, enter the project name
as kotlin-random
, select Kotlin
on the Language
section, select Intellij
on the Build System
section, and finally press the Create
button to generate a new project.
Use random()
With Range Inclusive in Kotlin
Create a Main.kt
file under the kotlin
folder and copy and paste the following code into the file.
fun exampleOne(): Int{
return (0..10).random();
}
fun main(){
println("... Example One ...")
println(exampleOne());
}
In this code, we have created a method named exampleOne()
that returns an Int
. The integer is randomly generated by the random()
function in the method.
The random()
function is an extension function of IntRange
and returns a randomly generated element from the provided range.
If the range is empty, the method throws an IllegalArgumentException
. A range is empty when the first element of the range is greater than the last element of the range.
The random()
function uses the nextInt()
extension function of Random
behind the scenes to generate a number that is uniformly distributed within the provided range.
The number generated can be any number from 0
to 10
inclusive. If you run the above code multiple times, you will notice that you will get any random number in the range.
Output:
... Example One ...
3
Use random()
With Range Exclusive in Kotlin
Comment out the previous example and copy and paste the following code into the Main.kt
file after it.
fun exampleTwo(): Int{
return (0 until 10).random();
}
fun main(){
println("... Example Two ...")
println(exampleTwo());
}
In the previous example, we used ..
in the range, which is used to denote the rangeTo()
method. However, in this example, we have used until
, which denotes the Int.until()
extension function.
The until()
extension functions return a range from the start value up to excluding the last value provided. The start value is often denoted as this
to indicate the current value being acted upon.
If you run the previous example multiple times, you will notice a random number will always be generated, but a value of 10
will never be generated.
Output:
... Example Two ...
5
Use the shuffled()
Extension Function of Iterable
in Kotlin
The shuffled()
function is an extension function of Iterable
interface and returns a List
of elements. The list returned allows us to use the random()
extension function of the Collection
interface to generate a random number.
Comment on the previous example and copy and paste the following code into the Main.kt
file after it.
fun exampleThree(): Int{
return (0..10).shuffled().random()
}
fun main(){
println("... Example Three ...")
println(exampleThree());
}
In this code, we have created a method named exampleThree()
that returns an integer generated in the method.
The range of numbers from 0
to 10
are returned as a shuffled list by the shuffled()
function. Note that the range is inclusive.
If you want the range to be exclusive, use the Int.until()
function, as discussed in the previous example.
The random()
function generates a random number from the shuffled list, and if the list is empty, the function throws NoSuchElementException
.
If you run this code multiple times, a random number will be generated from the shuffled list for each execution.
Output:
... Example Three ...
7
Use ThreadLocalRandom
in Kotlin
The current thread uses the ThreadLocalRandom
to generate a random number. This generator is mostly used in concurrent programs to reduce overhead and contention.
The generator is specifically used when different programs access random numbers in parallel on thread pools.
When all our usages are of the form ThreadLocalRandom.current.nextX()
where the X
can be either nextInt()
, nextLong()
, etc. It ensures that a generator cannot be shared across multiple threads.
The current()
method is a static method that returns an object of ThreadLocalRandom
and can only be invoked by the current thread.
Comment on the previous code and copy and paste the following code in the Main.kt
file after it.
import java.util.concurrent.ThreadLocalRandom
fun exampleFour(bound: Int): Int{
return ThreadLocalRandom.current().nextInt(bound)
}
fun main(){
println("... Example Four ...")
println(exampleFour(9))
}
The nextInt()
method generates a pseudorandom number that is uniformly distributed between 0
up to but excluding the number we provided as bound
.
If the bound
is not positive, the method throws IllegalArgumentException
. If you run the above code multiple times, you will get a different pseudorandom number that is uniformly generated.
Output:
... Example Four ...
3
Conclusion
This tutorial has taught the different ways that we can generate a random number in Kotlin, and the approaches covered include:
- Using the
random()
extension function ofIntRange
. - Using the
shuffled()
extension function ofIterable
. - Using
ThreadLocalRandom
.
We also learned to modify the range generated to be inclusive or exclusive by using ..
or until
in the range.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub