How to Implement the Floor Modulo Function in Kotlin
- Create a New Kotlin Project
-
Create a Custom Floor Modulo Function for
Int
in Kotlin -
Create a Custom Floor Modulo Function for
Double
-
Create a Custom Floor Modulo Function for
Float
- Conclusion
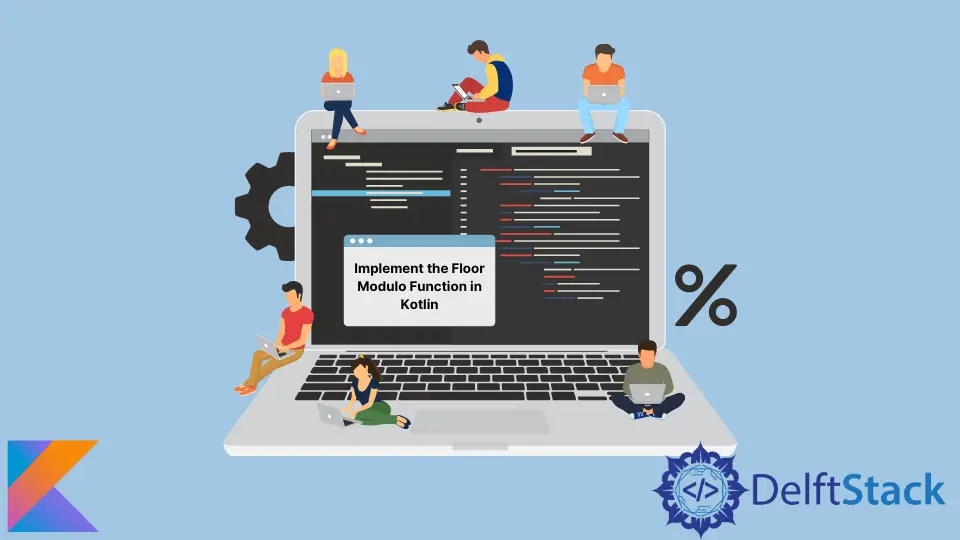
Every programming language has operators that help do different computations, including arithmetic operators, assignment operators, comparison operators, and logical operators.
These operators help us perform common arithmetic operations, but they do not always meet our requirements. In such situations, the developer goes out of his way to create an algorithm that uses these operators to meet their requirements.
These algorithms are usually abstracted using a method; we only need to invoke this method with the right parameters to get our result.
For example, mod()
is an extension function implemented by all the Number
types in Kotlin to calculate the remainder of the flooring division of this number with another number, but it only accepts an argument of type Double
or Float
.
In this tutorial, we will learn how to create a custom function to calculate the remainder of the flooring division of this number with another number using any argument that meets the developer’s requirements.
Create a New Kotlin Project
Open IntelliJ IDEA and select File
> New
> Project
. On the window that opens, enter a project named floor-modulo
, select the Kotlin
on the Language
section, and select Intellij
on the Build system
section.
Finally, press the Create
button to generate the Kotlin project.
Create a Custom Floor Modulo Function for Int
in Kotlin
Once the project has been generated, create a file named Main.kt
under the src/main/kotlin
folder and copy and paste the following code into the file.
infix fun Int.floorMod(other: Double) =
((this % other) + other) % other
fun main(){
val intValue = 5
println(intValue.floorMod(3.2))
}
In this code, we have created an extension function named floorMod()
, a function of the Int
data type. This method calculates the remainder of the flooring division of an Int
value with another value of type Double
.
This is an example to show how to create a custom implementation, which means the developer can use any of the Number
type implementations as the argument of this function.
Note that the function must first be defined before application use. Run this code and ensure the output is as shown below.
1.7999999999999998
Create a Custom Floor Modulo Function for Double
infix fun Double.floorMod(other: Int) =
((this % other) + other) % other
fun main(){
val intValue = 5
println(3.2.floorMod(intValue));
}
In this code, we have created a method named floorMod()
, a function of the Int
data type. This method calculates the remainder of the flooring division of a Double
value with another value of type Int
.
This example works the same way as the previous example, and if we need to pass a different argument to this method, we have to define a function that accepts the argument we want. Run this code and ensure the output is as shown below.
3.1999999999999993
Create a Custom Floor Modulo Function for Float
infix fun Float.floorMod(other: Double) =
((this % other) + other) % other
fun main(){
val floatValue = 5F
println(floatValue.floorMod(3.2))
}
By now, we have known how to create a custom function to calculate floor modulo to meet the required specification. This code creates an extension function named floorMod()
and is a function of the Float
data type.
This method calculates the remainder of the flooring division of a Float
value with another value of type Double
.
As mentioned in the previous examples, the process of creating an extension function for Float
to accept a different argument. Run this code and ensure the output is as shown below.
1.7999999999999998
Conclusion
In this tutorial, we have learned how to create a custom extension function to help us calculate the floor modulo of two numbers. The topics we have covered include calculating floor modulo for Int
, Double
, and Float
data types.
Note that we should use the same approach for the remaining Number
implementations such as Long
, Short
, and Byte
.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub