How to Create an Empty Mutable List in Kotlin
-
Use the
mutableListOf()
Method in Kotlin -
Use the
arrayListOf()
Method in Kotlin - Use the Constructor of a Data Structure in Kotlin
- Use the Implicit Declaration in Kotlin
- Conclusion
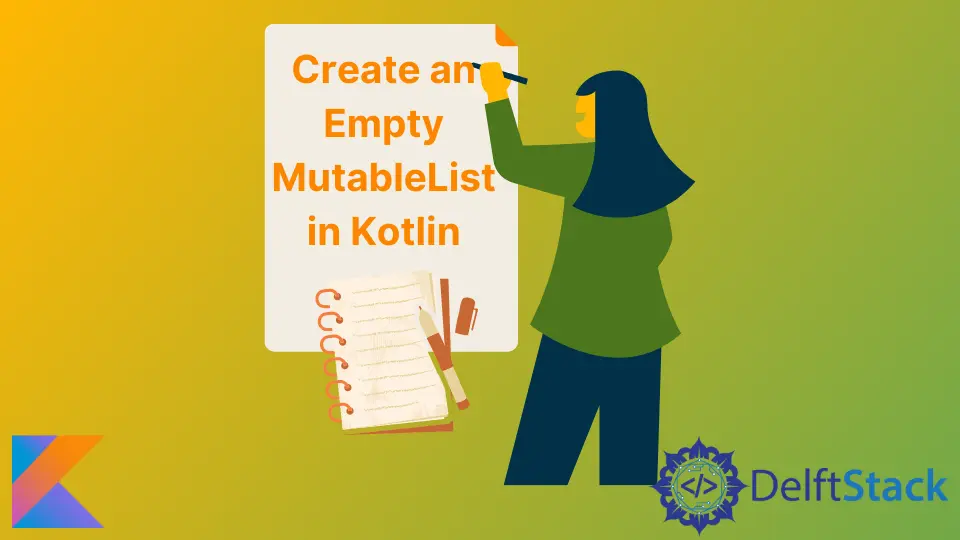
Before diving into the examples, we must understand two common terms when working with the type List
data.
The terms include mutable and immutable. A mutable list is a list that can be modified by adding or removing elements in the data structure.
An immutable list is a list that cannot be modified by either adding or removing elements in the data structure.
From the two terms, we can have two types of lists that include List
and MutableList
. Note that MutableList
is a list because it inherits from the List
interface.
The MutableList
type returns a list that can be modified, and the List
type returns a list that cannot be modified. However, when you create an implementation of the List
without specifying whether the list is mutable or immutable, it is assumed to be mutable by default.
This tutorial will teach us how to create an empty mutable list. An empty list means that there are no elements in the data structure.
Use the mutableListOf()
Method in Kotlin
Go to IntelliJ
and create a new Kotlin project. Create a new Kotlin file named Main.kt
under the kotlin
folder.
Copy the following code and paste it into the file.
val mutableEmptyList: MutableList<String> = mutableListOf();
fun main() {
println(mutableEmptyList.isEmpty());
mutableEmptyList.add("I am the first element !")
println(mutableEmptyList.isEmpty());
}
The mutableListOf()
method returns an empty list of type MutableList
, meaning that we can add and remove elements in the data structure. To verify this, you can invoke the add()
or remove()
method on the returned object.
Run the above code and note that the isEmpty()
method returns true
before any element is added and false
after an element is added, as shown below.
true
false
Use the arrayListOf()
Method in Kotlin
Comment on the previous example and paste the following code into the Main.kt
file.
val mutableEmptyList: MutableList<String> = arrayListOf();
fun main() {
println(mutableEmptyList.isEmpty());
mutableEmptyList.add("I am the first element !")
println(mutableEmptyList.isEmpty());
}
The arrayListOf()
method returns an empty ArrayList
, but in the previous example, we are returning a MutableList
. How is this possible?
In the introduction section, we mentioned that the MutableList
is a List
because it inherits from the List
interface; since ArrayList
implements the List
interface, we can return MutableList
using the ArrayList
.
Once we have access to the mutable list, we can invoke the isEmpty()
method to verify whether the method is empty. Run the above code and observe that the outputs are as shown below.
true
false
Use the Constructor of a Data Structure in Kotlin
Comment on the previous example and paste the following code into the Main.kt
file.
import java.util.LinkedList
val mutableEmptyList: MutableList<String> = LinkedList();
fun main() {
println(mutableEmptyList.isEmpty());
mutableEmptyList.add("I am the first element !")
println(mutableEmptyList.isEmpty());
}
The LinkedList
class implements the List
interface, which helps us return a MutableList
of type LinkedList
.
The LinkedList()
constructor returns an empty list and applies to the constructors of other data structures that implement the List
interface.
Run the above example and note that the output prints the same as the other examples. The output is shown below.
true
false
Use the Implicit Declaration in Kotlin
Comment on the previous example and paste the following code into the Main.kt
file.
val mutableEmptyList = ArrayList<String>();
fun main() {
println(mutableEmptyList.isEmpty());
mutableEmptyList.add("I am the first element !")
println(mutableEmptyList.isEmpty());
}
As you have noted in the previous examples, we were directly specifying the return type we wanted, which was the MutableList
. In the introduction section, we mentioned that any list implementation is assumed to be mutable if we do not specify whether we want a read-only list.
This example shows how the MutableList
is returned implicitly by defining a List
implementation without indicating the return type.
Run the above code and note that the isEmpty()
method inherited from the AbstractCollection
class returns true
before any element is added and false
after an element is added. The output is shown below.
true
false
Conclusion
In this tutorial, we have learned how to create an empty MutableList
using different approaches that included: using the mutableListOf()
method, using the arrayListOf()
method, using the constructor of a data structure, and using the implicit declaration approach.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub