How to Create Constants in Kotlin
- Use Class Constants in Kotlin
- Use a Singleton in Kotlin
- Use Top-Level Constants in Kotlin
- Use a Separate File Containing Constants in Kotlin
- Conclusion
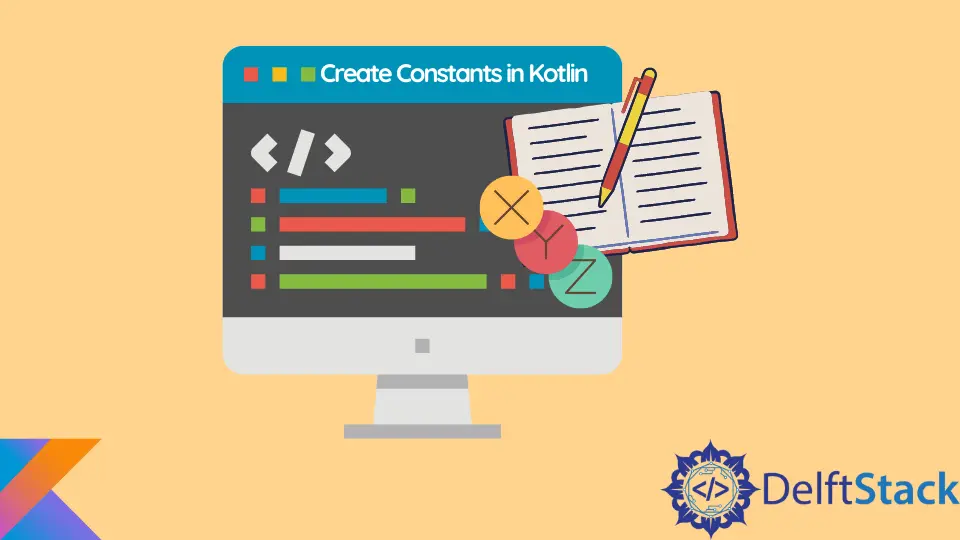
A constant is used to store data whose values are always known, and the data does not change during the program execution. Constants differentiate from variables in that variables can change the data during the program execution.
For example, let’s say you are developing a motorbike simulator. Different properties of the motorbike will never change during its entire life, such as the number of wheels.
To ensure the number of wheels is not changed, we declare a constant in the application and assign it to the number of wheels a motorbike can have. We use constants to avoid errors from user input, such as providing four wheels for a motorbike instead of two.
In this tutorial, we will learn how to create constants in Kotlin, the naming convention used, and how to include them in your application.
Use Class Constants in Kotlin
Go to IntelliJ and select File > New > Project
to create a new Kotlin project. Enter kotlinConstants
as the project name or any name preferred.
Select Kotlin on the Language section and Intellij on the Build System section. Press the Create button to create the project.
Create the folder structure com/constants
under the kotlin folder. Create a Main.kt
file under the constants
folder, and copy and paste the following code into the file.
package com.constants
class Car(private val name: String){
/**
* This is a constant that is only used
* inside this class
*/
private val numberOfWheels = 4
/**
* The companion object defines a constant
* that exists only as a single copy and
* Can also be used outside the class
*/
companion object{
const val NUMBER_OF_WHEELS = 4
}
fun showMessage(): String{
return "A ${this.name} has ${this.numberOfWheels} wheels"
}
}
fun main() {
val nissan = Car("Nissan");
println(nissan.showMessage());
println("Number of wheels = ${Car.NUMBER_OF_WHEELS}");
}
We have declared two constants inside the Car class; the first constant declared using the private
keyword is only meant to be used in this class and cannot be accessed from outside the class.
As for the naming convention, we have used the camel case naming convention since it is only used inside the class.
The second constant is declared inside a companion object in the class. A companion object is used to declare constants and functions that belong to this class, meaning that it cannot be instantiated using the class objects.
These constants are similar to static fields and methods in Java. To invoke the constants, we usually use the class because only one copy of the constants exists.
The constants declared inside a companion object can be accessed from outside the class, and the naming convention used for these constants is a combination of uppercase letters and underscores.
The main method invokes Car()
to create a new Car
object. The showMessage()
method uses the constant used within the class to log a message containing the value to the console.
The last println()
message logs the value of the constant declared using a companion object. Run the code to verify that the program outputs the following.
Output:
A Nissan has 4 wheels
Number of wheels = 4
Use a Singleton in Kotlin
Singleton is a design pattern in programming, especially in high-level languages. The singleton design pattern is used when we have resources that need to be shared in the application and in situations when it is expensive to create the resources.
Note that when you use a singleton, only one instance will be created during the whole lifetime of the application.
Comment the previous code and copy and paste the following code into the Main.kt
file after the comment.
package com.constants
object Configuration{
const val USERNAME = "john"
const val PASSWORD = "1234"
}
fun userLogin(user: String, pass: String): String{
return "$user logged in with password $pass"
}
fun main() {
println(userLogin(Configuration.USERNAME,
Configuration.PASSWORD));
}
In this example, we have created a singleton using the object
keyword. Always use this syntax when you want to create a singleton in Kotlin.
Behind the scenes, the compiler makes the constructor private, creates a reference of our Configuration
, and initializes it in a static block. The singleton will only be initialized once we first access any static fields.
The userLogin()
is a custom method we have defined to consume the singleton properties. To access the values, we invoke the singleton name followed by the property name.
This is similar to what we did with constants declared in a companion object, but this approach is less resource-intensive. Run the code and verify that the program outputs the following.
Output:
john logged in with password 1234
Use Top-Level Constants in Kotlin
Comment the previous code, and copy and paste the following code into the Main.kt
file after the comment.
package com.constants
const val CONNECTION_POOL = 10
class DBDriver{
companion object{
fun isConnectionExceeded(value: Int): Boolean{
return (value > CONNECTION_POOL)
}
}
}
fun main() {
println(DBDriver.isConnectionExceeded(11));
}
Top-level constants are not enclosed in a function, a class, or an interface, which means you can call them from outside the class without creating any object.
In this example, we have declared a top-level constant named CONNECTION_POOL
and consumed it inside the isConnectionExceeded()
method by passing its name.
Run the code and note that the constant value is read as usual to perform the expected computations, as shown below.
Output:
true
Use a Separate File Containing Constants in Kotlin
Create a new package named consts
under the com
folder. Create a file named NetConstants.kt
under the consts
folder, and copy and paste the following code into the file.
package com.consts
const val PORT = 8080
const val PROTOCOL = "DNS"
const val HOST_ADDRESS = "192.168.1.0"
The constants defined in the above file are similar to top-level constants but are declared in a separate file with other application parts.
The following example shows the use of constants in a separate file of the same application. Comment on the last example we covered in the Main.kt
file, and copy and paste the following code into the file after the comment.
package com.constants
import com.consts.HOST_ADDRESS
import com.consts.PORT
import com.consts.PROTOCOL
class Server{
companion object{
fun connectServer(port: Int, protocol: String, host: String): String{
return "application connected to " +
"$host using port " +
"$port through" +
" $protocol"
}
}
}
fun main() {
println(Server.connectServer(PORT, PROTOCOL, HOST_ADDRESS));
}
This example defines the connectServer()
method declared inside a companion object of a class. The method consumes the three constants we defined in the NetConstants.kt
file.
This is achieved by passing the constant’s name to the method and importing the fully qualified constant’s name into the class. The following is the output of the application after we run it:
application connected to 192.168.1.0 using port 8080 through DNS
Conclusion
In this tutorial, we have learned the different ways we can use to create constants in a Kotlin application. The approaches that we have learned include: using class constants, singleton, and top-level constants, and finally, we covered how to use constants declared in a separate file.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub