How to Check if an Element Is Hidden in jQuery
- Using jQuery’s is(’:hidden’) Method
- Using jQuery’s is(’:visible’) Method
- Using jQuery’s CSS Method
- Conclusion
- FAQ
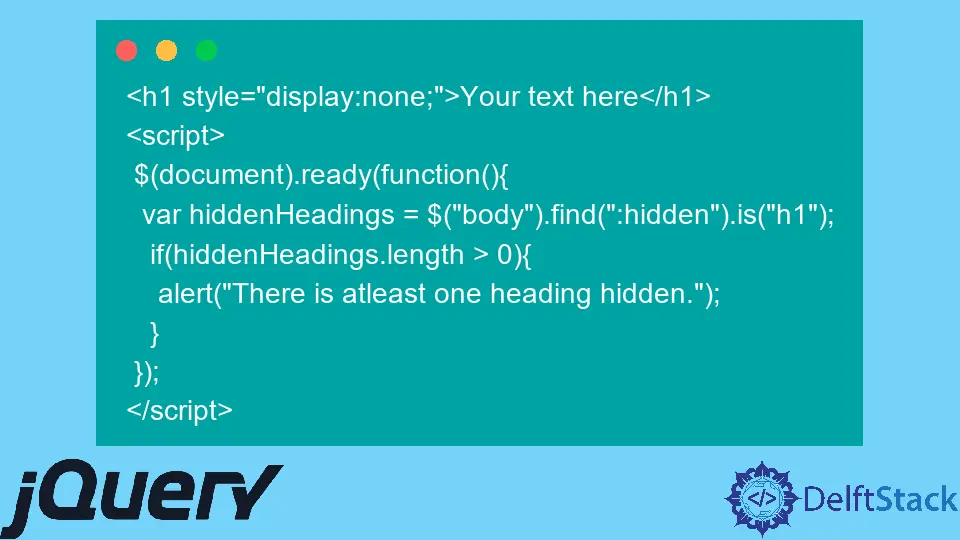
When working with web development, particularly with jQuery, you may often need to determine whether a specific element on your page is hidden or visible. Understanding how to check if an element is hidden is crucial for creating dynamic and interactive user experiences.
In this tutorial, we will explore various methods in jQuery to check the visibility of elements. Whether you are toggling menus, displaying alerts, or managing content dynamically, knowing how to evaluate the visibility of elements is a key skill. Let’s dive into the ways you can achieve this with jQuery.
Using jQuery’s is(’:hidden’) Method
One of the simplest ways to check if an element is hidden in jQuery is by using the is(':hidden')
method. This method returns true
if the element is hidden and false
if it is visible. This is particularly useful when you want to execute certain actions based on the visibility of an element.
Here’s how you can implement this method:
$(document).ready(function() {
if ($('#myElement').is(':hidden')) {
console.log('The element is hidden.');
} else {
console.log('The element is visible.');
}
});
Output:
The element is hidden.
In this example, we first check if the element with the ID myElement
is hidden. The is(':hidden')
method efficiently checks the visibility state. If the element is hidden, it logs a message indicating that; otherwise, it confirms that the element is visible. This method is straightforward and effective for quick checks.
Using jQuery’s is(’:visible’) Method
Conversely, you can also use the is(':visible')
method to check if an element is visible. This method works similarly to is(':hidden')
but returns true
if the element is visible and false
if it is hidden. This can be particularly useful for scenarios where you want to perform an action only when an element is visible.
Here’s an example of how to use this method:
$(document).ready(function() {
if ($('#myElement').is(':visible')) {
console.log('The element is visible.');
} else {
console.log('The element is hidden.');
}
});
Output:
The element is visible.
In this code snippet, we check the visibility of the element with the ID myElement
using the is(':visible')
method. Depending on the result, it logs whether the element is visible or hidden. This approach is equally efficient and can be used interchangeably with the is(':hidden')
method, depending on your needs.
Using jQuery’s CSS Method
Another method to check if an element is hidden is by using the css()
method to examine the display property of the element. If the display property is set to none
, the element is considered hidden. This method provides a more granular approach as you can check for specific CSS properties.
Here’s how you can implement this:
$(document).ready(function() {
if ($('#myElement').css('display') === 'none') {
console.log('The element is hidden.');
} else {
console.log('The element is visible.');
}
});
Output:
The element is hidden.
In this example, we use the css()
method to retrieve the display property of the element. If it returns none
, we conclude that the element is hidden. This method allows for more flexibility, as you can check for other CSS properties if needed. However, it is slightly more verbose than using the is()
methods.
Conclusion
Checking if an element is hidden in jQuery is a fundamental skill for web developers. Whether you choose to use the is(':hidden')
, is(':visible')
, or css()
methods, each approach provides a reliable way to determine the visibility of elements on your web page. By mastering these techniques, you can enhance user interaction and create more dynamic web applications. Remember to choose the method that best fits your specific needs and coding style.
FAQ
- How can I check if multiple elements are hidden in jQuery?
You can use theeach()
method to iterate through a collection of elements and check their visibility usingis(':hidden')
.
-
What should I do if the element is hidden due to CSS styles?
You can still use thecss()
method to check the display property, or you can inspect the element using browser developer tools to understand the applied styles. -
Can I check the visibility of elements that are hidden due to JavaScript?
Yes, jQuery methods likeis(':hidden')
will still work regardless of how the element was hidden, whether through CSS or JavaScript. -
Is there a performance difference between these methods?
Generally, theis(':hidden')
andis(':visible')
methods are faster and more efficient than using thecss()
method, especially when checking multiple elements. -
Can I use these methods in a responsive design?
Absolutely! These methods work well in responsive designs, allowing you to check visibility based on different screen sizes and layouts.