How to Add Table Row in jQuery
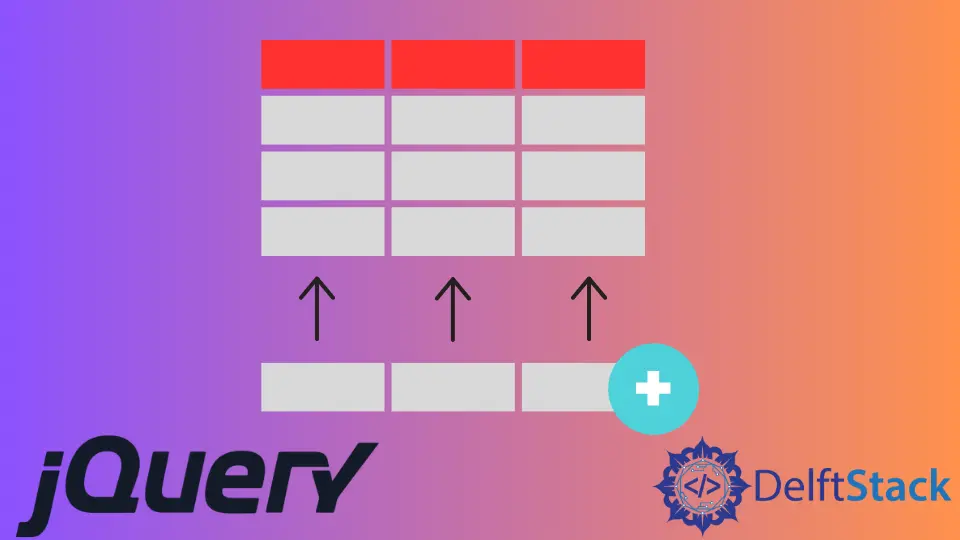
In present-day HTML table element has various inner elements like tbody
, thead
, tfoot
to contain rows of data.
<table id="test">
<tbody>
<tr><td>Foo</td></tr>
</tbody>
<tfoot>
<tr><td>footer information</td></tr>
</tfoot>
</table>
It leads to the need to be precise while inserting the table row in the DOM. jQuery comes with two methods of inside and outside DOM insertion to add an element.
append()
/ prepend()
to Add Table Row in jQuery
To add a row in the table body using jQuery, we can use DOM inside insertion methods of append()
or prepend()
that adds an element to the suggested element’s start or end. Here we will select the tbody
element of table
element with id="test"
to add a row after it.
<script>
$("#test>tbody").append("<tr><td>Test Row Append</td></tr>");
//adding row to end and start
$("#test>tbody").prepend("<tr><td>Test Row Prepend</td></tr>");</script>
After running the following script, the new HTML will look like the following.
<table id="test">
<tbody>
<tr><td>Test Row Prepend</td></tr>
<tr><td>Foo</td></tr>
<tr><td>Test Row Append</td></tr>
</tbody>
<tfoot>
<tr><td>footer information</td></tr>
</tfoot>
</table>
This method of adding a row in jQuery can be achieved by using appendto()
and prependto()
method.
<script>
$("<tr><td>Test Row Append</td></tr>").appendto("#test>tbody");
$("<tr><td>Test Row Prepend</td></tr>").prependto("#test>tbody");
</script>
Use Outside DOM Insertion to Insert Table Row in jQuery
jQuery comes with .after()
and .before()
methods to insert an element after and before the specified element respectively. We can use these methods to be precise by adding a table row in various table positions.
<table>
<tbody>
<tr><td> Item First </td></tr>
<tr><td> Item Last </td></tr>
</tbody>
</table>
<script>
$("table tbody:nth-child(1)").after("<tr><td> Item Second </td></tr>");
//adding second item after 1st item
$("table tbody:last-child").before("<tr><td> Item Just Before Last</td></tr>");
//adding an item before last item
<script>
Add Table Row Using JavaScript
Alternatively, it also has a native JavaScript way of adding a row to the table using the insertRow()
function.
<table id="test">
<tr>
<td>row1 column1</td>
<td>row1 column2</td>
</tr>
</table>
<script>
function addrow(){
var table = document.getElementById("test");
var row = table.insertRow(0);
//this adds row in 0 index i.e. first place
row.innerHTML = "<td>row0 column1</td><td>row0 column2</td>";
}
</script>