How to Fix TypeError: Converting Circular Structure to JSON
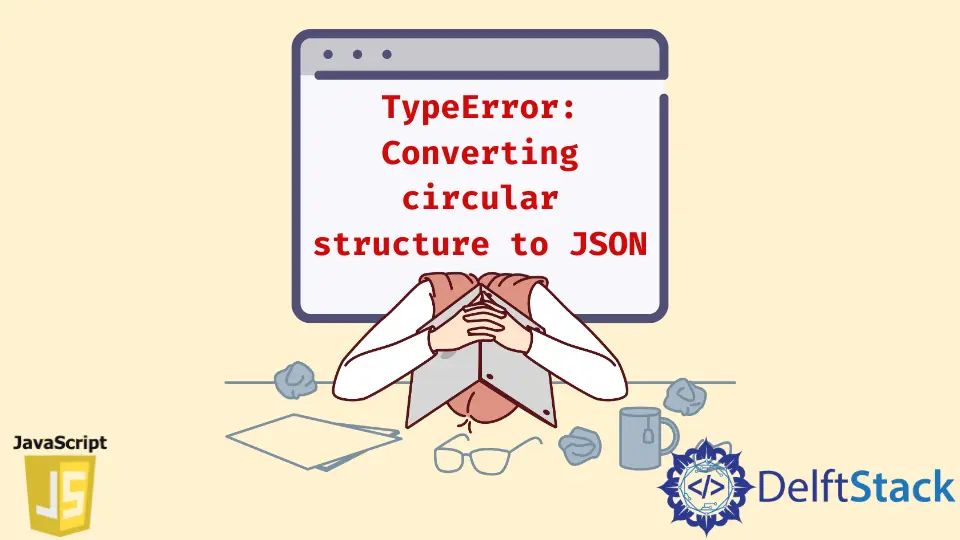
When we send an object containing circular references to the JSON.stringify()
function, we get the TypeError: Converting circular structure to JSON
problem. Before converting the object to JSON, avoid any circular reference.
Fix TypeError: Converting circular structure to JSON
in JavaScript
This issue can be solved using the flatted
package. The flatted
package is a Circular JSON parser that is incredibly light and quick, directly from the inventor of CircularJSON
.
First, you have to install a flatted
package, and you can do it the following way.
npm i flatted
Let’s see one example of using a flatted
package.
const {parse, stringify} = require('flatted/cjs');
var sports = [{cricket: 1}, {football: '2'}];
sports[0].a = sports;
stringify(sports);
console.log(sports);
Output:
JSON.stringify()
not only turns valid objects into strings but also has a replacer parameter that may replace values if the function is configured.
let sportsmanObj = {
name: 'Shiv',
age: 22,
gender: 'Male',
};
sportsmanObj.myself = sportsmanObj;
const circularFunc = () => {
const sited = new WeakSet();
return (key, value) => {
if (typeof value === 'object' && value !== null) {
if (sited.has(value)) {
return;
}
sited.add(value);
}
return value;
};
};
JSON.stringify(sportsmanObj, circularFunc());
console.log(sportsmanObj);
We are calling on the WeakSet
object in our circularFunc
above, which is an object that stores weakly held items or pointers to things.
Each object in a WeakSet
can only appear once, removing repetitive or circular data. The new
keyword is an operator for creating a new object.
We have nested if
statements in our return
statement. The typeof
operator is used in our first if
statement to return the primitive (Undefined
, Null
, Boolean
, Number
, String
, Function
, BigInt
, Symbol
) being evaluated.
If our type of value is precisely equivalent to an object and the importance of that object is not null, it will proceed to the second if
condition, which will check to see if the value is in the WeakSet()
. We send our original circular structure and replacer method to JSON.stringify()
.
This will give us the stringified result we want on the console.
{
age: 22,
gender: "Male",
myself: [circular object Object],
name: "Shiv"
}
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn