How to Create A Simple Calculator in JavaScript
Muhammad Muzammil Hussain
Feb 02, 2024
- The JavaScript Calculator
-
Use the
if-else if
Statement to Build a Calculator in JavaScript -
Use the
switch
Statement to Build a Calculator in JavaScript
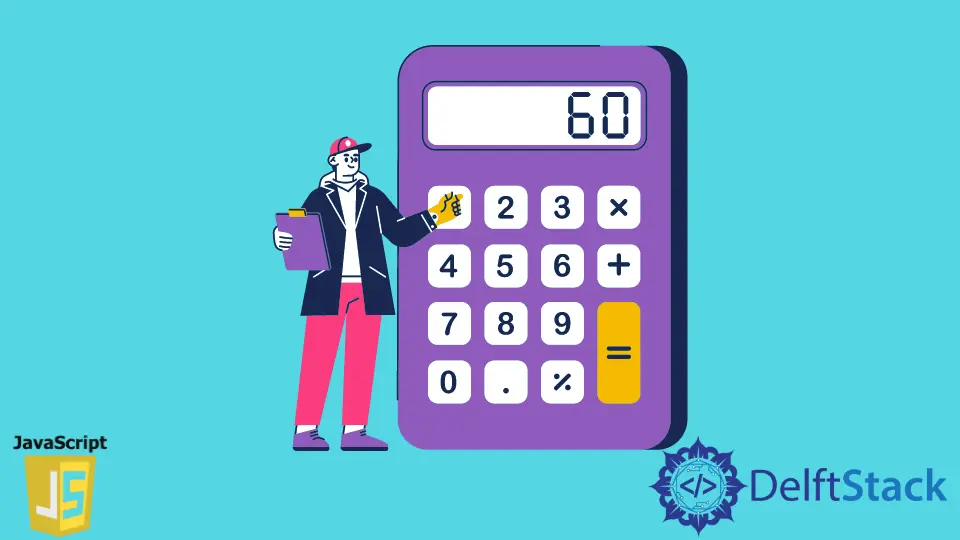
This article will let you know how to develop a simple calculator using multiple ways on JavaScript. To understand this, you must know about the following JavaScript conditions which are:
- The JavaScript
If else if
statement - The JavaScript
switch
statement
The JavaScript Calculator
We use a calculator to perform numerous mathematical operations like addition, subtraction, multiplication in our daily life routine.
We can also have scientific calculators used to solve complex problems, like square root and log functions.
JavaScript is the most common and preferred language to build calculators for adding web pages.
Use the if-else if
Statement to Build a Calculator in JavaScript
<html>
<head>
<title>
JavaScript calculator using If Else If
</title>
</head>
<script>
// getting number-1 from user
var no1 = parseFloat(prompt("Please Enter your 1st No. : "));
// getting operator to perform operation on two numbers
var operator = prompt("Enter operator ( either +, -, * or / ) : ");
// getting number-2 from user
var no2 = parseFloat(prompt("Please Enter your 2nd No. : "));
var result;
// using if else if to perform operation according to the operator
if (operator == "+")
{
result = no1 + no2; // to add two numbers
}
else if (operator == "-")
{
result = no1 - no2; // to subtract two numbers
}
else if (operator == "*")
{
result = no1 * no2; // to multiple two numbers
}
else
{
result = no1 / no2; // to divide two numbers
}
// Displaying the result
window.alert("Required result is : " + result);
</script>
<body>
<h2 style="text-align: center">Thats practical demo about JavaScript
calculator using JavaScript If Else If</h2>
<body>
</html>
Output:
Please Enter your 1st No. : 12
Enter operator ( either +, -, * or / ) : +
Please Enter your 2nd No. : 13
Required result is : 25
That's a practical demo about JavaScript calculators using JavaScript. If Else If
Use the switch
Statement to Build a Calculator in JavaScript
<html>
<head>
<title>
JavaScript calculator using switch statement
</title>
</head>
<script>
// getting number-1 from user
var no1 = parseFloat(prompt("Please Enter your 1st No. : "));
// getting operator to perform operation on two numbers
var operator = prompt("Enter operator ( either +, -, * or / ) : ");
// getting number-2 from user
var no2 = parseFloat(prompt("Please Enter your 2nd No. : "));
var result;
// using switch statement to operate according to the operator
switch(operator)
{
case "+":
result = no1 + no2; // adding two numbers
break;
case "-":
result = no1 - no2; // subtracting two numbers
break;
case "*":
result = no1 * no2; // multiplying two numbers
break;
case "/":
result = no1 / no2; // dividing two numbers
break;
default:
console.log('Invalid operator');
break;
}
// Displaying the result
window.alert("Required result is : " + result);
</script>
<body>
<h2 style="text-align: center">Thats practical demo about JavaScript
calculator using JavaScript switch statement</h2>
<body>
</html>
Output:
Please Enter your 1st No. : 25
Enter operator ( either +, -, * or / ) : /
Please Enter your 2nd No. : 5
Required result is : 5
That's a practical demo about JavaScript calculator using JavaScript switch statement