How to Send POST Request Using XMLHttpRequest in JavaScript
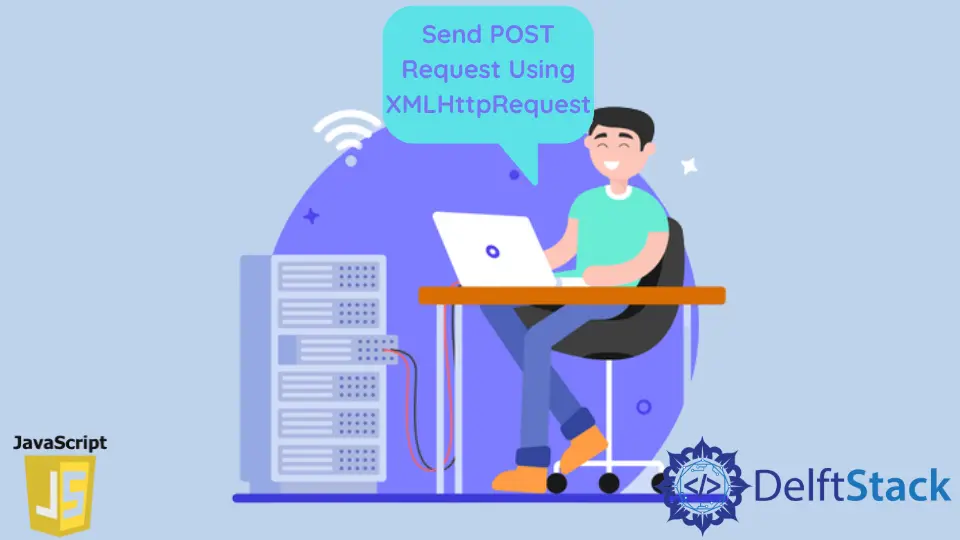
This article will explain how to send an XMLHttpRequest post request in AJAX programming using JavaScript code with different examples.
The XMLHttpRequest
To fetch data from a web server, we use XMLHttpRequest
(XHR). It is an API in the form of an object which transfers data between a web browser and a web server.
The XMLHttpRequest
is primarily used in AJAX programming.
AJAX Programming
AJAX stands for Asynchronous JavaScript and XML. It is not a programming language, but AJAX is a set of web development techniques that use several web technologies to develop asynchronous web applications on the client-side.
We can send data to a web server in the background using AJAX.
Once the page loads, we can read data from a web server and use AJAX without reloading. We can update the webpage as well.
Basic syntax for creating an XMLHttpRequest
object:
my_variable = new XMLHttpRequest();
We define the call-back function during the loading of a request.
my_variable.onload = function() {
// Here we can use the Data
}
Now, we can send a request.
xhttp.open("REQUEST_METHOD, "FILE_PATH")
xhttp.send();
Send POST
Request Using XMLHttpRequest in JavaScript
POST request helps us send data from the client-side to the server. We use the POST
method if we need to update a file or data in our database.
The POST
method has no limitations on size, which means we can send a huge amount of data to the server. We commonly use the POST
method to receive user inputs and store them in our DB like the signup form.
The POST
method is more secure than the GET
method.
Basic Syntax:
my_variable = new XMLHttpRequest();
my_variable.onload = function() {
// Here, we can use the data
} xhttp.open('POST', 'MY_FILE_PATH');
xhttp.send();
By using the POST
method, we will create a complete JavaScript source as an example to better understand the syntax and working of the POST
request. Remember that we need to set headers in our object before executing a request.
Example code:
<!DOCTYPE html>
<html>
<body>
<h2>XMLHttpRequest using POST method</h2>
<button type="button" onclick="loadRequest()">Request post method</button> // calling of a request
<p id="test"></p>
<script>
function loadRequest() {
const requestObject = new XMLHttpRequest(); // object of request
requestObject.onload = function() {
document.getElementById("test").innerHTML = this.responseText; // displaying response text in paragraph tag
}
requestObject.open("POST", "MY_FILE_PATH");
requestObject.setRequestHeader("Content-type", "application/x-www-form-urlencoded"); // setting of headers in request
requestObject.send("DATA_TO_SEND"); // data to send in request
}
</script>
</body>
</html>
Example code explanation:
- In the HTML source code above, we created a paragraph element and defined an ID to assign its text value.
- We have created a button,
Request post method
, and theonclick
event of that button is our function calledloadRequest()
. - In the
loadRequest()
function, we have created an object ofXMLHttpRequest()
. - Then, we used the call-back function to get the data and assign a request-response to the paragraph using
document.getElementById("test")
. - Now, we opened a request connection and passed the request method
POST
and the network request file path. - We have set up the request headers to define the content type.
- Finally, we sent the request using the data we wanted to post.
- You can save this HTML source with the correct network request file path and save the file with the
.html
extension. - Open it on any browser to see the result.