JavaScript Time Picker Example in HTML
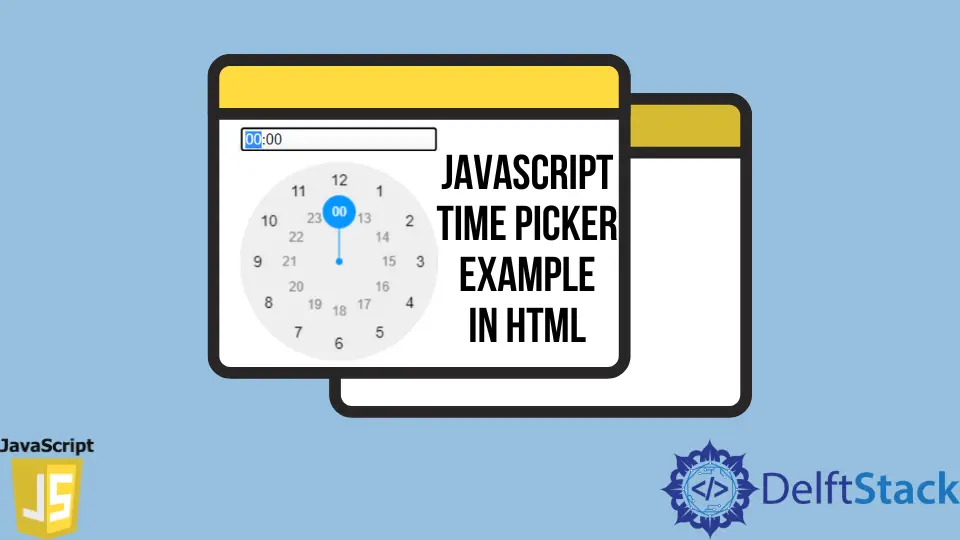
JavaScript Time Picker allows the end-user to select time from directly entering the value or from a pop-up. Time can be present in multiple formats, and it depends on what type of format you require.
JavaScript Time Picker Example in HTML
Here, you can see an example of JavaScript’s clockTimePicker()
method, which helps users select a time value from the pop-up menu. The method takes help from jQuery libraries to show the time options.
Here in the first step, include the following CDNs.
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/md-date-time-picker@2.3.0/dist/css/mdDateTimePicker.min.css">
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/jquery-clock-timepicker@2.5.0/jquery-clock-timepicker.min.js"></script>
The following HTML source code we have included the CDNs and created a form element with tag <input>
by giving id="timePicker"
, and initialized the $('#timePicker').clockTimePicker()
with the same input ID in script
tag.
<!doctype html>
<html lang="en">
<head>
<title>Time Picker</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/md-date-time-picker@2.3.0/dist/css/mdDateTimePicker.min.css">
</head>
<body>
<form>
<input type="text" id="timePicker">
</form>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/jquery-clock-timepicker@2.5.0/jquery-clock-timepicker.min.js"></script>
<script>
$('#timePicker').clockTimePicker();
</script>
</body>
</html>
In the above HTML
page source, you can see a simple input form type of time to get the integer value from the end-user. We can include more properties for further customization by giving options in the object.
$('#timePicker').clockTimePicker({
duration: true,
durationNegative: true,
precision: 5,
i18n: {cancelButton: 'Abbrechen'},
onAdjust: function(newVal, oldVal) {
//...
}
});
Here duration: true
, set the hours settings greater than 23
. Whereas durationNegative
is by default false
, and if its value is true
, the duration will be negative.
Still, it only affects the settings when the duration
condition is true. The precision
condition creates a sequence in minutes like, precision: 5
allows users to select minutes steps with 5-minute difference values (6:05
, 6:10
, 6:15
, 6:20
, …). The precision
condition allows only these values: 1
, 5
, 10
, 15
, 30
, 60
.
The cancelButton: 'Abbrechen'
is only for mobile screens. Using this button, you can cancel the time change.
Here function(newVal, oldVal)
is default value of onAdjust
. The onAdjust
function works when the value is changing.