Difference Between event.stopPropagation and event.preventDefault in JavaScript
- Concept of Propagation
- Phases of Propagation
-
the
stopPropagation()
in JavaScript -
the
preventDefault()
in JavaScript
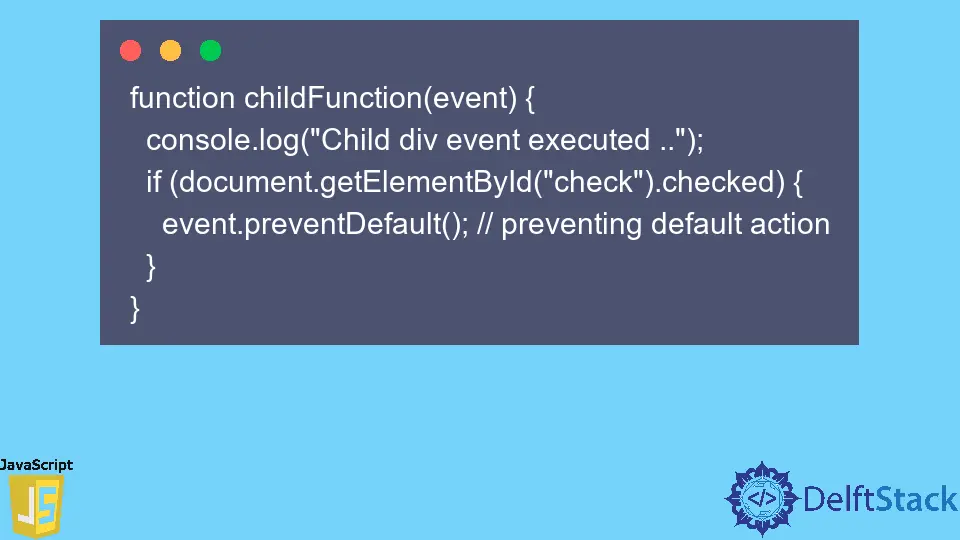
In this article, we will learn the purpose of stopPropagation()
and its work in JavaScript source code. We will also see how we can use a stopPropagation()
event to prevent propagation in JavaScript.
Concept of Propagation
In web development, the DOM is considered a tree containing elements like (parent, child, and siblings) that are related to each other. Propagation is a concept of events traveling through the Document Object Model (DOM) tree.
To better understand propagation, we will use an electric wire as an example, in which current flow is running in a wire until it reaches its defined destination. Events are passed through every node on the DOM until it reaches the last node.
Phases of Propagation
Event Bubbling and Capturing are the phases of propagation. In simple words, Bubbling travels from the targeted node to the root, and Capturing travels inversely to Bubbling; it travels from the root node to the target.
The DOM node, which is used to trigger an event like a button click, is known as a target, and a button with a click event is an event target.
the stopPropagation()
in JavaScript
In the default propagation behavior, when we click a button, it triggers every single event handler along the way, which is associated with the same type. This kind of behavior is not feasible, and it causes performance issues.
To prevent this propagation behavior through the DOM tree, we use the stopPropagation()
event. Using that event, we can execute the only event handler from which it was triggered.
Basic Syntax:
event.stopPropagation()
The stopPropagation()
method is used to prevent the propagation behavior of the same event from being called.
Example Code:
<!DOCTYPE html>
<html>
<body>
<style>
.myDiv {
border: 2px outset black;
padding: 30px;
text-align: center;
}
</style>
<h1 style="color:blueviolet">DelftStack Learning</h1>
<h3>JavaScript stop propagation event</h3>
<p>Click on CHILD DIV</p>
<div class="myDiv" onclick="parentFunction()">
PARENT DIV
<div class="myDiv" onclick="childFunction(event)">CHILD DIV</div>
</div>
Prevent Propagation:
<input type="checkbox" id="check">
<script>
function childFunction(event) {
console.log("Child div event executed ..");
if (document.getElementById("check").checked) {
event.stopPropagation(); // preventing propagation
}
}
function parentFunction() {
console.log("Parent div event executed ..");
}
</script>
</body>
</html>
Output With Propagation:
Output Without Propagation:
In the above HTML source, we created the Child div inside the Parent div, and on the onClick
event of that div, we have called separate functions.
In the <script>
tags, we have declared parentfunction()
and childFunction()
in which we have displayed the event executed in logs. In childFunction()
, we passed event
as an argument and used that event to stop propagation.
We have created a checkbox element to get the current state from a user if he wants to prevent propagation or not. In childFunction()
, we use a conditional statement to find out whether the check box is checked or not.
If it is checked, we just called event.stopPropagation();
to prevent propagation. If the user clicks on the CHILD DIV along with checkbox selection, then only childFunction()
will be executed; else, both functions will be executed.
Save the above source with the HTML extension, and open it in a browser. Open debugger mode along with the console tab to see the results.
the preventDefault()
in JavaScript
The event.stopPropagation()
method is not useful to stop any default behaviors of HTML elements, such as link click or checkbox. To achieve this, we can use event.preventDefault()
.
It prevents the default action the browser makes on that event. So if we use the preventDefault()
method, then only the browser’s default action is prevented, but our div’s click handler still triggers.
Example Code:
function childFunction(event) {
console.log('Child div event executed ..');
if (document.getElementById('check').checked) {
event.preventDefault(); // preventing default action
}
}
In the above JavaScript function childFunction()
, we have just replaced the event.stopPropagation()
with event.preventDefault()
to see the behavior difference in logs.