How to Parse Query String in JavaScript
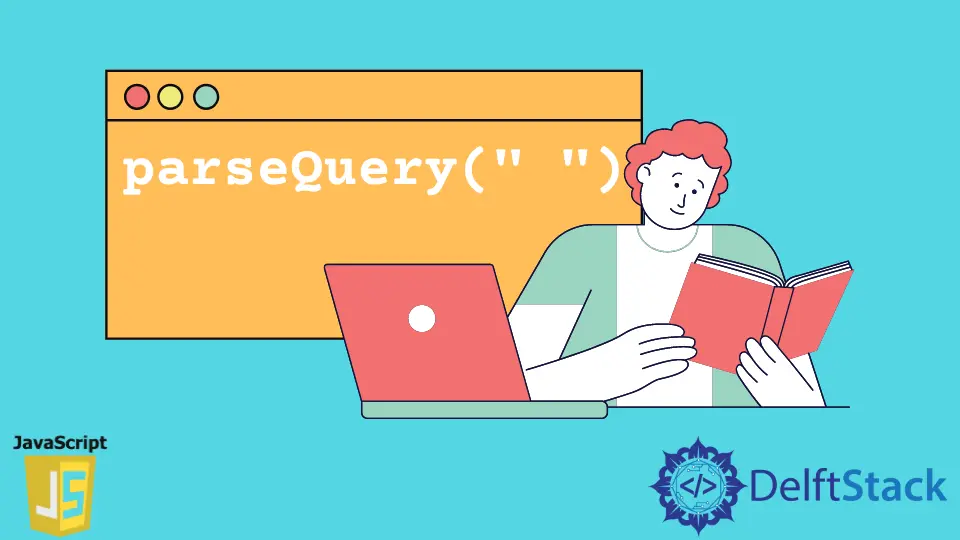
In this tutorial, we look at how parse query strings in JavaScript work, but before getting any further, let’s revisit history and see why we need these query strings in the first place?
A query string
is a part of a hyperlink or URL that comes right after the question mark ?
it sets values to variables known as keys or parameters, somewhat similar to a python dictionary. It sends data from one web page to another along with retrieving specified data from the database or the webserver.
Dummy Hyperlink
www.mysite
.com // default.aspx?username=user+1&units=kgs&units=pounds&permission=false
In the above hyperlink, this part refers as the query:
username = user + 1 & units = kgs& units = pounds& permission = false
That out of the way, parsing
means to scoop out some portion of something, and here we’re talking about query string, which means that this process will take a portion of our query string and work with it. That parsed query string plays an important part in referring or retrieving data requests.
Here’s what a parsed query would look like:
username = user 1
Now let’s see a more tangible example that shows how JavaScript parses the query string of the given hyperlink going through multiple test cases; In this example, our parseQuery
function takes in the hyperlink as an argument and spits out the parsed query in the form of a JavaScript object.
Syntax of parseQuery
parseQuery(query)
- query: The specific string that is passed as the query.
function parseQuery(query) {
object = {};
if (query.indexOf('?') != -1) {
query = query.split('?');
query = query[1];
}
parseQuery = query.split('&');
for (var i = 0; i < parseQuery.length; i++) {
pair = parseQuery[i].split('=');
key = decodeURIComponent(pair[0]);
if (key.length == 0) continue;
value = decodeURIComponent(pair[1].replace('+', ' '));
if (object[key] == undefined)
object[key] = value;
else if (object[key] instanceof Array)
object[key].push(value);
else
object[key] = [object[key], value];
}
return object;
};
Let’s start by creating an empty object, then our query or hyperlink is broken from ?
to get rid of the excess URL.
Once that’s done, we split our query from &
to obtain what’s known as the attribute and its value in the form of a list which gets iterated to extract value and attribute individually.
Now, the key-value pair will check through some of the test cases, and finally, from the remaining value, we replace any +
sign with a space that gives us a more readable value.
Let’s go through our test cases:
Case 1
parseQuery('username=Eren&units=kgs&permission=true');
In this case, we pass a query instead of a URL username=Eren&units=kgs&permission=true
what will happen is that it will skip the first if block, and since there are more than one key and value pair, the query gets split, and this line of code gets checked to be true since we have no duplicate keys.
if (object[key] == undefined) object[key] = value;
Output:
{
username: 'Eren',
units: 'kgs',
permission: 'true'
}
Case 2
parseQuery(
'http:/www.mysite.com//default.txt?username=David+Rogers&units=kgs&permission=false');
Now we use the complete hyperlink
http: /www.mysite.com/ / default.txt ? username = David + Rogers& units =
kgs& permission = false
as argument and the below given if
condition gets True
and this block will extract query from our hyperlink
if (query.indexOf('?') != -1) {
Output:
{
username: 'David Rogers',
units: 'kgs',
permission: 'false'
}
Case 3
parseQuery(
'http:/www.mysite.com//default.txt?username=user1&insect=%D0%B1%D0%B0%D0%B1%D0%BE%D1%87%D0%BA%D0%B0');
Let’s try giving in a URL that contains an encoded message.
http: /www.mysite.com/ / default.txt ? username = user1& insect = % D0 % B1 %
D0 % B0 % D0 % B1 % D0 % BE % D1 % 87 % D0 % BA % D0 % B0
We have used the decodeURIComponent()
function to decode this message.
Output:
{
username: 'user 1',
insect: 'бабочка'
}
Case 4
parseQuery(
'http:/www.mysite.com//default.txt?username=Eren&units=kgs&permission=false&units=pounds');
Finally, we consider a hyperlink containing duplicate keys
http: /www.mysite.com/ / default.txt ?
username = Eren& units = kgs& permission = false& units = pounds
for the first duplicate value below the line of code will execute and create an array.
else object[key] = [object[key], value];
After that, every duplicate entry will get pushed in this array.
else if (object[key] instanceof Array) object[key].push(value);
Output:
{
username: 'Eren',
units: [ 'kgs', 'pounds'],
permission: 'false'
}