Difference Between let and var in JavaScript
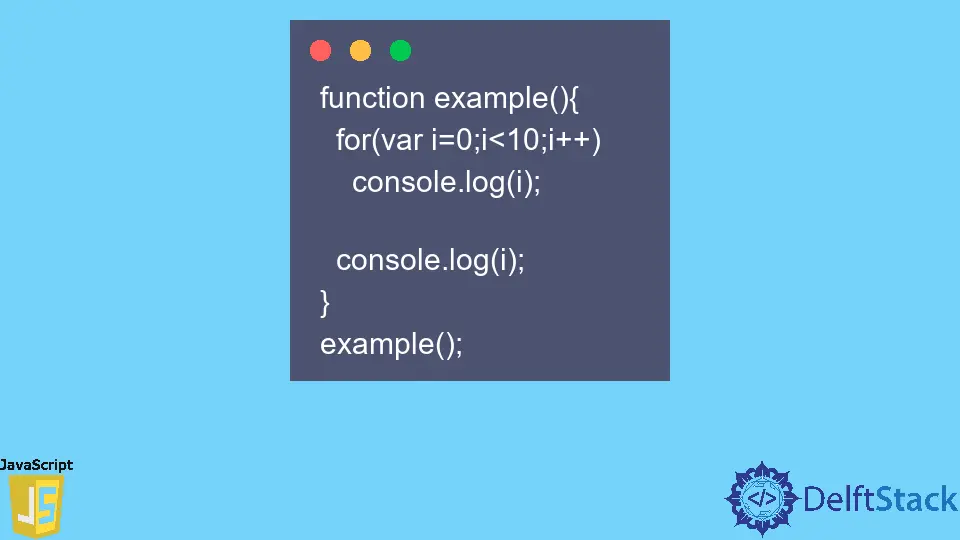
This tutorial article explains the actual working of both var
and let
keywords in JavaScript and also their key differences.
Like other programming languages, JavaScript has variables to store values and data. And in JavaScript, we use both let
and var
keywords to declare variables.
Some people think these two keywords can be used interchangeably, but that is not true. The key differences between the two can cause major errors in our programming.
Before the ES6 update to JavaScript, there was only one way to declare variables and constants in JavaScript. But since the ES6 update, we now have let
and const
keywords used to declare variables and constants.
One of the major reasons for adding the let
and const
keywords to JavaScript was that the variable declared with the var
keyword was not the block in which it was declared. Instead, its scope was limited to the function, causing some programming problems, which we will discuss in the latter part of the article.
Let us look at this code segment for a better understanding.
function example() {
for (let i = 0; i < 10; i++) console.log(i);
console.log(i);
}
example();
In this code segment, we declared variable i
in the for
loop and used a console.log
to get the values of the variable. We have not used the {}
brackets after the for
loop, so the block for the for
loop is only the very next line.
However, we have used an extra console.log
to show the value of i
. But the second console.log
will not be able to get the value of variable i
and will show the following error:
ReferenceError: i is not defined
This error occurred because the scope of the variable i
was only for the for
loop block and could not be accessed outside the block. So, in this way, we cannot use the variable outside the block as the variable declared using the let
keyword has its scope limited to the block only.
To see the difference between let
and var
look at the following code segment:
function example() {
for (var i = 0; i < 10; i++) console.log(i);
console.log(i);
}
example();
In the code above, we can observe that we have used the var
keyword in place of the let
keyword.
While there remains the same two console.log
in the previous example, in this case, the second console.log
will also have an output. So, let us have a look at the output:
0
1
2
3
4
5
6
7
8
9
10
The first console.log
printed the values starting from 0
to 9
, as was the condition i<10
.
But we can see 10
as an output that certainly did not come out of the first console.log
. Therefore, this 10
is the second console.log
output.
The problem here is, the variable i
was supposed to be used within its block, but as it was accessed by the console.log
outside the block, it means it has exceeded its scope.
It proves that let
and var
keywords are used to declare variables, but the var
keyword declares variables limited to the block in terms of their scope. However, the var
keyword has its scope limited to the function.
If we declare a variable outside the function, both let
and var
have another major difference between them. If we use the let
keyword outside the function, a local variable is created that cannot be accessed from outside.
But in case we use the var
keyword, it becomes a global variable. Let us have a look at the following code segment:
var color = 'blue';
let model = '2021';
Here, two variables have been declared in this code segment, one using the let
keyword and the other using the var
keyword. So, the variable declared using the var
keyword has become a global variable
and will get attached to the window
object in the browser.
In browsers, we have a window object
that has many properties and methods and is very complex. The front-end app developers widely know window object
as they work with it a lot.
Every time we use the var
keyword outside of a function, the variable becomes a global variable
and gets itself attached with the window object
and can be accessed from the window object
. In this case, it would be accessed as window.color
will show the value inside the color
variable, which is blue
.
We use third-party libraries and declare variables outside the function using the var
keyword. If the third-party library had a variable with the same name as our variable, that variable would overwrite our variable.
It is another reason for us to avoid adding anything to the window object
; we mean avoiding using the var
keyword in such cases.