How to Create Fibonacci in JavaScript
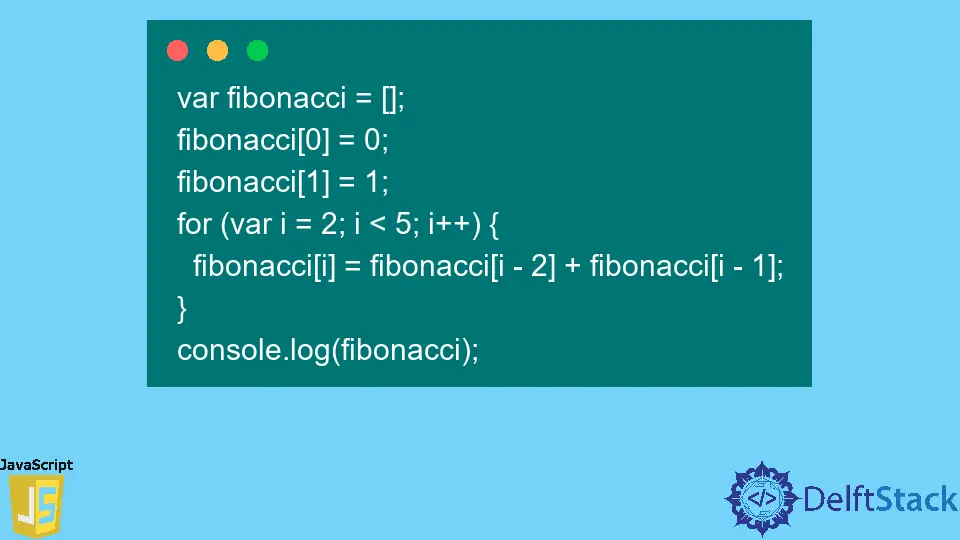
The Fibonacci sequence is a fascinating mathematical concept that has intrigued mathematicians and programmers alike for centuries. Defined as a series of numbers where each number is the sum of the two preceding ones, the sequence begins with 0 and 1. In programming, generating a Fibonacci sequence can be a fun exercise to understand loops and recursive functions.
In this article, we’ll explore how to create a Fibonacci sequence using a loop in JavaScript. Whether you’re a beginner or an experienced developer, you’ll find this guide easy to follow and implement in your own projects. Let’s dive into the world of Fibonacci numbers and see how we can generate them using JavaScript!
Generating Fibonacci Using a Loop
To generate a Fibonacci sequence in JavaScript, we can utilize a simple loop. This method is efficient and easy to understand, making it a great choice for beginners. Here’s how you can implement it:
function generateFibonacci(n) {
let fibSequence = [0, 1];
for (let i = 2; i < n; i++) {
fibSequence[i] = fibSequence[i - 1] + fibSequence[i - 2];
}
return fibSequence.slice(0, n);
}
console.log(generateFibonacci(10));
Output:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34
In this code, we define a function called generateFibonacci
that takes a single parameter, n
, which represents how many Fibonacci numbers we want to generate. We initialize an array, fibSequence
, with the first two Fibonacci numbers, 0 and 1. The loop starts at index 2 and continues until it reaches n
. Inside the loop, we calculate each subsequent number by summing the two preceding numbers, which are accessed via their indices in the array. Finally, we return a sliced version of the array to ensure we only get the first n
Fibonacci numbers.
Using Recursion to Generate Fibonacci
While loops are straightforward, recursion offers another elegant way to generate Fibonacci numbers. This method can be less efficient for larger values of n
due to repeated calculations, but it’s an excellent way to understand the recursive nature of the Fibonacci sequence.
function fibonacciRecursive(n) {
if (n <= 1) return n;
return fibonacciRecursive(n - 1) + fibonacciRecursive(n - 2);
}
let fibSequence = [];
for (let i = 0; i < 10; i++) {
fibSequence.push(fibonacciRecursive(i));
}
console.log(fibSequence);
Output:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34
In this code, we define a recursive function fibonacciRecursive
that calculates the Fibonacci number at position n
. If n
is 0 or 1, it returns n
. Otherwise, it calls itself twice, summing the results of fibonacciRecursive(n - 1)
and fibonacciRecursive(n - 2)
. We then use a loop to populate an array with the first ten Fibonacci numbers by calling our recursive function. This method beautifully illustrates the recursive definition of the Fibonacci sequence, although it may not be the most efficient for large inputs.
Conclusion
Creating a Fibonacci sequence in JavaScript can be accomplished in a variety of ways, with loops and recursion being two of the most popular methods. while
loops provide a straightforward and efficient approach, recursion offers a deeper insight into the mathematical properties of the Fibonacci sequence. Depending on your needs and preferences, you can choose the method that best suits your project. By understanding these techniques, you can enhance your programming skills and tackle more complex problems with confidence. Happy coding!
FAQ
-
What is the Fibonacci sequence?
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, starting from 0 and 1. -
Can I generate Fibonacci numbers in other programming languages?
Yes, Fibonacci sequences can be generated in virtually any programming language using similar logic. -
What are the practical applications of the Fibonacci sequence?
The Fibonacci sequence appears in various fields, including computer science, mathematics, and even nature, such as in the arrangement of leaves or the branching of trees. -
Is recursion always better than loops for generating Fibonacci numbers?
Not necessarily. While recursion is elegant, it can be less efficient due to repeated calculations. Loops are generally more efficient for generating Fibonacci numbers. -
How can I improve the performance of a recursive Fibonacci function?
You can improve performance by using memoization, which stores previously calculated results to avoid redundant calculations.