The debounce() Function in JavaScript
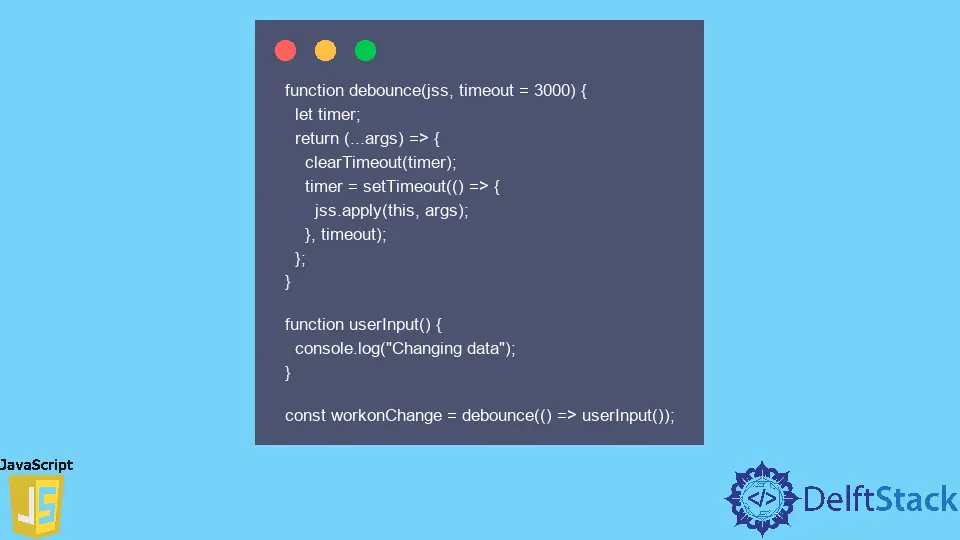
This article will teach you how to utilize the JavaScript debounce()
function to enhance the performance of your applications.
Understanding the Term Debounce
in JavaScript
JavaScript uses the debouncing technique to speed up browser performance. A debounce is a throttle’s relative, and they both enhance the performance of the online application.
Nevertheless, they are observed in many contexts. A debounce is employed when all we consider is the end state.
For instance, they are delaying the retrieval of type-ahead search results until the user has done typing. The ideal tool to utilize is a throttle if we want to control the speed of all intermediate states.
Use the debounce()
Function in JavaScript
Here’s a code illustration to help you understand how to debounce a function:
function debounce(jss, timeout = 3000) {
let timer;
return (...args) => {
clearTimeout(timer);
timer = setTimeout(() => {
jss.apply(this, args);
}, timeout);
};
}
function userInput() {
console.log('Changing data');
}
const workonChange = debounce(() => userInput());
So, what’s going on here? The debounce()
is a unique function that performs two functions:
- Assigning the timer variable a scope
- Setting up your function to run at a specific time
Let’s look at the first use case with text input to see how this works.
The debounce()
function initially resets the timer using clearTimeout
when a visitor writes the first letter and releases the key (timer). The step is not required at this time because nothing is scheduled.
The function userInput()
is expected to be called in 3000 milliseconds (3 seconds). However, if the visitor continues to type, each essential release will re-trigger the debounce()
function.
Every invocation must reset the timer, or, in other words, cancel any earlier userInput()
preparations and reschedule it for a new time - 3000 milliseconds in the future. This will continue as long as the visitor maintains the keystrokes within 3000 milliseconds.
Because the previous schedule will not be cleared, userInput()
will be invoked.
Output:
You’ll see that the setTimeout
method keeps deferring when you type s
, h
, i
, or v
(despite the called function userInput
). When we pause for three seconds while typing, the primary function is printed: Changing data
.
Debouncing improves application speed by only invoking functions after a set amount of pause time rather than on every operation. You do not, however, have to accomplish this on your own.
A debounce
function is available in Lodash, for example. Contributors have improved libraries such as Lodash to improve the debounce
function.
We have discussed what debouncing is, why it’s useful, and how to do it in JavaScript in this article.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn