JavaScript call vs apply vs bind
-
the
function.call()
Method in JavaScript -
the
function.apply()
Method in JavaScript -
the
bind()
Method in JavaScript
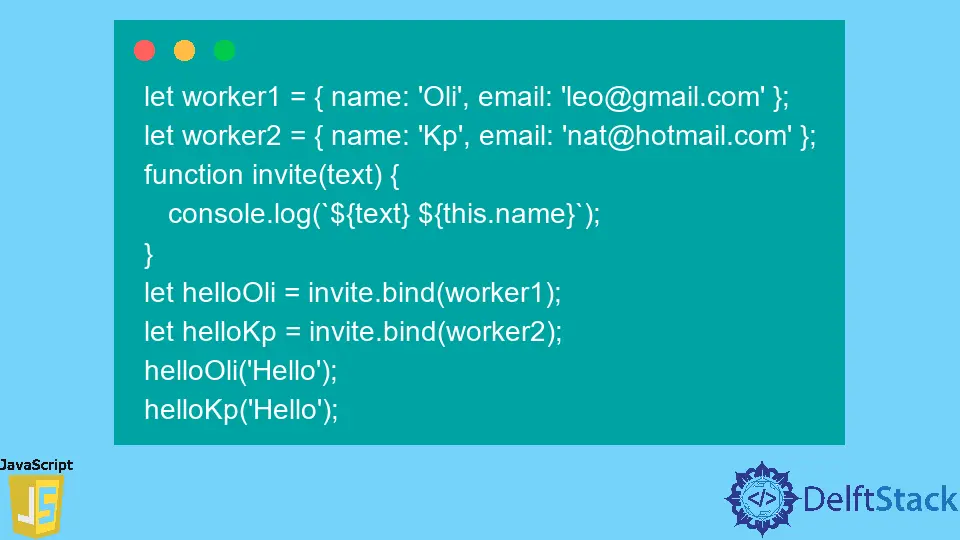
We’ll explain the differences between apply()
, call()
, and bind()
methods today. Using these JavaScript techniques, you can modify the value of this
for a given function.
the function.call()
Method in JavaScript
The function.call()
method calls the function and allows you to send parameters one at a time using commas. Herein mentioned example, this refers to the person object, and this.name
is the name of worker1
and worker2
.
Example:
let worker1 = {name: 'Oli', email: 'oli@gmail.com'};
let worker2 = {name: 'Kp', email: 'kp@hotmail.com'};
function invite(text) {
console.log(`${text} ${this.name}`);
}
invite.call(worker1, 'Hello Upwork');
invite.call(worker2, 'Hello Upwork');
Output:
"Hello Upwork Oli"
"Hello Upwork Kp"
the function.apply()
Method in JavaScript
You can call a function with a supplied this
value and parameters provided as an array using the function.apply()
method. The apply()
and call()
methods are similar, but instead of individual arguments, it takes the function’s parameters as an array.
Example:
let worker1 = {name: 'Oli', email: 'oli@gmail.com'};
let worker2 = {name: 'Kp', email: 'kp@hotmail.com'};
function invite(text, text2) {
console.log(`${text} ${this.name}, ${text2}`);
}
invite.apply(worker1, ['Hello', 'How are you?']);
invite.apply(worker2, ['Hello', 'How are you?']);
Output:
"Hello Oli, How are you?"
"Hello Kp, How are you?"
the bind()
Method in JavaScript
The bind()
method makes a new function that takes this array and any number of arguments. Use it when you want to invoke a function later with a specific context, such as events.
Example:
let worker1 = {name: 'Oli', email: 'leo@gmail.com'};
let worker2 = {name: 'Kp', email: 'nat@hotmail.com'};
function invite(text) {
console.log(`${text} ${this.name}`);
}
let helloOli = invite.bind(worker1);
let helloKp = invite.bind(worker2);
helloOli('Hello');
helloKp('Hello');
Output:
"Hello Oli"
"Hello Kp"
The following example is the Bind implementation.
Example:
Function.prototype.bind = function(context) {
var func = this;
return function() {
func.apply(context, arguments);
};
};
The terms call
and apply
are interchangeable. You can choose whether an array or a comma-separated list of arguments is more convenient. Bind
is not like the others.
It returns a new function every time. As shown in the example, we can use Bind
to curry functions.
We can make a "helloOli"
or "helloKp"
out of a simple hello operation. It can be used for events when we don’t know when they’ll be fired but what context they’ll be in.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn