How to Detect Arrow Key Presses in JavaScript
- What Are Events
-
What Are the Most Common
EventListeners
in JavaScript - Keydown Event Listener in JavaScript
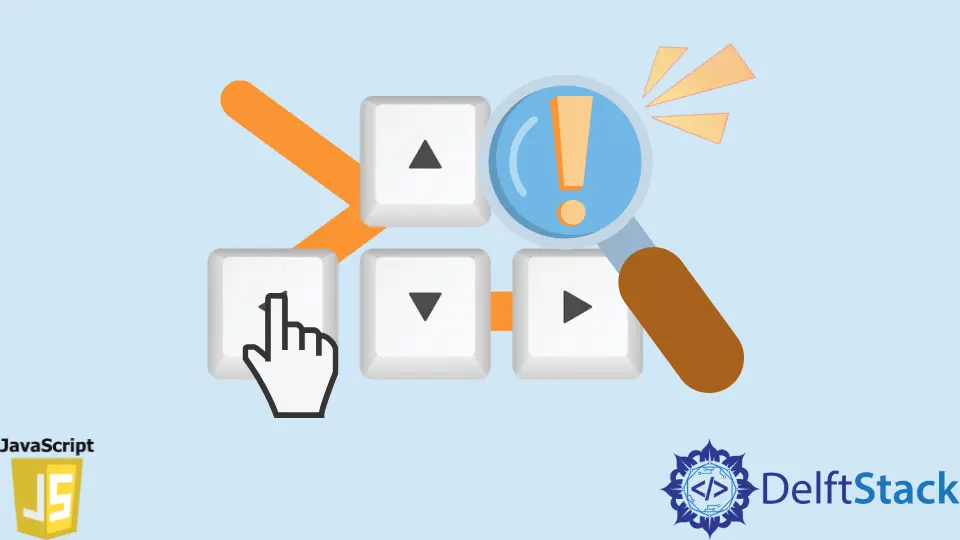
This tutorial explains how you can create shortcuts and set up hotkeys by using certain built-in functions that listen to the user’s input in JavaScript. This article will also include a detailed description of Events and EventListeners in JavaScript.
What Are Events
In this coding perspective, the physical activity we perform causes an event. These events are the driving force of program execution; it’s helpful when working with Graphical User Interface (GUI).
We can make these shortcuts and hotkeys by using an event listener
procedure that listens for a particular event and passes on this information to the handler to handle the event.
In simple words, takes an input of the key (mouse or keyboard) pressed, we can also use an event handler
directly that processes these events. We will demonstrate both paradigms in depth.
What Are the Most Common EventListeners
in JavaScript
There are many event handlers in JS, these two are the most common ones, and their functions are:
keydown
: registers when you press a key and continuously keeps registering if you hold itkeyup
: registers when you release the key
Keydown Event Listener in JavaScript
The .onkeydown
event handler tells the compiler to run a certain function()
as soon as the desired key is pressed; by placing an alert("message")
, we can display an alert box containing a specified message.
In the code below, we use two event properties, .key
, which will return the label of the key pressed, and .keyCode
that returns the code of the particular key. These keycodes are like ASCII since each key is mapped to a particular alphanumeric value.
In our example, we enter k
as the function parameter.
document.onkeydown = function(e) {
alert(e.key + e.keyCode); // shows k75
};
As soon as a particular key is pressed, we get an alert that shows the key pressed concatenated with its key code. Key labels and key codes make it easier to manipulate code logic and are widely used in event-driven operations.
Let’s look at an example that shows how we can use these parameters to our advantage.
document.onkeydown = function(e) {
switch (e.keyCode) {
case 37:
alert('left'); // show the message saying left"
break;
case 38:
alert('up'); // show the message saying up"
break;
case 39:
alert('right'); // show the message saying right"
break;
case 40:
alert('down'); // show the message saying down"
break;
}
};
Here, we use a simple switch case that takes in the key pressed key code, checks which case it belongs, and evaluates it to display a message. Let’s say that we press the left arrow key; then it will display the left
alert, and so on.
We can also define event listener in this manner:
document.addEventListener('keydown', function(event) {});
Here we take a look at another paradigm and how it’s different from our previous approach:
document.addEventListener('keydown', function(event) {
if (event.key == 'ArrowLeft') {
alert('Left key'); // show the message saying Left key"
} else if (event.key == 'ArrowUp') {
alert('Up key'); // show the message saying Up key"
} else if (event.key == 'ArrowRight') {
alert('Right key'); // show the message saying Right key"
} else if (event.key == 'ArrowDown') {
alert('Down key'); // show the message saying Down key"
}
});
This code seems like it gives the same output as our previous code. Still, there’s a catch, here instead of using key codes explicitly to play around with our logic, we use key labels such as Arrow down
and Arrow up
that directly and compare them to display the desired message.
Let’s say we pressed the up arrow, then our if
code blocks will check if the key label returned from the function matches with the given key labels. If it does, then that block is executed, displaying a message.
Now, we don’t have to remember the key codes to each key when we can remember the mnemonic key labels that are way easier to remember. This is extremely handy when we do not know the key codes and dabble with logic.