How to Display Variable Value in an Alert Box in JavaScript
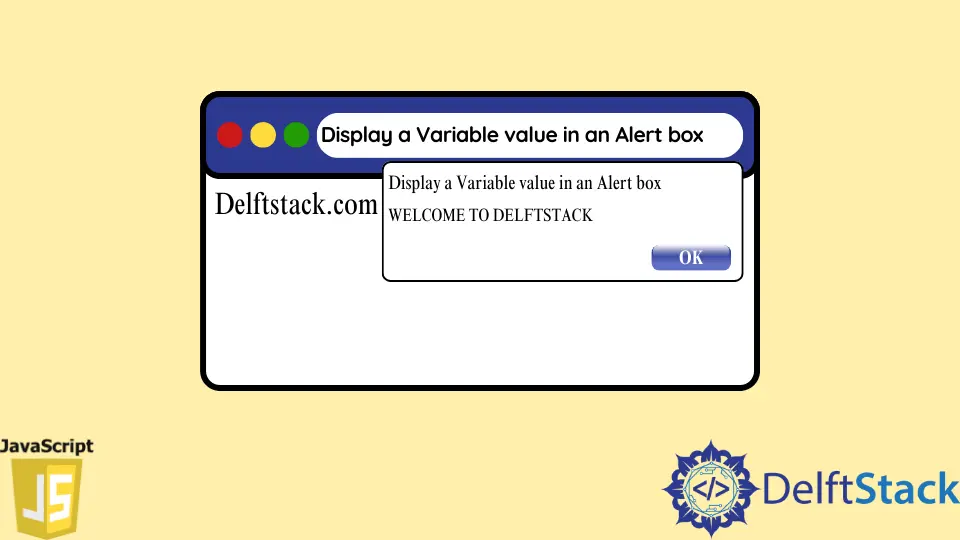
In this article, we will learn to use the alert()
method in JavaScript. We will learn how to use an alert box to display a message or any other useful information as a popup to the user on a web page.
Display Variable Value in an Alert Box in JavaScript
To display any value in the popup, we must use an alert box. By default, it contains the OK
button to hide and dismiss the popup dialog.
When a user interacts with a web page, most of the time, developers need to convey a useful message to the user with the help of an alert box. For example, when users upload their data to the server from the client-side, web pages show a success or failure message in the popup dialog after validation.
The alert box removes the user’s focus from the active web page, forcing the user to read the alert message.
In JavaScript, the default alert()
method can display an alert message, variable value, and text along with a variable with the help of the concatenation operator +
.
Basic Syntax:
let data = 'hello world'
alert(data);
As shown above, we only need to pass a value or variable to the alert()
method as an argument.
Example:
<html>
<head>
<title> display JavaScript alert </title>
</head>
<script>
function showPopup()
{
var data = document.getElementById("value").value;
//check empty data field
if(data == "")
{
alert("Please enter any value..");
}
else
{
alert("Your entered value is: "+data); //display variable along with the string
}
}
</script>
<body>
<h1 style="color:blueviolet">DelftStack</h1>
<h3>JavaScript display alert box</h3>
<form onsubmit ="return showPopup()">
<!-- data input -->
<td> Enter Value: </td>
<input id = "value" value = "">
<br><br>
<input type = "submit" value = "Submit">
</form>
</body>
</html>
We used the form
element in the above HTML source code to create the user input field and the Submit
button. The user will insert a value, and when the user clicks the Submit
button, the function showPopup()
will be triggered.
In the script tags, we have declared the showPopup()
function, where we get the user input value in the data
variable and use the conditional statement if
to check if data
is empty or not.
If the data
variable is empty, it will display an alert with an error message. Otherwise, it will show a success message with the data
variable.