How to Generate Random Color in JavaScript
-
Using
Math.floor(Math.random())
to Generate a Random Color Using JavaScript in HTML -
Use the
hsla()
Function for JavaScript Random Color Generation
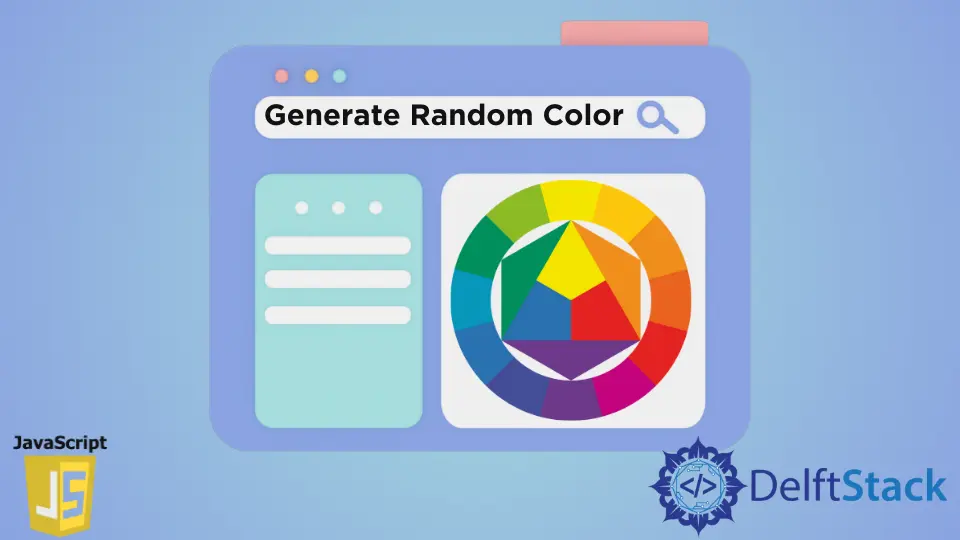
This article will discuss the usage of JavaScript built-in Math functions and JQuery library for UI (user interface) to change the background color of HTML webpage onClick
randomly by an example. Using Math functions, we can easily get the rounded values as floor and ceiling, and we can get a random value using math.random()
.
Using Math.floor(Math.random())
to Generate a Random Color Using JavaScript in HTML
The getRandomColor
method is triggered whenever the user presses the Change background color
button. That method uses JQuery to assign CSS styles to our body tag of the HTML web page that will change the background color.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Random colors</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css">
<script src="https://code.jquery.com/jquery-1.12.4.js"></script>
<script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
</head>
<body id ="bodyId" >
<Div style="text-align: center; width: 100%;">
<H1>Generate Random Color using JavaScript/jquery</H1>
<button onclick="getRandomColor()">Change background color</button>
</Div>
</body>
<script>
//The ColorCode() will give the code every time.
function ColorCode() {
var makingColorCode = '0123456789ABCDEF';
var finalCode = '#';
for (var counter = 0; counter < 6; counter++) {
finalCode =finalCode+ makingColorCode[Math.floor(Math.random() * 16)];
}
return finalCode;
}
//Function calling on button click.
function getRandomColor() {
$("#bodyId").css("background-color", ColorCode());
}
</script>
</html>
In the above HTML
page source, you can see JQuery links in <script>
tags that are necessary to use JQuery
libraries in doctype html. In the next step, the function getRandomColor()
is declared inside of the <script>
tag inside another function called function ColorCode()
that assigns a CSS style with property background-color.
The function ColorCode()
contains a string of Hexa decimal values in sequence (0-F
) we used a for
loop to execute the code statements until the given condition is true. In this case, the condition to be met is var counter = 0; counter < 6; counter++
.
That loop will generate a finalCode
string by concatenating random values with #
length of 6
, then we return that finalCode
string and use it as the color attribute.
Examine the Given HTML
Code
Follow these four simple steps to understand the getRandomColor
method.
-
Create a text document. You can use notepad or any other text editing tool.
-
Paste the given code in the created text file.
-
Save that text file with the extension of
.html
and open it with any default browser. -
You can see the button
Change background color
. Using that button, you can change the background color randomly.
Use the hsla()
Function for JavaScript Random Color Generation
Do you want to build a random dark color generator using JavaScript? This method will use hsla
which stands for hue
, saturation
, lightness
, and alpha
. It is the extension of hsl
. The hsla
function shows the bright colors according to the value of its four parameters.
// Syntax of HSLA
hsla(hue, saturation, lightness, alpha);
Following is the startup code for you.
HTML Code:
<html>
<head>
<title>Random Color Generator</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
</head>
<body>
<div id="color_box"></div>
<br />
<button id="rgcbtn" onclick="generateRandomColor()">
Generate Random Color
</button>
</body>
</html>
CSS Code:
#color_box {
width: 200px;
height: 200px;
background-color: #000;
}
#rgcbtn {
font-size: 15px;
fornt-weight: bold;
}
JavaScript Code:
function generateRandomColor() {
$('#color_box')
.css(
'background-color',
'hsla(' + Math.random() * 360 + ', 100%, 50%, 1)');
}
In this code, the function named generateRandomColor()
gets executed if someone clicks on the button whose id’s value is rgcbtn
. The random number is calculated using Math.random()
function and passed on the position of hue
parameter. The values of saturation
, lightness
and alpha
are 100%
, 50%
, and 1
. The .css()
function sets the background-color
with the value returned by hsla
function.
OUTPUT: