How to Get Full-Screen Window in JavaScript
- Understanding the Full-Screen API
- Method 1: Using requestFullscreen() to Enter Full-Screen Mode
- Method 2: Exiting Full-Screen Mode with exitFullscreen()
- Method 3: Handling Full-Screen Change Events
- Conclusion
- FAQ
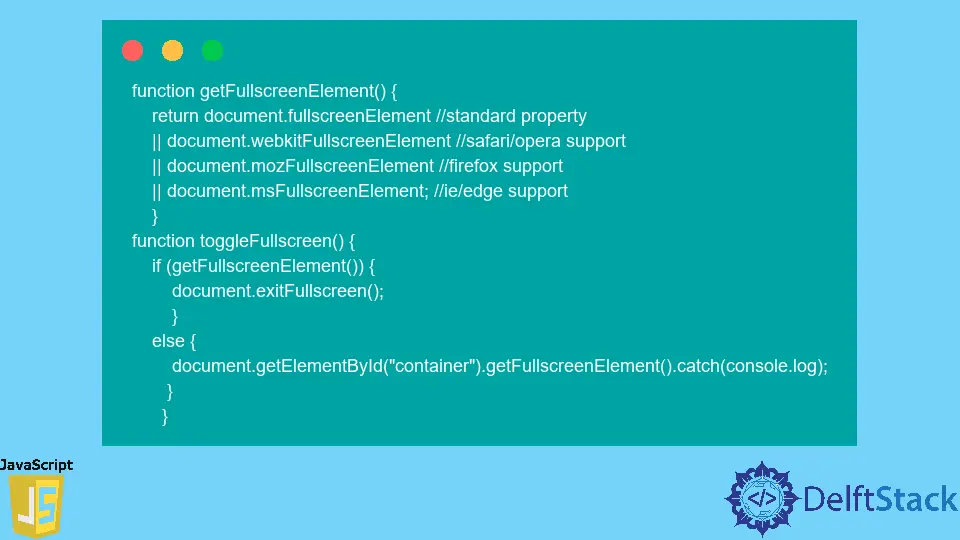
In the world of web development, creating an immersive experience for users can significantly enhance engagement. One way to achieve this is by utilizing the full-screen capabilities of modern browsers.
This tutorial demonstrates how to get the full-screen window using JavaScript. Whether you’re building a game, a video player, or a presentation tool, knowing how to manipulate the full-screen API can be invaluable. In this guide, we’ll walk through the steps to achieve full-screen functionality, ensuring your application captures the user’s attention and provides a seamless experience. Let’s dive into the details!
Understanding the Full-Screen API
The Full-Screen API is a powerful feature that allows web applications to present content in full-screen mode. This API is supported by most modern browsers and provides a straightforward way to enhance user experience. By using the Full-Screen API, developers can enable users to view content without distractions from the browser’s interface.
To implement this functionality, we typically use the methods requestFullscreen()
and exitFullscreen()
. The former allows an element to be displayed in full-screen mode, while the latter exits full-screen mode.
Method 1: Using requestFullscreen() to Enter Full-Screen Mode
To enter full-screen mode, you can use the requestFullscreen()
method on the desired element. Below is a simple example that demonstrates how to implement this in JavaScript.
const btn = document.getElementById('fullscreen-btn');
const elem = document.getElementById('myElement');
btn.addEventListener('click', () => {
if (elem.requestFullscreen) {
elem.requestFullscreen();
} else if (elem.mozRequestFullScreen) {
elem.mozRequestFullScreen();
} else if (elem.webkitRequestFullscreen) {
elem.webkitRequestFullscreen();
} else if (elem.msRequestFullscreen) {
elem.msRequestFullscreen();
}
});
Output:
Element goes full screen when the button is clicked.
In this code, we first select the button and the element we want to display in full-screen mode. When the button is clicked, we check for the availability of the requestFullscreen()
method on the element. If it’s available, the element enters full-screen mode. The code also includes vendor prefixes for compatibility with older browsers, such as Firefox, WebKit, and Internet Explorer.
Method 2: Exiting Full-Screen Mode with exitFullscreen()
Just as important as entering full-screen mode is the ability to exit it. The exitFullscreen()
method allows users to return to their normal view. Here’s how you can implement this functionality.
const exitBtn = document.getElementById('exit-fullscreen-btn');
exitBtn.addEventListener('click', () => {
if (document.exitFullscreen) {
document.exitFullscreen();
} else if (document.mozCancelFullScreen) {
document.mozCancelFullScreen();
} else if (document.webkitExitFullscreen) {
document.webkitExitFullscreen();
} else if (document.msExitFullscreen) {
document.msExitFullscreen();
}
});
Output:
Exits full screen when the exit button is clicked.
In this snippet, we add an event listener to the exit button. When clicked, the code checks if the exitFullscreen()
method is available. If it is, the document exits full-screen mode. Similar to the previous method, we account for browser compatibility by including vendor-specific methods.
Method 3: Handling Full-Screen Change Events
To enhance user experience further, you might want to handle events that occur when the full-screen state changes. This can help you trigger specific actions or update the UI accordingly. Below is an example of how to listen for these events.
document.addEventListener('fullscreenchange', () => {
if (document.fullscreenElement) {
console.log('Entered full-screen mode');
} else {
console.log('Exited full-screen mode');
}
});
Output:
Logs the current full-screen status to the console.
In this example, we listen for the fullscreenchange
event on the document. When the event fires, we check if there is a full-screen element. If there is, we log that the user has entered full-screen mode; otherwise, we log that they have exited. This can be particularly useful for updating UI elements or providing feedback to the user.
Conclusion
Mastering the Full-Screen API in JavaScript can significantly enhance the user experience of your web applications. By utilizing methods like requestFullscreen()
and exitFullscreen()
, as well as handling full-screen change events, you can create an immersive environment for your users. With this tutorial, you now have the tools to implement full-screen functionality effectively. Whether for games, videos, or presentations, full-screen mode can make your application stand out. Experiment with these methods and see how they can transform your projects!
FAQ
-
What browsers support the Full-Screen API?
Most modern browsers, including Chrome, Firefox, Safari, and Edge, support the Full-Screen API. -
Can I make any element go full-screen?
Yes, you can make almost any HTML element go full-screen, but it’s common to use it for video players, images, or entire web pages. -
How do I know if my element is currently in full-screen mode?
You can check ifdocument.fullscreenElement
is not null to determine if any element is in full-screen mode. -
Are there any limitations to using the Full-Screen API?
Yes, some limitations include that full-screen mode can only be triggered by user actions (like clicks) and may behave differently across various devices and browsers. -
How can I style my application when in full-screen mode?
You can use CSS to style your elements specifically when they are in full-screen mode by using the:fullscreen
pseudo-class.