How to Calculate the Median in JavaScript
- Understanding the Median
- Method 1: Using a Simple Function
- Method 2: Utilizing Array Methods
- Method 3: Handling Edge Cases
- Conclusion
- FAQ
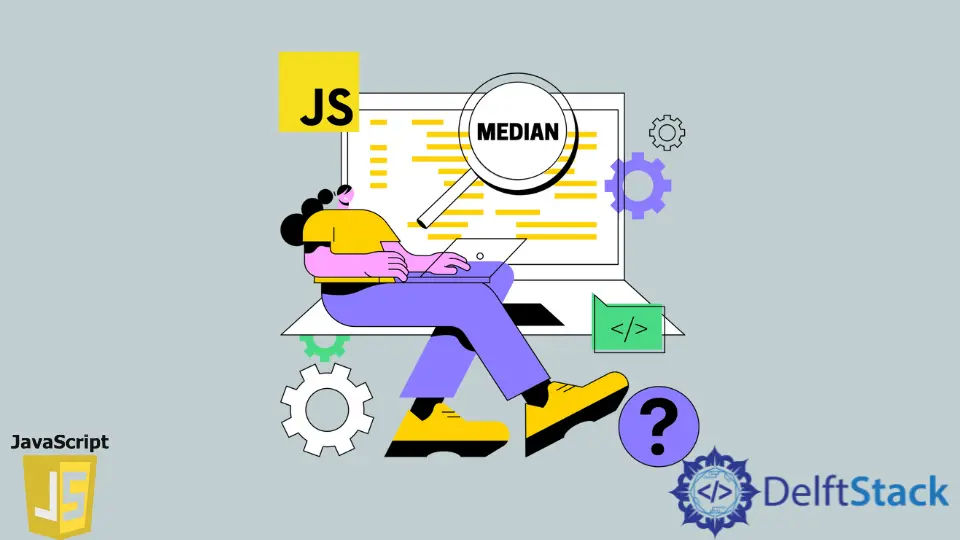
Calculating the median is a fundamental statistical operation that can be particularly useful in data analysis and programming. In JavaScript, finding the median of a list of values is straightforward, but it requires a clear understanding of the steps involved. The median is the middle value in a list of numbers, which means that half of the numbers are above it and half are below it.
In this tutorial, we will explore how to calculate the median in JavaScript, providing you with clear code examples and explanations. Whether you’re working with arrays of numbers in your applications or simply want to enhance your JavaScript skills, this guide is designed for you.
Understanding the Median
Before diving into the code, it’s essential to grasp what the median is. The median is a measure of central tendency, which means it helps to identify the center point of a data set. To find the median, you must first sort the values in ascending order. If the number of values is odd, the median is the middle number. If it’s even, the median is the average of the two middle numbers.
For example, consider the array [3, 1, 4, 2]. When sorted, it becomes [1, 2, 3, 4]. Since there are four numbers (even), the median is (2 + 3) / 2 = 2.5. Now that we understand the concept, let’s move on to the implementation in JavaScript.
Method 1: Using a Simple Function
One of the most straightforward ways to calculate the median in JavaScript is by writing a simple function that sorts the array and then finds the median based on the number of elements.
function calculateMedian(arr) {
arr.sort((a, b) => a - b);
const mid = Math.floor(arr.length / 2);
return arr.length % 2 !== 0 ? arr[mid] : (arr[mid - 1] + arr[mid]) / 2;
}
const numbers = [5, 3, 8, 1, 2];
const median = calculateMedian(numbers);
console.log(median);
Output:
3
In this function, we first sort the array using the sort
method. The sorting function (a, b) => a - b
ensures that numbers are ordered from smallest to largest. After sorting, we determine the midpoint of the array. If the array length is odd, we return the middle value directly. If it’s even, we calculate the average of the two middle values. This method is efficient for small to medium-sized arrays and provides a clear and concise way to find the median.
Method 2: Utilizing Array Methods
Another effective approach to calculate the median in JavaScript is by leveraging built-in array methods. This method can be particularly useful if you prefer a more functional programming style.
const calculateMedian = (arr) => {
const sorted = arr.slice().sort((a, b) => a - b);
const mid = Math.floor(sorted.length / 2);
return sorted.length % 2 === 0
? (sorted[mid - 1] + sorted[mid]) / 2
: sorted[mid];
};
const values = [7, 5, 3, 9, 1];
const medianValue = calculateMedian(values);
console.log(medianValue);
Output:
5
In this example, we use an arrow function for a more modern JavaScript syntax. The slice()
method creates a shallow copy of the array to avoid mutating the original data. After sorting, we calculate the midpoint similarly to the previous method. This approach is elegant and takes advantage of the expressive capabilities of JavaScript’s array methods, making it a favorite among many developers.
Method 3: Handling Edge Cases
When calculating the median, it’s crucial to consider edge cases, such as empty arrays or arrays with non-numeric values. Here’s how you can enhance the previous functions to handle these scenarios.
function calculateMedian(arr) {
if (!Array.isArray(arr) || arr.length === 0) {
return null;
}
const filtered = arr.filter(num => typeof num === 'number');
filtered.sort((a, b) => a - b);
const mid = Math.floor(filtered.length / 2);
return filtered.length % 2 !== 0
? filtered[mid]
: (filtered[mid - 1] + filtered[mid]) / 2;
}
const mixedValues = [5, 'a', 3, null, 9];
const medianMixed = calculateMedian(mixedValues);
console.log(medianMixed);
Output:
5
In this method, we first check if the input is a valid array and whether it contains any elements. If not, we return null
. We also filter out non-numeric values to ensure that our calculations are accurate. This approach makes the function more robust and reliable in real-world applications, ensuring that it can handle a variety of inputs gracefully.
Conclusion
Calculating the median in JavaScript is a valuable skill that can enhance your programming toolkit. Whether you’re working on data analysis, statistical applications, or just improving your coding skills, understanding how to find the median will serve you well. We explored several methods, from simple functions to more advanced techniques that handle edge cases. By implementing these strategies, you can efficiently calculate the median in any JavaScript application.
FAQ
-
What is the median?
The median is the middle value in a list of numbers, separating the higher half from the lower half. -
How do I calculate the median of an array in JavaScript?
You can calculate the median by sorting the array and finding the middle value or the average of the two middle values. -
What if my array contains non-numeric values?
You should filter out non-numeric values before calculating the median to ensure accurate results. -
Can I use this method for large datasets?
Yes, but for very large datasets, consider using more efficient algorithms or libraries designed for statistical calculations. -
Is there a built-in method in JavaScript to calculate the median?
No, JavaScript does not have a built-in method for calculating the median, so you need to implement it manually.