How to Clear All Cookies With JavaScript
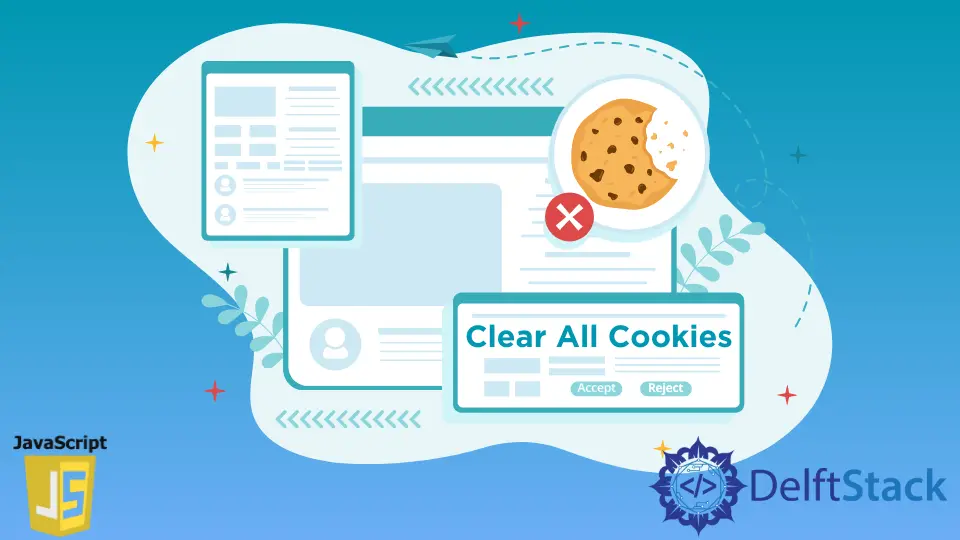
This article will help you to clear all cookies using JavaScript.
Cookies
allow clients and servers to communicate and transfer information over HTTP. It allows the client to persist state information even when using the stateless protocol HTTP.
- Information about the cookie is sent in HTTP request headers on each subsequent visit to the domain from the user’s browser.
- Information such as login information, consent, and other parameters improves and personalizes the user experience.
Delete All Cookies for the Current Domain Using JavaScript
The cookie attribute in the current document is used to change the attributes of a cookie purchased using the HTML DOM cookie
attribute. The document.cookie
returns a string of all semicolon-delimited cookies associated with the current document.
Syntax:
document.cookie = 'key=value';
The code below shows how to delete cookies using JavaScript. The code is run on an online editor to demonstrate that the code can delete only cookies generated by your site.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>
</title>
</head>
<body>
<main>
<script type="text/javascript">
document.cookie = "username=shiv";
document.cookie = "CONSENT=YES+IN.en+20170903-09-0";
function displayCookies() {
var displayCookies = document.getElementById("display");
displayCookies.innerHTML = document.cookie;
}
function deleteAllCookies() {
var cookies = document.cookie.split(";");
for (var i = 0; i < cookies.length; i++) {
var cookie = cookies[i];
var eqPos = cookie.indexOf("=");
var name = eqPos > -1 ? cookie.substr(0, eqPos) : cookie;
document.cookie = name + "=;expires=Thu, 01 Jan 1970 00:00:00 GMT";
}
}
</script>
<button onclick="displayCookies()">Display Cookies</button>
<button onclick="deleteAllCookies()">Delete Cookies</button>
<p id="display"></p>
</main>
</body>
</html>
The code above has two limitations.
- Cookies with the
HttpOnly
flag set are not deleted because theHttpOnly
flag disables JavaScript access to the cookie. - Cookies set as path values are not deleted. (Although these cookies appear under
Deleted
, they cannot be deleted without specifying the same value for the installation path.)
Output:
When you click on Display Cookies
, it will display the cookies.
You can also view the cookies in the inspector.
After clicking on Delete Cookies
, it will delete it, and you have to again click on display cookies to see whether it is deleted or not.
You can also see in the inspector whether it is deleted or not.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn