How to Change the Class of an HTML Element With JavaScript
- Select an HTML Element in JavaScript
- Change All Classes Applicable to an Element in JavaScript
- Insert an Extra Class to the Element in JavaScript
- Delete a Class in an Element in JavaScript
- Check if a Class Has Been Used in an Element in JavaScript
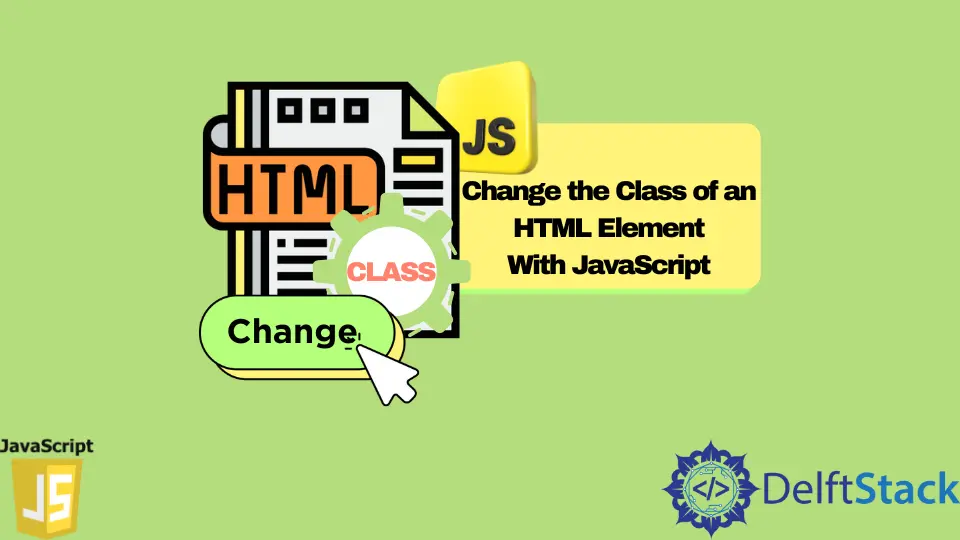
This article demonstrates how to change the class of an HTML element in response to JavaScript events.
Select an HTML Element in JavaScript
Inarguably, the easiest way to select an HTML element in JavaScript is through the document.getElementById()
.
For instance, if you need to select an element with the class ID Students, here’s how to select it.
document.getElementById('Students')
Change All Classes Applicable to an Element in JavaScript
After selecting the element using its class ID, you can change its class or replace all its classes, as shown above. You will need to set a new one using the .className
attribute to replace the old class, as shown below.
Document.getElementById('Fruits').classname = 'Veggies';
Insert an Extra Class to the Element in JavaScript
You can also add a new class to an element without changing any of the existing classes. You can do this by using the +=
operator to add the new class.
For example,
Document.getElementById('Subjects').className += 'Physics';
It adds an extra class named Physics to other classes in the Subjects element.
Delete a Class in an Element in JavaScript
Deleting a single class in an element without affecting other classes is slightly more complex. You will need to use the .className.replace
together with the following regex.
?:^|\s)RemoveThis(?!\S)/g
?:^|\s
captures the beginning of a string and/or any single whitespace character used.RemoveThis
is the name of the class to be removed.(?!\S)
verifies thatRemoveThis
is a full class name and checks for any non-space characters at the end. In other words, it ensures that the regex is placed at the end of the string.g
is the flag that ensures that the replace command is effected as necessary in all cases where the class has been added.
See the below example.
document.getElementById('ElementOne').className =
document.getElementById('ElementOne')
.className.replace(/(?:^|\s)RemoveThis(?!\S)/g, '')
Check if a Class Has Been Used in an Element in JavaScript
To check whether a class has been applied to a specific element, you can use the regex above . This Is best achieved using an if();
statement to give a result. For instance:
if (document.getElementById('ElementOne')
.className.match(/(?:^|\s)ClassOne(?!\S)/))
print('Class has been used.')
The output will be Class has been used
if the check comes out positive, or no output if it is negative unless an alternative action or output is defined with the else
statement.
It is not recommendable to write JavaScript code directly in HTML event attributes. It could make it harder to execute and slow down applications. Instead, you can write the JavaScript in a function, calling the said function in an onclick
attribute then moving the event from HTML code to JavaScript. You can achieve this with the .addEventListener()
attribute.