apply() vs call() Methods in JavaScript
-
the
call()
Method in JavaScript -
the
apply()
Method in JavaScript -
the
call()
Method Example in JavaScript -
Alternative Way by Using the
apply()
Method
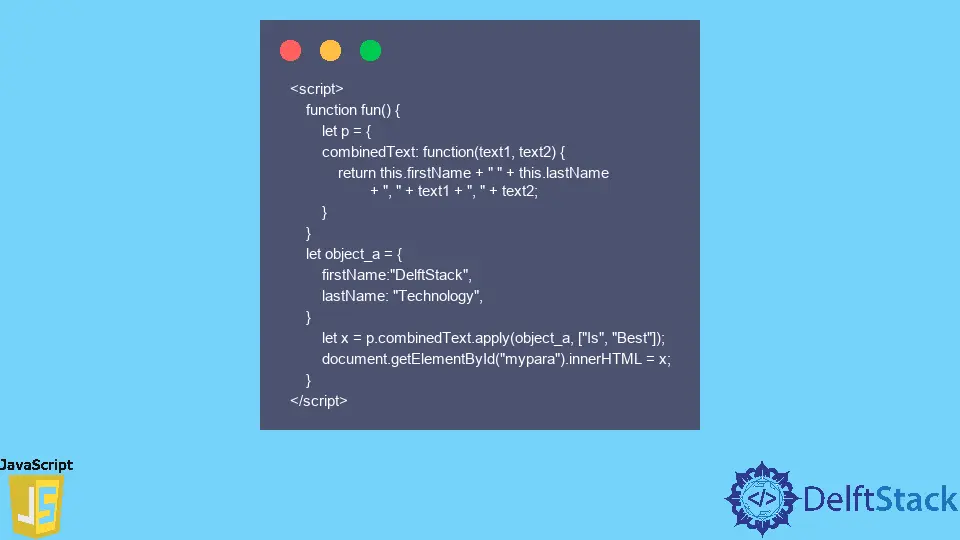
This article explains how to use the call()
and apply()
methods in JavaScript. The difference between both functions is explained using some easy examples.
Let us have a look at the following methods and how they work.
the call()
Method in JavaScript
Taking the owner object as an argument is the call()
method. The keyword this
refers to the owner
of the function or the object it belongs to.
We can also call a method to utilize it on different objects. It will let us invoke the methods with comma-separated arguments.
Syntax of the call()
Method in JavaScript
abc_object.object_method.call(object_instance, arguments, ...)
As mentioned above, the call()
method accepts two parameters, which we will describe below.
- In the first parameter,
object_instance
will hold the instance of an object. - Comma-separated arguments are in the second parameter.
the apply()
Method in JavaScript
apply()
is used to write on a different object. It takes the values in the form of an array.
Syntax of the apply()
Method in JavaScript
abc_object.object_method.apply(object_instance, [arguments_array...])
The apply()
method accepts two parameters as displayed above.
- In the first parameter,
object_instance
will hold the instance of an object. - In the second parameter, the arguments are in the form of an array.
the call()
Method Example in JavaScript
We will make a simple button, Click to display
, that will trigger a function on click event.
The function will use the call()
method, and we will display a combined text result using objects that contain multiple string values as key-value pairs. It will simply combine strings of the given object by passing a parameter using the call()
method.
The result will be displayed as a paragraph.
<!DOCTYPE html>
<html>
<head>
<title>
call() method Example
</title>
</head>
<body style = "text-align:center;">
<h1 >
Click on "Click to display" for display combined Text using call()
</h1>
<button onClick="fun()">
Click to display
</button>
<p id="mypara"></p>
<!-- Script tags to use call() method to call
function -->
<script>
function fun() {
let object_b = {
combinedText: function(text1, text2) {
return this.firstName + " " + this.lastName
+ " " + text1 + " " + text2;
}
}
let object_a = {
firstName:"DelftStack",
lastName: "Technology",
}
let x = object_b.combinedText.call(object_a, "is", "Best");
document.getElementById("mypara").innerHTML = x;
}
</script>
</body>
</html>
In the above HTML
page source, you can see a simple button Click to display
that calls the function fun()
.
In the fun()
body is initialized with another combinedText
method in object_b
. It returns a concatenated string of passed parameters.
Another initialized object object_a
that contains multiple strings as a key-value using call()
parameters (object_a,"is","best")
. Both concatenate in the string and return the result.
The result is displayed by the getElementById()
.
Alternative Way by Using the apply()
Method
Same results are achievable by the apply()
method. You can use the apply()
method by passing array of arguments instead of comma separated arguments object_b.combinedText.apply(object_a, ["is", "Best"])
.
<script>
function fun() {
let p = {
combinedText: function(text1, text2) {
return this.firstName + " " + this.lastName
+ ", " + text1 + ", " + text2;
}
}
let object_a = {
firstName:"DelftStack",
lastName: "Technology",
}
let x = p.combinedText.apply(object_a, ["Is", "Best"]);
document.getElementById("mypara").innerHTML = x;
}
</script>
In above example, x = object_b.combinedText.apply()
method is used with parameters (object_a,[array_arguments])
.
In the next step, we need to append the result variable in paragraph id mypara
to display the value on the HTML page.