How to Replace Space With %20 in Java
-
Use the
replaceAll()
Method to Replace Space With%20
in Java -
Use the
StringBuilder
Class to Replace Space With%20
-
Use the
String Tokenizer
WithStringBuilder
Method to Replace Space With%20
-
Use the
split
andjoin()
Methods to Replace Space With%20
-
Use the
java.net.URLEncoder
Method to Replace Space With%20
in Java - Conclusion
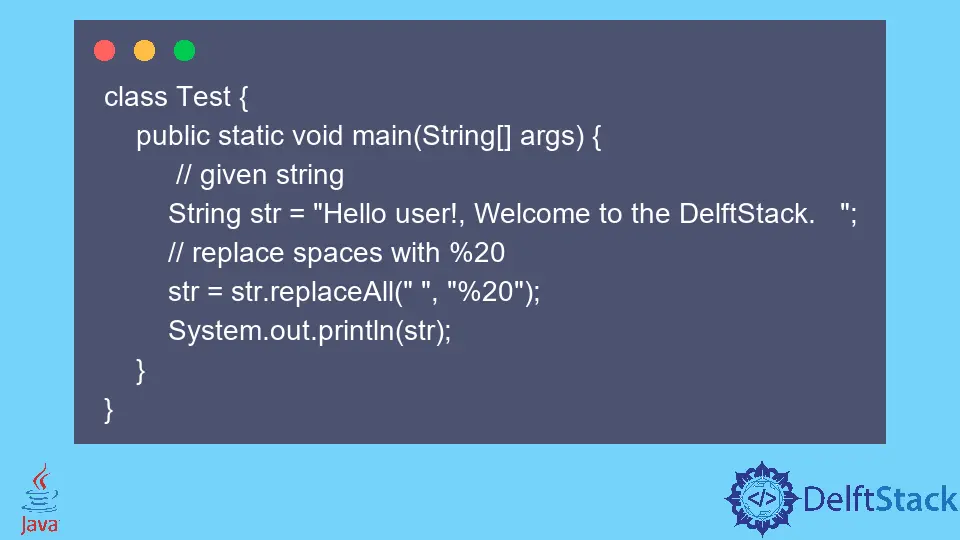
In this tutorial, we’ll explore various methods in Java for replacing spaces with %20
, including replaceAll()
, StringBuilder
, StringTokenizer
with StringBuilder
, split
() and join()
, and java.net.URLEncoder
. Whether you’re seeking simplicity, efficiency, or standardized URL encoding, this guide will provide concise insights into each method, helping you choose the approach that best suits your specific programming needs.
In the realm of string manipulation, the importance of replacing spaces with %20
cannot be overstated, particularly in the context of web development. Ensuring that spaces are encoded as %20
is crucial for creating valid and functional URLs.
This transformation plays a vital role in preventing issues related to URL parsing, enabling seamless communication between clients and servers. Whether constructing query parameters, forming file names, or managing dynamic content, the consistent replacement of spaces with %20
is an essential practice to maintain the integrity and effectiveness of web applications.
In this tutorial, we’ll delve into various methods in Java to achieve this transformation, each offering unique advantages for different programming scenarios.
Use the replaceAll()
Method to Replace Space With %20
in Java
Using replaceAll()
is advantageous for replacing spaces with %20
in Java because it allows for a direct and concise approach.
It simplifies code, enhancing readability, and is well-suited for straightforward string transformations.
Other methods, while effective, may involve additional complexity or be more tailored to specific scenarios, making replaceAll()
a versatile and preferred choice for a common and simple task like space replacement.
The replaceAll()
method in Java is used to replace all occurrences of a specified substring with another substring within a given string. In the context of replacing spaces with %20
, the syntax is as follows:
String replacedString = originalString.replaceAll(" ", "%20");
In the context of the replaceAll()
method in Java, the originalString
serves as the input string containing spaces.
This method is invoked with two parameters: the first being the regular expression to search for, represented by the space character " "
, and the second being the replacement string, denoted as "%20"
. The regular expression identifies all occurrences of spaces within the originalString
, and each space is replaced with %20
, resulting in a new string stored in the variable replacedString
.
The method returns a new string (replacedString
) with all spaces replaced by %20
.
Example:
public class SpaceReplacementExample {
public static void main(String[] args) {
// Original string with spaces
String originalString = "Hello World, Java Programming";
// Replace spaces with %20 using replaceAll() method
String replacedString = originalString.replaceAll(" ", "%20");
// Display the result
System.out.println("Original String: " + originalString);
System.out.println("Replaced String: " + replacedString);
}
}
We begin by declaring a class named SpaceReplacementExample
. This class encapsulates our main method and the logic for replacing spaces.
The main
method is the starting point of our program. It serves as the entry point where our space replacement logic will be implemented.
We initialize a String
variable, originalString
, with a sample string containing spaces.
The core of our operation is the replaceAll()
method.
Invoked on originalString
, it takes two parameters: a regular expression to search for (here, a space " "
) and the replacement string ("%20"
). This method replaces all space occurrences with %20
, and the modified string is stored in the variable replacedString
.
Finally, we print both the original and the replaced strings to the console. This allows us to observe the transformation achieved through the replaceAll()
method.
Output:
Original String: Hello World, Java Programming
Replaced String: Hello%20World,%20Java%20Programming
As demonstrated, the code successfully replaces spaces with %20
, showcasing the effectiveness and simplicity of the replaceAll()
method in Java string manipulation.
The replaceAll()
method in Java provides a convenient and concise way to replace all occurrences of a specified character or sequence within a string. In our case, it efficiently transforms spaces into %20
, making it particularly useful for tasks involving URL encoding or preparing data for web-related operations.
Use the StringBuilder
Class to Replace Space With %20
Using StringBuilder
to replace spaces with %20
in Java is beneficial for efficiency.
Unlike concatenation, StringBuilder
is mutable, reducing memory overhead. It’s ideal for large strings and enhances performance by modifying the content in place.
This method is concise and ensures optimal memory utilization, making it a preferred choice for dynamic string manipulation.
Example:
public class SpaceReplacementExample {
public static void main(String[] args) {
// Original string with spaces
String originalString = "Hello World, Java Programming";
// Replace spaces with %20 using StringBuilder
StringBuilder stringBuilder = new StringBuilder(originalString);
for (int i = 0; i < stringBuilder.length(); i++) {
if (stringBuilder.charAt(i) == ' ') {
stringBuilder.replace(i, i + 1, "%20");
}
}
String replacedString = stringBuilder.toString();
// Display the result
System.out.println("Original String: " + originalString);
System.out.println("Replaced String: " + replacedString);
}
}
We begin by declaring a public class, SpaceReplacementExample
, which will contain our main method and the logic for replacing spaces.
The main
method is where our program starts execution. It serves as the entry point, implementing our space replacement logic.
We initialize a String
variable, originalString
, with a sample string containing spaces.
A StringBuilder
is created and initialized with the content of originalString
. This mutable character sequence efficiently facilitates string modification.
We use a for
loop to iterate through each character of the StringBuilder
.
If a space is encountered, the replace
method is employed to substitute it with %20
. This dynamic replacement ensures the efficiency of the StringBuilder
for such tasks.
After space replacement, we convert the StringBuilder
back to a regular String
using the toString
method, and the result is stored in replacedString
.
Finally, we print both the original and replaced strings to the console, allowing us to observe the successful transformation achieved through the StringBuilder
method.
Output:
Original String: Hello World, Java Programming
Replaced String: Hello%20World,%20Java%20Programming
As demonstrated, the StringBuilder
method efficiently replaces spaces with %20
, providing a flexible and performance-conscious solution for string manipulation in Java.
Using StringBuilder
for string manipulation, especially in scenarios involving the replacement of characters, offers efficiency and mutability. In our case, the code successfully replaces spaces with %20
, showcasing the effectiveness of StringBuilder
in such string transformation tasks.
Use the String Tokenizer
With StringBuilder
Method to Replace Space With %20
Using StringTokenizer
in Java for replacing spaces with %20
is advantageous for simplicity. It efficiently breaks the string into tokens, allowing seamless manipulation.
This method is straightforward, especially for basic tokenization tasks, making it a concise choice for replacing spaces without the complexity of regular expressions or additional array processing.
Example:
import java.util.StringTokenizer;
public class SpaceReplacementExample {
public static void main(String[] args) {
// Original string with spaces
String originalString = "Hello World, Java Programming";
// Replace spaces with %20 using StringTokenizer
StringTokenizer tokenizer = new StringTokenizer(originalString, " ");
StringBuilder stringBuilder = new StringBuilder();
while (tokenizer.hasMoreTokens()) {
stringBuilder.append(tokenizer.nextToken());
if (tokenizer.hasMoreTokens()) {
stringBuilder.append("%20");
}
}
// Display the result
String replacedString = stringBuilder.toString();
System.out.println("Original String: " + originalString);
System.out.println("Replaced String: " + replacedString);
}
}
We start by importing the StringTokenizer
class from the java.util
package, a crucial step for utilizing this specific string manipulation approach.
Our journey begins with the declaration of a public class named SpaceReplacementExample
. This class encapsulates our main method and the logic for replacing spaces.
The main
method serves as the program’s entry point, where we implement our space replacement logic.
We initialize a String
variable, originalString
, with a sample string containing spaces.
A StringTokenizer
is created, taking originalString
and the space character as delimiters. This class efficiently breaks the string into tokens based on specified delimiters.
We enter a while
loop that iterates through the tokens obtained by the StringTokenizer
. For each token, we append it to a StringBuilder
. If there are more tokens (indicating the presence of spaces), we append %20
to the StringBuilder
.
After space replacement, we convert the StringBuilder
back to a regular String
using the toString
method, and the result is stored in replacedString
.
Finally, we print both the original and replaced strings to the console, allowing us to observe the successful transformation achieved through the StringTokenizer
method.
Output:
Original String: Hello World, Java Programming
Replaced String: Hello%20World,%20Java%20Programming
As demonstrated, the StringTokenizer
method efficiently replaces spaces with %20
, offering an alternative solution for string manipulation in Java.
The StringTokenizer
method, although considered somewhat old-fashioned in modern Java, remains a viable option for specific string manipulation tasks. In our case, the code successfully replaces spaces with %20
, showcasing the utility of StringTokenizer
for token-based string transformations.
Use the split
and join()
Methods to Replace Space With %20
Using split
and join
in Java for replacing spaces with %20
is advantageous for simplicity and readability. The combination offers a concise and modern approach, making the code clear and easy to understand.
In Java, the split()
and join()
methods are used for string manipulation.
The split()
method is a member of the String
class and is used to split a string into an array of substrings based on a specified delimiter. Here’s the syntax:
String[] split(String regex)
In Java, the split()
method is used to break a string into an array of substrings based on a specified delimiter. The regex
parameter in the split()
method represents the regular expression that serves as the delimiter.
This allows for flexible and customizable string splitting based on patterns defined by the regular expression. In essence, split()
provides a straightforward means to divide a string into components according to a specified pattern.
The join()
method is part of the String
class in Java and is used to concatenate strings. It was introduced in Java 8.
Here’s the syntax:
static String join(CharSequence delimiter, CharSequence... elements)
In Java, the join()
method facilitates the concatenation of elements into a single string. The delimiter
parameter specifies the character or sequence of characters to be placed between the joined elements, while the elements
parameter represents the array of elements to be combined.
Essentially, join()
provides a convenient way to construct a string by joining multiple elements with a specified delimiter.
It minimizes complexity compared to regular expressions or iterative methods, providing an efficient and straightforward solution for the common task of space replacement in strings.
Example:
public class SpaceReplacementExample {
public static void main(String[] args) {
// Original string with spaces
String originalString = "Hello World, Java Programming";
// Replace spaces with %20 using split and join
String[] words = originalString.split(" ");
String replacedString = String.join("%20", words);
// Display the result
System.out.println("Original String: " + originalString);
System.out.println("Replaced String: " + replacedString);
}
}
We declare a public class, SpaceReplacementExample
, to encapsulate our main method and the logic for replacing spaces.
The main
method is the starting point of our program, where we implement the space replacement logic. We initialize a String
variable, originalString
, with a sample string containing spaces.
The split
method is invoked on originalString
, breaking it into an array of substrings using the space character as the delimiter. This creates the words
array, where each word is an element.
The join
method is then applied, combining the elements of the words
array with %20
as the delimiter. This results in a single string with spaces replaced by %20
.
Finally, we print both the original and replaced strings to the console, allowing us to observe the successful transformation achieved through the straightforward split
and join
methods.
Output:
Original String: Hello World, Java Programming
Replaced String: Hello%20World,%20Java%20Programming
As demonstrated, the split
and join
methods offer an elegant and concise way to replace spaces with %20
in Java, making string manipulation more readable and efficient.
The combination of the split
and join
methods provides a concise and readable solution for string manipulation in Java. In our case, the code successfully replaces spaces with %20
, showcasing the effectiveness and simplicity of this modern approach.
Use the java.net.URLEncoder
Method to Replace Space With %20
in Java
Using java.net.URLEncoder
is crucial for URL encoding in Java, ensuring standardized encoding of special characters.
It simplifies the process, adheres to URL encoding standards, and handles spaces and other characters in a way that is compatible with web protocols.
This method is especially important for constructing valid URLs in web development, preventing issues related to spaces and other reserved characters that might disrupt proper communication between clients and servers.
Example:
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.nio.charset.StandardCharsets;
public class URLEncoderExample {
public static void main(String[] args) {
// Original string with spaces
String originalString = "Hello World, Java Programming";
// Replace spaces with %20 using java.net.URLEncoder
String encodedString = encodeSpaces(originalString);
// Display the result
System.out.println("Original String: " + originalString);
System.out.println("Encoded String: " + encodedString);
}
private static String encodeSpaces(String input) {
try {
return URLEncoder.encode(input, StandardCharsets.UTF_8.toString()).replace("+", "%20");
} catch (UnsupportedEncodingException e) {
// Handle encoding exception
e.printStackTrace();
return input; // Return the original string in case of an exception
}
}
}
We begin by importing necessary classes from the java.net
package, setting the stage for URL encoding.
The main
method serves as our program’s entry point, where we implement URL encoding logic. We initialize a String
variable, originalString
, with a sample string containing spaces.
A method named encodeSpaces
is called, passing originalString
as an argument. This method handles the URL encoding process.
Within the encodeSpaces
method, the input string is attempted to be encoded using UTF-8. The replace
method then substitutes any +
character (used for space in URL encoding) with %20
.
Encoding exceptions are caught, and the stack trace is printed.
Finally, we print both the original and encoded strings to the console, allowing us to observe the successful transformation achieved through the java.net.URLEncoder
class.
Output:
Original String: Hello World, Java Programming
Encoded String: Hello%20World,%20Java%20Programming
As demonstrated, the java.net.URLEncoder
class simplifies the process of URL encoding, making it a robust choice for handling special characters in URL strings.
The java.net.URLEncoder
class provides a standardized and reliable solution for URL encoding in Java. In our case, the code successfully replaces spaces with %20
, showcasing the effectiveness of this library implementation for URL encoding tasks.
Conclusion
In conclusion, the choice of method for replacing spaces with %20
in Java depends on the specific requirements and preferences of the developer.
replaceAll()
stands out for its simplicity and directness, making it suitable for uncomplicated string transformations, especially when regular expressions are unnecessary. StringBuilder
excels in efficiency and mutability, proving optimal for large strings and versatile for dynamic string manipulation.
StringTokenizer
combined with StringBuilder
provides a traditional yet effective approach, particularly useful when breaking a string into tokens is a primary requirement. The modern and concise approach of split
and join
enhances code clarity, which is ideal for straightforward tasks like space replacement.
Lastly, java.net.URLEncoder
ensures standardized URL encoding, simplifying the process and proving valuable for handling special characters within URLs and adhering to encoding standards.
Ultimately, the selection depends on factors such as code simplicity, performance considerations, and adherence to specific standards, allowing developers to tailor their choice based on the context of their application.