How to Validate Password in Java
Waleed
Feb 02, 2024
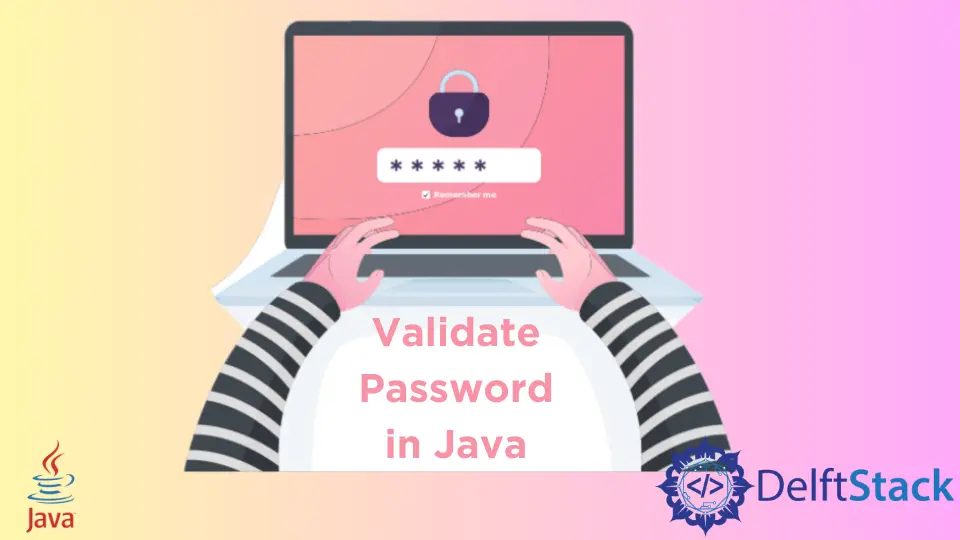
While creating a login page, we often have to validate the password and check whether it follows the proper standard or not.
Validating password included checking the password whether it is correct or not according to the password policy discussed below:
- A standard password contains at least eight characters
- A regular password contains at least includes two uppercase letters
- A standard password contains two lowercase letters
- A standard password includes two special symbols
Algorithm to Validate Password in Java
- Before checking our password, we must specify how many uppercase, lowercase, special case letters, or digits an ideal password should contain. For this purpose, we define the variable MAX, MIN_Uppercase, MIN_Lowercase, Num_Digits, and Special of type int.
- To count how many uppercase, lowercase, digits, or special characters our password has, we’ll declare variables uppercaseCounter, lowercaseCounter, special, and digitCounter of type integer.
- After declaring the counter variables, we initialize them by 0. By initializing the counter variable by 0, we ensure they don’t contain any garbage value.
- We’ll first ask the user to enter a password to validate the given password. To take user input, we first import the scanner class in our program and then create an object. In our program object of the scanner, class is input.
- The scanner function has a default function of
.nextLine()
that reads user input and then store that in a string. - We then iterate all the password characters and store each character in a
c
variable of type char. - The character data type has a default function
isUpperCase
,isLowerCase
, andisDigit
. Using these default functions, we calculate how many uppercase and lowercase characters, special symbols, or numbers our password contains. - If a character stored in the c variable is uppercase, we increment the uppercaseCount variable by 1.
- If a character stored in a c variable is lowercase, we increment the lowercaseCounter variable by 1.
- If a character stored in c is a number, we increment the
digitCounter
variable by 1. - If a character stored in c is a special symbol, we increment a special counter by 1.
- After doing that, we run the conditional statement to check whether our password contains the specified characters like
@#%
, lowercase and uppercase letters, and digits or not. - If the password meets our specified criteria, we’ll print the
Validate password
statement. - In case the password doesn’t meet all or some of our conditions, we first display the statement,
Your password doesn't contain the following
. - Lastly, we’ll check what the specified condition(s) password are doesn’t meet and then display the message accordingly.
package sampleProject;
import java.util.*;
import java.util.Scanner;
public class Codesample {
public static void main(String[] args) {
// Specify the maximum number of letters in a password
final int MAX = 8;
// Specifying the number of uppercase letters in password
final int MIN_Uppercase = 2;
// Specifying the minimum lowercase letters in password
final int MIN_Lowercase = 2;
// Specifying the number of digits in a password
final int NUM_Digits = 2;
// Specify the minimum number of special case letters
final int Special = 2;
// Count number of uppercase letters in a password
int uppercaseCounter = 0;
// Counter lowercase letters in a password
int lowercaseCounter = 0;
// Count digits in a password
int digitCounter = 0;
// count special case letters in a password
int specialCounter = 0;
// Take input of password from the user
System.out.println("Enter the password\n");
Scanner input = new Scanner(System.in);
// Take password input from the user and save it in the string password
String password = input.nextLine();
for (int i = 0; i < password.length(); i++) {
char c = password.charAt(i);
if (Character.isUpperCase(c))
uppercaseCounter++;
else if (Character.isLowerCase(c))
lowercaseCounter++;
else if (Character.isDigit(c))
digitCounter++;
if (c >= 33 && c <= 46 || c == 64) {
specialCounter++;
}
}
if (password.length() >= MAX && uppercaseCounter >= MIN_Uppercase
&& lowercaseCounter >= MIN_Lowercase && digitCounter >= NUM_Digits
&& specialCounter >= Special) {
System.out.println("Valid Password");
} else {
System.out.println("Your password does not contain the following:");
if (password.length() < MAX)
System.out.println(" atleast 8 characters");
if (lowercaseCounter < MIN_Lowercase)
System.out.println("Minimum lowercase letters");
if (uppercaseCounter < MIN_Uppercase)
System.out.println("Minimum uppercase letters");
if (digitCounter < NUM_Digits)
System.out.println("Minimum number of numeric digits");
if (specialCounter < Special)
System.out.println("Password should contain at lest 3 special characters");
}
}
}
Output:
Enter the password
abcd1234
Your password does not contain the following:
At least 8 characters
Minimum lowercase letters
Minimum, uppercase letters
Minimum number of numeric digits
Password should contain at least 3 special characters
Enter the password
DElft@#90
Valid Password