MySQL Connector Java Maven Dependency
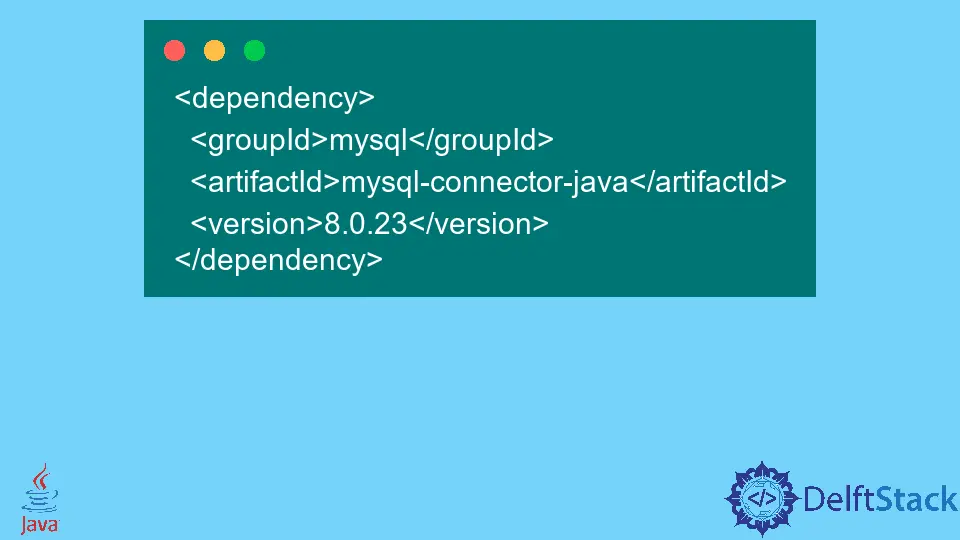
MySQL Connector Java is a Java driver that allows you to connect to a MySQL database from your Java application. This driver is an open-source, pure Java implementation of the MySQL protocol, and it provides a Java API for accessing and manipulating a MySQL database.
You need to obtain the Maven dependency to use MySQL Connector Java in your Java application. This article will discuss obtaining the MySQL Connector Java Maven dependency.
Steps to Use MySQL Connector Java Maven Dependency
-
Check for the Latest Version
Before starting, you need to check the latest version of the MySQL Connector Java library. You can check this by visiting the MySQL Connector Java download page on the MySQL website.
Once you have the latest version, you can proceed to the next step.
-
Add the Dependency to Your POM File
To obtain the MySQL Connector Java Maven dependency, you must add it to your project’s
pom.xml
file. Thepom.xml
file is the main configuration file for a Maven project, containing information about the project and its dependencies.To add the MySQL Connector Java dependency, you must add the following code to the
pom.xml
file.<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>8.0.23</version> </dependency>
Note that you should replace the version number in the code with the latest version of the MySQL Connector Java library you checked in step 1.
-
Update the Project
Once you have added the MySQL Connector Java dependency to your
pom.xml
file, you need to update your project to download the library. You can do this by running the following command in the terminal.mvn clean install
This command will download the MySQL Connector Java library and all its dependencies and add them to your project’s
classpath
. Once the command has finished, you can use the MySQL Connector Java library in your Java application. -
Import the Library in Your Java Code
Once you have obtained the MySQL Connector Java Maven dependency, you need to import it into your Java code to use it. You can add the following code at the top of your Java file.
import java.sql.DriverManager;
-
Connect to the MySQL Database
Finally, you can use the MySQL Connector Java library to connect to your database. To do this, you need to create a connection object and set the properties for the connection, such as the URL, username, and password.
You can do this by using the following code:
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/database", "username", "password");
In the code, you must replace
"localhost:3306/database"
with the URL of your MySQL database,"username"
with the username, and"password"
with the password.Once you have created the connection object, you can execute SQL statements and retrieve data from the database.
Note: This code cannot be run on any online compiler.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook