How to Mock Objects in Java
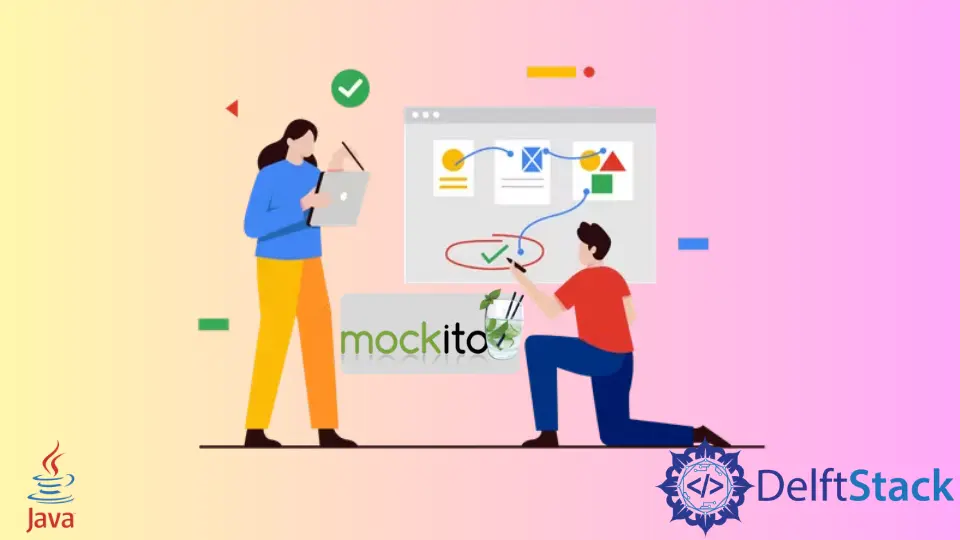
Mock objects help simulate and verify real objects without running the real code in those objects. You can set up a mock to return specific results on the method calls and easily verify whether the method was called or not.
As discussed above, the Mock objects are used for unit testing. If you have an object whose methods you want to test and those methods are dependent on some other objects, then, in that case, you can create a mock of the dependency rather than the actual instance of that dependency, and you can test your objects in isolation.
Mock Objects in Java
The common Java frameworks for mock object creation include JMock and EasyMock. These two frameworks generally allow you to create mock objects, and you can define their behavior accordingly to know what exactly to expect from the return values and the effect.
For instance, one common use case might be in a Model-View-Controller (MVC) application where we have a Data Access Objects (DAO) layer and a controller that performs the business logic. If you want to test the controller and the controller has a dependency on a Data Access Object (DAO), then you can make a mock object of the DAO that will return dummy objects to your controller.
Mock objects help in testing the functionality of an application that you want to test because we can achieve all the dependencies by mocking those dependent objects.
And if they pass the test, it’s sure that the unit under this test is correct.
One important thing to consider is that mock objects implement the same interface as the real objects they are mocking. This allows your code to deal with them via the interface type as if they are instances of the real thing.
To use mock objects, import the Mockito
library; import static org.mockito.Mockito.*;
allows you to use the mock()
method, which helps create mock object instances.
Next step is the mock creation and the syntax is List mockedList = mock(List.class);
. Now to add some values we use the the defined object of List
as follows, mockedList.add("Value one")
, and verify it using the verify()
method as follow, verify(mockedList).add("one")
.
// Import Library
import static org.mockito.Mockito.*;
// Create Mock
List mockedList = mock(List.class);
// using mock object
mockedList.add("one");
mockedList.clear();
// Varifying
verify(mockedList).add("one");
verify(mockedList).clear();
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn