How to Convert Milliseconds to Minutes and Seconds in Java
- Importance of Converting Milliseconds to Minutes and Seconds
- Converting Milliseconds to Minutes and Seconds Using Division and Modulus Method in Java
-
Converting Milliseconds to Minutes and Seconds Using
TimeUnit
in Java -
Converting Milliseconds to Minutes and Seconds Using
Duration
in Java - Best Practices for Converting Milliseconds to Minutes and Seconds
- Conclusion
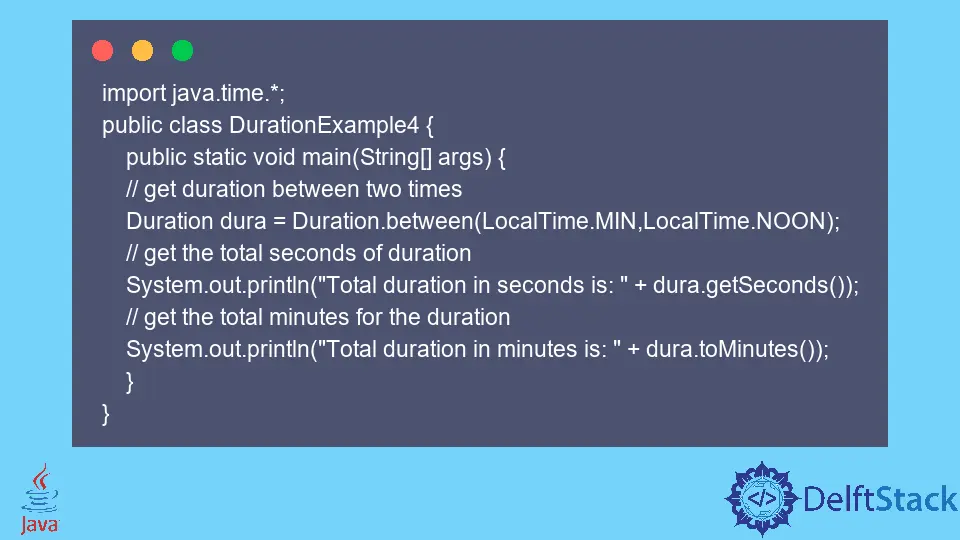
In this article, we delve into the significance of converting milliseconds to minutes and seconds, exploring the importance of this operation in Java programming and providing practical insights into various methods to achieve this conversion seamlessly.
Importance of Converting Milliseconds to Minutes and Seconds
Converting milliseconds to minutes and seconds is crucial for handling time-related data in Java applications. It transforms raw time values into a human-readable format, facilitating efficient representation and manipulation of temporal information.
This conversion is essential for various functionalities, including timers, scheduling, and any application requiring precise time calculations, enhancing overall code clarity and user understanding.
Converting Milliseconds to Minutes and Seconds Using Division and Modulus Method in Java
The division and modulus method is crucial for converting milliseconds to minutes and seconds as it provides a simple and efficient mathematical approach. By dividing milliseconds by the appropriate constants (1000 and 60), it extracts the minute and second components.
The modulus operation ensures accurate calculation, preventing potential errors and simplifying the conversion process in a concise manner.
Code Example:
public class MillisecondsConverter {
public static void main(String[] args) {
long milliseconds = 150000; // Example milliseconds
// Convert milliseconds to minutes and seconds
long minutes = (milliseconds / (1000 * 60)) % 60;
long seconds = (milliseconds / 1000) % 60;
// Display the result
System.out.println("Minutes: " + minutes);
System.out.println("Seconds: " + seconds);
}
}
In this example, we begin by initializing a variable milliseconds
with a value of 150,000 milliseconds, serving as the input for our conversion. Moving into the core of the conversion process, we employ a two-step method.
Initially, milliseconds are converted to minutes by dividing by (1000 * 60)
, where 1000 signifies the number of milliseconds in a second, and 60 represents the seconds in a minute. The modulus operation % 60
is applied to obtain the remaining minutes after the conversion.
Similarly, the remaining milliseconds are converted to seconds by dividing by 1000, with the modulus operation % 60
ensuring the retrieval of the remaining seconds post-conversion.
Finally, to provide a clear visualization of the converted values, we use System.out.println
to display the minutes and seconds. This step concludes the process, offering a concise and informative representation of the time conversion.
If you run this program with the provided example, the output would be:
The division and modulus method for converting milliseconds to minutes and seconds in Java is a concise and effective approach. By breaking down the conversion process into simple mathematical operations, this method provides a clear and readable solution for handling time-related calculations in your Java applications.
Whether you are a beginner or an experienced Java developer, incorporating this method into your toolkit can significantly enhance your ability to work with time-related data in a more human-friendly format.
Converting Milliseconds to Minutes and Seconds Using TimeUnit
in Java
The TimeUnit
method is essential for converting milliseconds to minutes and seconds, offering a high-level abstraction that enhances code readability. By providing dedicated methods like toMinutes()
and toSeconds()
, it simplifies time unit conversions, reducing the need for explicit mathematical operations.
This approach not only improves code clarity but also mitigates the risk of errors, making the conversion process more intuitive and accessible for developers.
Code Example:
import java.util.concurrent.TimeUnit;
public class MillisecondsConverter {
public static void main(String[] args) {
long milliseconds = 150000; // Example milliseconds
// Convert milliseconds to minutes and seconds using TimeUnit
long minutes = TimeUnit.MILLISECONDS.toMinutes(milliseconds);
long seconds =
TimeUnit.MILLISECONDS.toSeconds(milliseconds) - TimeUnit.MINUTES.toSeconds(minutes);
// Display the result
System.out.println("Minutes: " + minutes);
System.out.println("Seconds: " + seconds);
}
}
To utilize the TimeUnit
class, we begin by importing it, as this class offers various time-based utility methods for handling time durations between different units. Following that, we initialize a variable milliseconds
with a value of 150,000 milliseconds, serving as the input for our conversion.
Moving into the conversion process, the TimeUnit.MILLISECONDS.toMinutes(milliseconds)
method is employed to seamlessly convert milliseconds to minutes. Subsequently, the corresponding seconds are determined by subtracting the total seconds equivalent to the converted minutes, achieved through TimeUnit.MINUTES.toSeconds(minutes)
.
Finally, to provide a clear visualization of the converted values, we use System.out.println
to display the minutes and seconds on the console. This step concludes the process, delivering a simple and effective representation of the time conversion.
If you run this program with the provided example, the output would be:
Utilizing the TimeUnit
class in Java for converting milliseconds to minutes and seconds streamlines the process and enhances code readability. This approach is especially beneficial when you want to achieve concise and expressive time-related operations in your Java applications.
Whether you are a novice or an experienced developer, incorporating the TimeUnit
class into your toolkit ensures a clean and efficient solution for handling time conversions.
Converting Milliseconds to Minutes and Seconds Using Duration
in Java
The Duration
method is vital for converting milliseconds to minutes and seconds, especially in Java 8 and later versions. It encapsulates time durations in a clear and readable form, offering a modern and concise solution.
With methods like toMinutes()
and getSeconds()
, it simplifies the extraction of minutes and seconds, enhancing code readability and reducing complexity. This approach ensures a straightforward and effective conversion process, contributing to the overall clarity and maintainability of the code.
Code Example:
import java.time.Duration;
public class MillisecondsConverter {
public static void main(String[] args) {
long milliseconds = 150000; // Example milliseconds
// Convert milliseconds to Duration
Duration duration = Duration.ofMillis(milliseconds);
// Extract minutes and seconds from Duration
long minutes = duration.toMinutes();
long seconds = duration.minusMinutes(minutes).getSeconds();
// Display the result
System.out.println("Minutes: " + minutes);
System.out.println("Seconds: " + seconds);
}
}
To leverage the Duration
class, we begin by importing it, as this class, introduced in Java 8 and residing in the java.time
package, serves as a representation of a time duration. Moving to variable initialization, we set the value of milliseconds
to 150,000, which can be replaced with any desired millisecond value.
The heart of the conversion process lies in using the Duration.ofMillis(milliseconds)
method, effectively creating a Duration
object that encapsulates the time duration in a convenient and readable format. To extract minutes, the toMinutes()
method of the Duration
class is utilized, while for seconds, a two-step approach involves subtracting the minutes using minusMinutes(minutes)
and obtaining the remaining seconds with getSeconds()
.
Concluding the process, we print the converted values of minutes and seconds to the console using System.out.println
, offering a clear and concise visualization of the time conversion.
If you run this program with the provided example, the output would be:
The Duration
class in Java offers a modern and intuitive way to handle time-based calculations. In the context of converting milliseconds to minutes and seconds, this approach provides clarity and conciseness to your code.
Whether you are new to Java or an experienced developer, incorporating the Duration
class into your projects ensures a robust and effective solution for managing time-related operations.
Best Practices for Converting Milliseconds to Minutes and Seconds
Choose Appropriate Method
Select the conversion method based on your Java version and project requirements, whether it’s the division and modulus approach, the TimeUnit
class, or the Duration
class.
Ensure Input Validation
Before conversion, validate the input milliseconds to ensure non-negative values and handle edge cases to prevent unexpected results.
Use Constants for Units
To enhance code readability and maintainability, use constants for time units, such as milliseconds, seconds, and minutes, rather than hardcoding numerical values.
Consider Java Version
Take into account the Java version in use, opting for modern approaches like TimeUnit
and Duration
in Java 8 and later for improved functionality and readability.
Document Your Code
Include concise comments or documentation to explain the chosen conversion method and any specific considerations for future reference or collaboration.
Conclusion
Mastering the conversion of milliseconds to minutes and seconds in Java not only proves crucial for various time-sensitive applications but also highlights the significance of utilizing efficient methods, such as division and modulus, TimeUnit
, or Duration
, to ensure code clarity, simplicity, and accuracy in time-related calculations.