Annotation Symbol @ in Java
-
the
@Override
Annotation in Java -
the
@SuppressWarnings
Annotation in Java -
the
@Deprecated
Annotation in Java
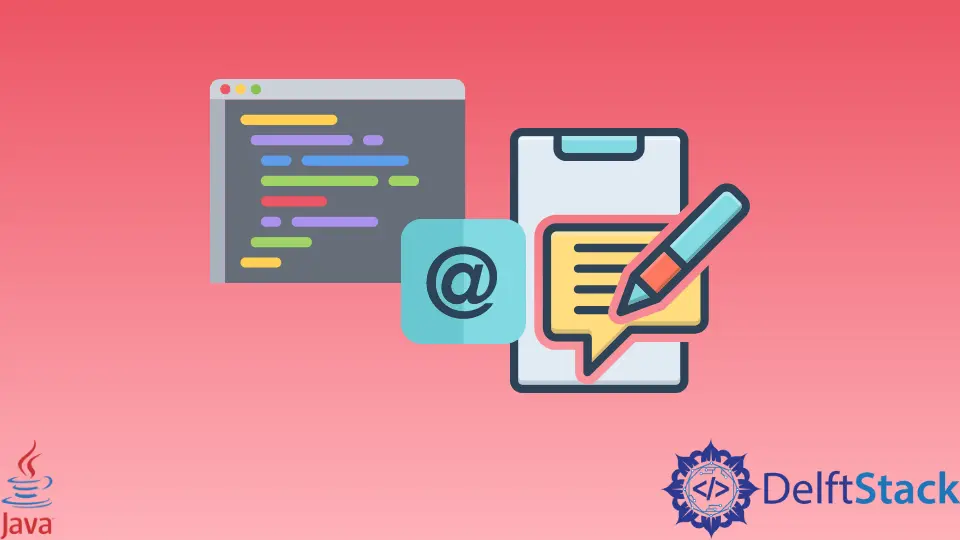
This article will introduce the @
symbol, which is called annotations in Java. We will focus on built-in java annotations like @Override
, @SuppressWarnings
, and @Deprecated
.
the @Override
Annotation in Java
Annotation is a way to indicate the extra information attached with a class, interface, and method in Java. The Java compiler and the JVM use the provided information with the use of annotations. An annotation starts with the @
symbol. The @Override
annotation ensures that the child class method overrides the parent class method. It is a marker annotation because it does not have any values or elements. When we use the @Override
annotation, the compiler will generate errors and warn us if there is any mistake while overriding the method. The probable mistakes that it checks are the spelling mistake and the errors in argument and return type. We can demonstrate the use of the @Override
annotation by creating an inheritance. Firstly, we will not use the annotation, but later we will use it to demonstrate how @Override
works.
For example, create a class Parent
and write a method message()
with a void
return type. Print some messages inside the method. Similarly, create another class, Child
, and extend the class Parent
. Create the same method message
with the void
type and write a message inside it. Just before the method, write the annotation @Override
. Then, inside the main class, create objects of each of the classes and call the message()
method.
Here, the subclass inherits the superclass, and each of the methods in each class is invoked without any problem.
Example Code:
class Annotation {
public static void main(String[] args) {
new Child().message();
new Parent().message();
}
}
class Parent {
void message() {
System.out.println("Invoked from the Parent class");
}
}
class Child extends Parent {
@Override
void message() {
System.out.println("Invoked from the Child class");
}
}
Output:
Invoked from the Child class
Invoked from the Parent class
Modify the message()
method in the Child
class into mesage()
. Then, run the program. It shows the following output.
Output:
Annotation.java:18: error: method does not override or implement a method from a supertype
Here, we changed the method name. Sometimes, the programmer can commit such types of mistakes while overriding. Thus, the @Override
annotation ensures any error while overriding a method and alerts the programmer.
the @SuppressWarnings
Annotation in Java
The @SuppressWarnings
annotation tells the compiler to suppress or ignore the error for the specified piece of the code. We can use the annotation for a type, field, method, parameter, constructor, and local variable. We can specify the value unchecked
between the parentheses of the annotation as @SuppressWarnings("unchecked")
to suppress the error. When we use the annotation before the class, it will suppress any errors inside the class. And when we use it before any method inside a class, it will only suppress the error from that particular class.
For example, import the util
package and create a class named Annotation
. Write @SuppressWarnings("unchecked")
before the main method. Inside the main method, create an instance of the ArrayList()
and assign it to the fruits
variable. Then, add the values apple
and mango
with the add()
method. Finally, print the fruits
variable.
In the example below, we have imported everything from the util
package. It imports the ArrayList
class that we are using afterward. The code above successfully executes, although there is an error. We have used the non-generic collection ArrayList
. We have not specified the type inside a <>
symbol. Therefore, the code is faulty. But it executes because we have suppressed the error using the @SuppressWarnings
annotation.
Example code:
import java.util.*;
class Annotation {
@SuppressWarnings("unchecked")
public static void main(String args[]) {
ArrayList fruits = new ArrayList();
fruits.add("apple");
fruits.add("mango");
System.out.println(fruits);
}
}
Output:
[apple, mango]
If we don’t use the annotation for the following code, the compiler will show the following error.
Output:
Note
Annotation.java uses unchecked or unsafe operations.
the @Deprecated
Annotation in Java
The @Deprecated
annotation is used to denote the specified piece of code as deprecated code. The compiler will show a deprecation warning when we use the annotation. We can annotate a type, method, field, and constructor using the @Deprecated
annotation.
For example, create a class Car
and create a constructor with the parameters color
, length
, and width
. Use the @Deprecated
annotation before the constructor. Leave the body empty. Create another constructor and write a string parameter name
in it. Create another class, Annotation
, and inside the main method, call these two constructors.
In the example below, we have used the @Deprecated
annotations ahead of the first constructor. It enables the compiler to generate a warning saying that the constructor is deprecated. In this way, we can use the annotation to specify something that is deprecated in the code. Removing the deprecated code is not encouraged because it can be used to roll back to previous versions if needed. The new alternative is encouraged to be used in such cases.
Example Code:
class Annotation {
public static void main(String args[]) {
new Car("red", 550, 300);
new Car("Lambo");
}
}
class Car {
@Deprecated
Car(String color, int length, int width) {}
Car(String name) {}
}
Output:
Note
Annotation.java uses or overrides a deprecated API.