How to Run Java .Class Files From Command Line
- Prerequisites for Running Java .Class Files
- Compiling Java Code
- Running Java .Class Files
- Running Java .Class Files with Classpath
- Conclusion
- FAQ
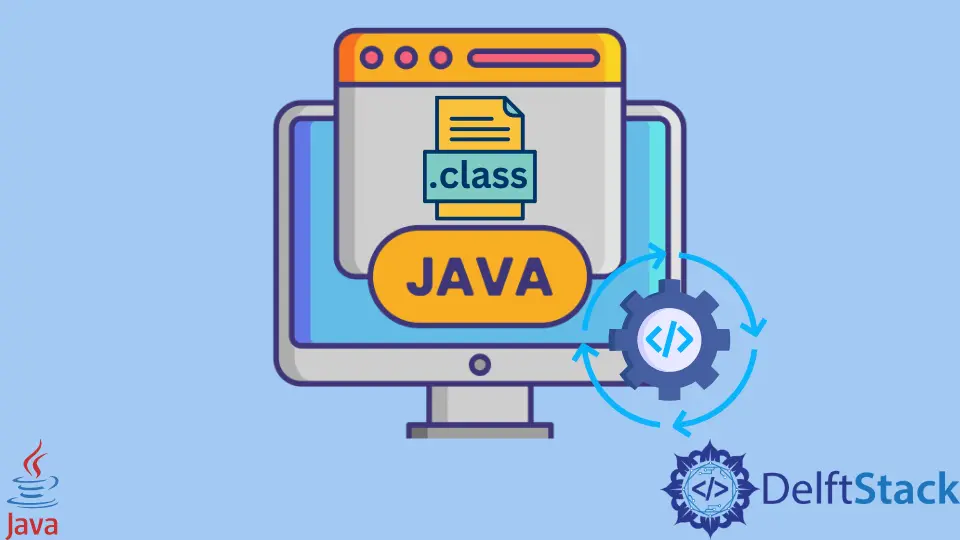
Executing Java .class files from the command line can seem daunting for beginners, but it’s a fundamental skill for any Java developer. Whether you’re debugging your code, running a simple program, or managing a larger project, knowing how to run .class files efficiently can save you time and frustration.
In this tutorial, we will guide you through the steps to run Java .class files directly from the command line. We’ll cover the necessary commands and provide clear examples to ensure you can execute your Java programs with ease. So, let’s dive in and unlock the power of Java right from your terminal!
Prerequisites for Running Java .Class Files
Before you start running Java .class files from the command line, there are a few prerequisites you need to have in place. First, ensure that you have Java Development Kit (JDK) installed on your system. You can verify this by running the command java -version
in your terminal. If it returns the version of Java installed, you are good to go. If not, you’ll need to download and install the JDK from the official Oracle website or your system’s package manager.
Next, make sure your environment variables are set correctly. For Windows users, the JAVA_HOME
variable should point to the JDK installation directory, and the Path
variable should include the bin
directory of your JDK. For macOS and Linux users, you can set these variables in your shell configuration file (like .bashrc
or .zshrc
). Once you have these prerequisites in place, you are ready to run your Java .class files.
Compiling Java Code
To run a Java .class file, you first need to compile your Java source code (.java files) into bytecode (.class files). This is done using the javac
command. Here’s a simple example to illustrate the process.
- Create a Java file named
HelloWorld.java
with the following content:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
-
Open your command line interface and navigate to the directory where your
HelloWorld.java
file is located. Use thecd
command to change directories. -
Compile the Java file using the following command:
javac HelloWorld.java
Output:
After running this command, you should see no output, which indicates that the compilation was successful. If there are any errors in your code, they will be displayed in the terminal.
The javac
command takes your Java source file and translates it into bytecode, creating a HelloWorld.class
file in the same directory. This .class file is what you will execute in the next step.
Running Java .Class Files
Now that you have compiled your Java code into a .class file, it’s time to run it. You can do this using the java
command followed by the name of the class (without the .class
extension). Here’s how you can run the compiled HelloWorld class.
- In the same directory where your
HelloWorld.class
file is located, execute the following command:
java HelloWorld
Output:
Hello, World!
When you run this command, the Java Virtual Machine (JVM) starts and looks for the main
method in the HelloWorld
class. It executes the code within that method, which prints “Hello, World!” to the console.
If you encounter an error stating that the main class could not be found, double-check that you are in the correct directory and that the class name is spelled correctly. Remember, the class name is case-sensitive.
Running Java .Class Files with Classpath
Sometimes, your Java classes may depend on other classes or libraries. In such cases, you might need to specify the classpath, which tells the JVM where to look for user-defined classes and packages. The -cp
or -classpath
option allows you to set this path.
Suppose you have a directory structure like this:
/project
/lib
helper.jar
HelloWorld.java
To compile and run HelloWorld.java
, which uses classes from helper.jar
, you can do the following:
- Compile the Java file:
javac -cp lib/helper.jar HelloWorld.java
Output:
- Run the Java class while specifying the classpath:
java -cp .:lib/helper.jar HelloWorld
Output:
Hello, World!
In this command, .
represents the current directory, and lib/helper.jar
is the path to the external library. The :
is used to separate multiple paths in Unix-based systems. On Windows, use a semicolon ;
instead.
By using the classpath, you can include external libraries and other classes, allowing your Java applications to leverage additional functionalities.
Conclusion
Running Java .class files from the command line is a straightforward process once you understand the basic commands. By compiling your Java source code with javac
and executing the compiled classes with java
, you can quickly test and run your Java applications. Remember to set your classpath correctly when dealing with external libraries. With these skills, you’ll be well on your way to becoming proficient in Java development. Keep practicing, and soon you’ll be running complex Java applications with ease!
FAQ
-
How do I check if Java is installed on my system?
You can check if Java is installed by running the commandjava -version
in your command line. -
What should I do if I receive a “class not found” error?
Ensure you are in the correct directory and that the class name is spelled correctly, including proper case sensitivity. -
Can I run Java .class files without compiling them first?
No, you must compile .java files into .class files before running them. -
How can I set the JAVA_HOME environment variable?
For Windows, go to System Properties > Environment Variables. For macOS/Linux, you can set it in your shell configuration file.
- What is the purpose of the classpath in Java?
The classpath tells the JVM where to find user-defined classes and packages, especially when using external libraries.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook