Multiset Data Structure in Java
- Options to Implement Multiset in Java
- Example of Multiset Data Structure in Java
- Convenient Implementation of Multiset in Java
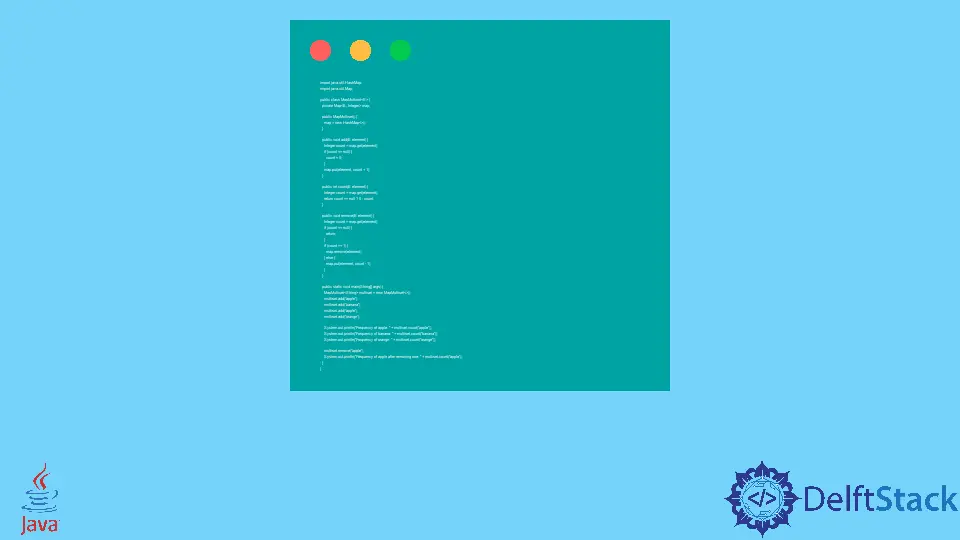
Multisets are data structures that allow you to store multiple elements of the same value. In C++, the Standard Template Library (STL) includes a multiset implementation that provides convenient operations for inserting, removing, and searching for elements.
This data structure is useful in various applications, such as when you need to count the frequency of elements in a collection.
But does Java have a multiset data structure like the one in C++ STL? The short answer is yes.
Options to Implement Multiset in Java
Java provides several options for implementing a multiset, including:
- Using a
Map
: AMap
is a collection that maps keys to values, and one option for implementing a multiset is to use aMap
where the keys are the elements, and the values are their frequencies. To insert an element, you can increment its frequency, and to remove an element, you can decrement its frequency. - Using a
List
or anArray
: Another option is to use aList
or anArray
to store the elements and then use a loop to count the frequency of each element. This approach can be slow when the collection size is large, as it requires linear time to find the frequency of an element. - Using a third-party library: Several third-party libraries are available that provide a multiset implementation for Java, such as Google’s Guava library and Apache Commons Collections. These libraries provide a convenient implementation similar to the C++ STL multiset and can be a good option if you want to avoid writing your implementation.
So, Java does have a multiset data structure like the one in C++ STL, but the implementation is not part of the core Java libraries. Instead, you must use a Map
, a List
or an Array
, or a third-party library to implement a multiset in Java.
Example of Multiset Data Structure in Java
Here is a simple example of a multiset data structure in Java using a Map<E, Integer>
:
import java.util.HashMap;
import java.util.Map;
public class MapMultiset<E> {
private Map<E, Integer> map;
public MapMultiset() {
map = new HashMap<>();
}
public void add(E element) {
Integer count = map.get(element);
if (count == null) {
count = 0;
}
map.put(element, count + 1);
}
public int count(E element) {
Integer count = map.get(element);
return count == null ? 0 : count;
}
public void remove(E element) {
Integer count = map.get(element);
if (count == null) {
return;
}
if (count == 1) {
map.remove(element);
} else {
map.put(element, count - 1);
}
}
public static void main(String[] args) {
MapMultiset<String> multiset = new MapMultiset<>();
multiset.add("apple");
multiset.add("banana");
multiset.add("apple");
multiset.add("orange");
System.out.println("Frequency of apple: " + multiset.count("apple"));
System.out.println("Frequency of banana: " + multiset.count("banana"));
System.out.println("Frequency of orange: " + multiset.count("orange"));
multiset.remove("apple");
System.out.println("Frequency of apple after removing one: " + multiset.count("apple"));
}
}
In this example, we use a Map<E, Integer>
to implement a multiset—the map stores elements as keys and their frequencies as values.
We have methods add
, count
, and remove
that allow us to add an element to the multiset, find the frequency of an element, and remove one occurrence of an element, respectively.
The add
method retrieves the current count of the element from the map, and if it is null
, sets it to 0
. The method then adds 1
to the count and stores the updated count on the map.
The count
method retrieves the count of the element from the map and returns it or returns 0
if the element is not in the map.
The remove
method retrieves the count of the element from the map, and if it is null
, returns immediately. If the count is 1
, the method removes the element from the map.
The method decrements and stores the updated count in the map if the count is greater than 1
.
When the code is executed, it adds several elements to the multiset, finds the frequency of each element, removes one occurrence of the "apple"
element, and prints the frequency of each element.
Click here to check the code.
Convenient Implementation of Multiset in Java
Suppose you are looking for a convenient and efficient multiset implementation in Java. Using a third-party library like Guava or Apache Commons Collections is a good idea.
These libraries provide a rich set of functionality, including various operations for inserting, removing, and searching for elements and other utility methods.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook