How to Create a Number Guessing Game in Java
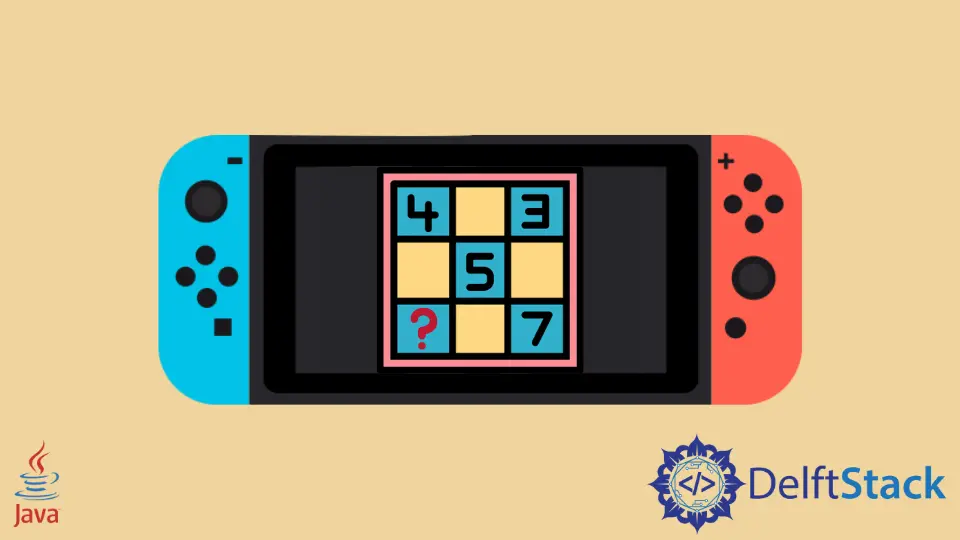
Whether played solo or with friends, the number guessing game provides a delightful way to exercise one’s mind and revel in the joy of a well-guessed number. But for the developer’s end, it is not that simple.
Number guessing games captivate players with a blend of challenge and excitement. The allure lies in the simplicity and suspense of deducing a randomly generated number.
As players take strategic guesses, the game fosters an engaging environment, providing a quick mental challenge and instant gratification upon success. It’s a timeless and accessible activity, appealing to people of all ages and backgrounds, offering a brief yet rewarding escape into a world of numerical possibilities.
Create a Number Guessing Game in Java
A Java developer creating a number guessing game should consider defining clear game rules, implementing user-friendly input handling, and incorporating the Random
class for unpredictability.
Balancing simplicity and engagement, setting reasonable difficulty levels, and optimizing code for readability and maintainability are essential. Additionally, incorporating error handling ensures a robust user experience.
Guessing Game Rules
In the Number Guessing Game, players are invited to predict a randomly generated number within a specified range. The game is initiated with a welcoming message and a randomly chosen number between 1 and 100.
Players can input their guesses, receiving feedback on whether the correct number is higher or lower than their estimate. In order to maintain engagement, the game limits players to a maximum of 10 attempts.
Importantly, players have the option to exit the game at any point by entering 0.
The game concludes when the player correctly guesses the number or exhausts their attempts. In the latter case, the correct number is revealed.
The simplicity of the game, coupled with the dynamic use of the Random
class and clear rules, ensures an enjoyable and interactive user experience.
Use the Random()
Method to Generate Random Numbers in Java
Randomization injects excitement into games by introducing an unpredictable element.
The Random
class in Java is a cornerstone for achieving this. By generating pseudo-random numbers, developers can create dynamic and engaging applications.
In our case, we’ll utilize this class to create an unpredictable target number for players to guess in our number guessing game.
Source Code:
import java.util.Random;
import java.util.Scanner;
public class NumberGuessingGame {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
Random random = new Random();
int lowerBound = 1;
int upperBound = 100;
int numberToGuess = random.nextInt(upperBound - lowerBound + 1) + lowerBound;
int numberOfAttempts = 0;
int maxAttempts = 10; // Set the maximum number of attempts
boolean hasGuessedCorrectly = false;
System.out.println("Welcome to the Number Guessing Game!");
System.out.println("I have selected a number between " + lowerBound + " and " + upperBound
+ ". Try to guess it.");
while (!hasGuessedCorrectly && numberOfAttempts < maxAttempts) {
System.out.print("Enter your guess (or enter 0 to exit): ");
int userGuess = scanner.nextInt();
if (userGuess == 0) {
System.out.println("Thanks for playing. Goodbye!");
System.exit(0);
}
numberOfAttempts++;
if (userGuess == numberToGuess) {
hasGuessedCorrectly = true;
} else if (userGuess < numberToGuess) {
System.out.println("Try again. The number is greater than your guess.");
} else {
System.out.println("Try again. The number is less than your guess.");
}
}
if (hasGuessedCorrectly) {
System.out.println(
"Congratulations! You guessed the number in " + numberOfAttempts + " attempts.");
} else {
System.out.println(
"Sorry, you've run out of attempts. The correct number was: " + numberToGuess);
}
}
}
The number guessing game in Java follows a structured sequence of steps. Firstly, essential classes, including Random
for random number generation and Scanner
for user input, are imported.
Subsequently, bounds for the target number are set, ensuring they align with the desired range. The Random
class is then employed to generate a pseudo-random number within the specified range.
When an instance is created, it’s seeded with the current time. Subsequent calls to methods like nextInt()
produce sequences of seemingly random integers.
The class balances efficiency and unpredictability, offering a versatile tool for introducing randomness in applications, as seen in games, simulations, or any scenario where unpredictability is desirable.
The game is configured by determining the maximum number of attempts (maxAttempts
) and initializing variables to track player progress.
A friendly welcome message is displayed to provide information about the game. The core game logic unfolds within a while
loop, prompting users to guess until they either guess correctly or exhaust the allowed attempts.
User input is accepted, with special handling for the case where the player wants to exit the game (by entering 0). The user’s guess is evaluated, providing feedback on whether it’s higher or lower than the target number.
The game outcome is revealed, displaying a congratulatory message for a correct guess or informing the player of the correct answer if attempts are depleted.
This systematic approach ensures a clear and engaging user experience.
Output:
Welcome to the Number Guessing Game!
I have selected a number between 1 and 100. Try to guess it.
Enter your guess(or enter 0 to exit) : 50
Try again. The number is less than your guess.
Enter your guess(or enter 0 to exit) : 25
Try again. The number is greater than your guess.
Enter your guess(or enter 0 to exit) : 30
Try again. The number is less than yourguess.
Enter your guess(or enter 0 to exit) : 27
Try again. The number is less than your guess.
Enter your guess(or enter 0 to exit) : 26
Congratulations! You guessed the number in 5 attempts.
Upon running this program, the player will be greeted with a welcome message and prompted to guess a number between the specified bounds.
The game will provide feedback after each guess, and the outcome will be revealed at the end, showcasing the effectiveness of using the Random
class to create an engaging and dynamic number guessing game.
Conclusion
Crafting a random number guessing game in Java involves blending simplicity with engagement. Leveraging the Random
class provides unpredictability, while clear rules and user-friendly input enhance the gaming experience.
Striking a balance between code simplicity and effectiveness results in an entertaining and dynamic game that showcases the developer’s skill in creating an engaging user interaction.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn