How to Connect an FTPS Server in Java
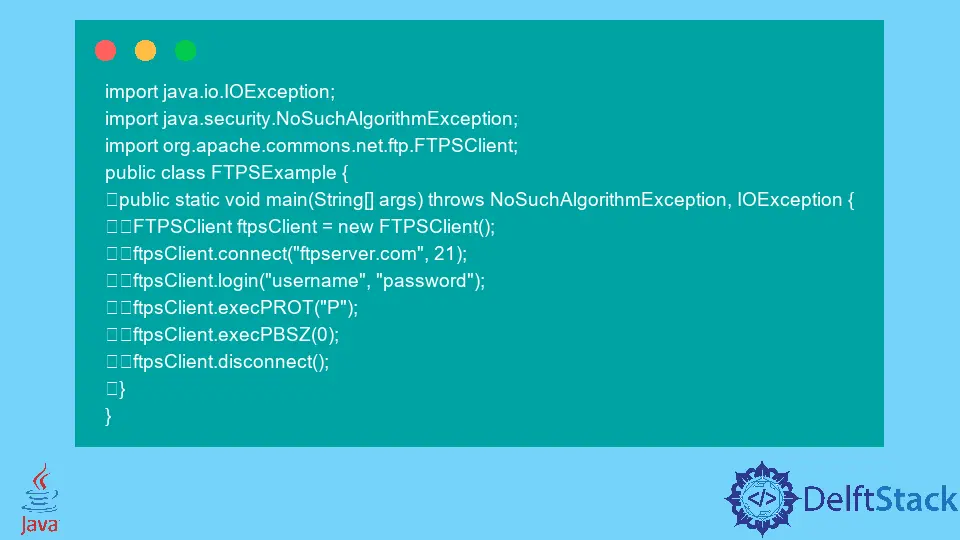
File Transfer Protocol Secure (FTPS) is a secure version of File Transfer Protocol (FTP), which uses SSL/TLS encryption to protect the data being transferred between a client and a server. This article will look at how to connect to an FTPS server in Java using the Java Secure Channel (JSSE) API.
FTP vs. FTPS
FTP is an old and widely-used protocol for transferring files between computers. While it is still widely used, it has several security issues that make it unsuitable for many applications.
One of the major issues is the lack of encryption, which makes it possible for eavesdroppers to intercept and read sensitive information, such as passwords and data being transferred.
FTPS is an extension of FTP that provides encrypted connections and improved security. There are two types of FTPS: Explicit FTPS and Implicit FTPS.
In explicit FTPS, the client requests a secure connection, while in implicit FTPS, the server requires a secure connection, and the client must comply. In this article, we will focus on explicit FTPS.
Connect an FTPS Server in Java
To follow this tutorial, you will need to have the following installed on your computer:
- Java Development Kit (JDK)
- An FTPS server to connect to (for example, FileZilla Server)
- A text editor
-
Setting up the FTPS Server
The first step is to set up an FTPS server. You can use any FTPS server that supports explicit FTPS, such as FileZilla Server.
To set up FileZilla Server, follow these steps:
- Download and install FileZilla Server from the official website.
- Launch the FileZilla Server Interface.
- Click on
Edit
>Settings
. - Go to the FTP
Settings
tab and check the"FTP over SSL/TLS"
option. - In the
SSL/TLS Settings
section, select the"Only allow secure FTP (FTPS) connections"
option. - Click
OK
to save the changes. - Start the server by clicking on
File
>Start Server
.
-
Writing the Java Code
Now that we have set up the FTPS server, we can write the Java code to connect to it. We will use the Java Secure Channel (JSSE) API to create a secure connection to the FTPS server.
Here is the code to connect to an FTPS server:
import java.io.IOException; import java.security.NoSuchAlgorithmException; import org.apache.commons.net.ftp.FTPSClient; public class FTPSExample { public static void main(String[] args) throws NoSuchAlgorithmException, IOException { FTPSClient ftpsClient = new FTPSClient(); ftpsClient.connect("ftpserver.com", 21); ftpsClient.login("username", "password"); ftpsClient.execPROT("P"); ftpsClient.execPBSZ(0); ftpsClient.disconnect(); } }
Let’s break down the code and see what each part does:
- The
FTPSClient
class is part of the Apache Commons Net library and implements the FTP protocol with support for secure connections using SSL/TLS. - The
connect
method connects to the FTPS server. It takes the server’s hostname, IP address, and port number (usually 21) as arguments. - The
login
method is used for logging in to the FTPS server using the specified username and password. - The
execPROT
method requests a secure connection. The argument"P"
indicates that we want to use a private (encrypted) connection. - The
execPBSZ
method sets the protection buffer size. A value of 0 disables protection buffers. - Finally, the
disconnect
method is used to close the connection to the FTPS server.
- The
-
Running the Code
To run the code, you must have the Apache Commons Net library on your
classpath
. You can download the library from the Apache Commons Net website.Compile the code using the following command:
javac FTPSExample.java
And then run the code using the following command:
java FTPSExample
If everything is set up correctly, you should see no output, which means the connection to the FTPS server was successful.
Note: This code will not run on any online compiler because you need to set up FTPS connection to run the code.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook