How to Find Memory Leak in Java
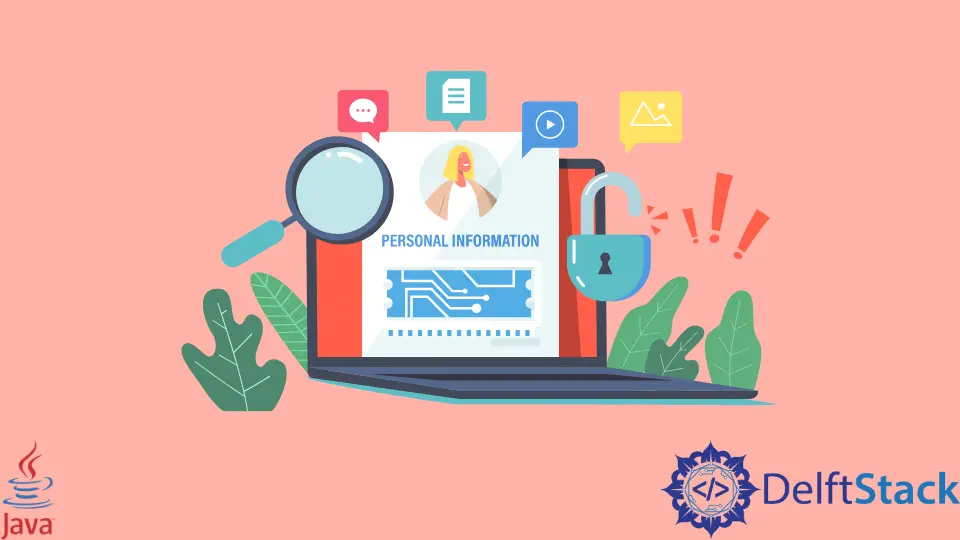
This article will teach us how to find a Java Memory Leak.
Unused items taking up extra memory space is known as a memory leak. Memory leaks are problematic because they clog memory resources and slow down system performance over time.
If this problem is not resolved, the program will ultimately run out of resources and crash with a fatal java.lang.OutOfMemoryError
.
One of its main advantages is the automatic memory management provided by Java’s built-in Garbage Collector
. The garbage collector may resolve the bulk of memory leak problems since it implicitly handles memory allocation and releasing.
Although the garbage collector successfully manages a large chunk of memory, it cannot provide a failsafe defense against memory leaks. The garbage collector is intelligent but not perfect.
Even apps created by a conscientious developer are susceptible to memory leaks.
Causes of Memory Leaks in Java
A Java memory leak often occurs when an application accidentally holds on to object references that are no longer needed. The Java garbage collection process cannot release the memory used by these objects because of the unintended object references.
The following are some of the most common causes of memory leaks.
- Caches with no limit.
- A large number of session objects.
- Insertion into Collection objects without deleting.
- Unused listener methods.
- Excessive page switching in the operating system.
- Custom data structures that are poorly written.
Find Memory Leaks in Java
Finding memory leaks can be challenging because there is no absolute way to find memory leaks. For finding the memory leaks in Java, we have some efficient ways, and those are:
Using Memory Profiler
Java profiling allows you to keep track of many JVM characteristics, such as method execution, object generation, thread execution, and trash collection. Memory profilers can track how much memory is being used and assist in finding memory leaks in an application.
Profilers may also assist in examining how resources are allocated inside an application, such as how much each function consumes memory and CPU. We can identify and find any problems using the Memory profiler.
Java profiling tools allow you to discover the following metrics.
- Details of method calls
- Usage of memory
- CPU time of a method
- The objects created
- The objects removed by the garbage collector.
Using Heap Dumps
It is another technique for finding memory leaks in Java. Heap dumps offer a snapshot of the heap memory used by a Java application at a specific moment.
They reveal the number of open object instances and their memory usage. Heap dumps can assist in determining how many objects are produced in an application and whether any of them are possibly causing memory leaks.
Verbose Garbage Collection
Verbose garbage collection can be enabled to get an in-depth trace of the Java garbage collection. Add the following argument to the JVM configuration to enable verbose garbage collection.
-verbose:gc
The summary displayed as the default error report can be used to learn how memory is handled and spot any memory leaks.
Using Eclipse Memory Leak Warnings
Using Eclipse’s memory leak warnings is another method for discovering memory leaks. Eclipse can alert you when a reference is terminated, but an object continues and is not closed if your code complies with JDK 1.5
or above.
Make sure that your project settings allow leak detection. Keep in mind that utilizing Eclipse might not be a complete solution.
In particular, if your code is not JDK 1.5
(or above) compatible, Eclipse may not catch all leaks and fail to detect some file closures.
Others Tools
-
NetBeans Profiler
– It can monitor memory, threads, and CPU resources and supports Java SE, Java FX, EJB, mobile apps, and Web applications. -
VisualVM
–VisualVM
, built on the NetBeans platform, is an easily extendable tool that uses a variety of plugins to offer you extensive information on your applications for monitoring remote and local programs. Using this program, you may manually run the garbage collector and obtain memory profiling. -
JProfiler
– It is a tool for profiling threads, memory, and CPUs that can also examine memory leaks and other performance problems. -
GCeasy
–GCeasy
is a tool that analyzes garbage collection logs and provides a simple approach to finding memory leak issues while reviewing garbage collection logs. The use ofGCeasy
is further encouraged by the fact that it can be accessed online without the requirement for computer installation. -
GC Viewer
– It is an open-source program that enables quick and simple information visualization using JVM data.GC Viewer
displays garbage collection performance information such as cumulative pauses, most extended pauses, and throughput.This program not only enables you to start garbage collection but also allows you to specify the initial heap size.
-
Patty in action
– It is another open-source program you may use to profile yourself and obtain targeted in-depth profiling. This tool may be used to examine heaps. -
JRockit
–JRockit
is a proprietary Oracle solution for Java SE applications that can forecast delay, display garbage collection, and analyze memory-related problems.
In this tutorial, we learned about memory leaks in Java, their different causes, and how to find them. Various tools and techniques are discussed in this tutorial.
Users can follow the techniques and tools to reduce memory leaks to improve the application’s performance.