The break Statement in Java
- What is the Break Statement?
- Using Break in Loops
- Using Break in Switch Statements
- Best Practices for Using Break Statements
- Conclusion
- FAQ
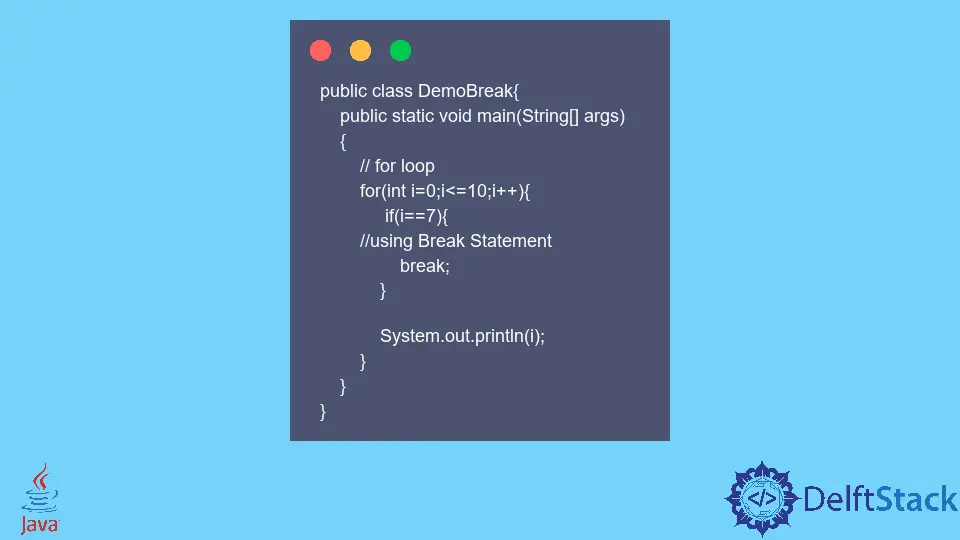
When diving into Java programming, understanding control flow statements is crucial for writing efficient code. One such statement is the break statement, which plays a vital role in controlling the flow of loops and switch statements.
This tutorial aims to demystify the break statement in Java, providing you with practical examples and insights on when and how to use it effectively. Whether you are a beginner looking to grasp the basics or an experienced coder needing a refresher, this guide will serve as a valuable resource. Let’s explore the ins and outs of the break statement and how it can enhance your Java programming skills.
What is the Break Statement?
The break statement is a control flow statement in Java that allows you to exit a loop or switch statement prematurely. It’s particularly useful when you want to stop the execution of a loop based on a specific condition. By using the break statement, you can prevent unnecessary iterations and streamline your code.
For instance, imagine you are searching for a specific value in an array. Once you find it, you can use the break statement to exit the loop immediately, rather than continuing to check the remaining elements. This not only makes your code cleaner but also improves performance by reducing unnecessary computations.
Using Break in Loops
One of the primary uses of the break statement is within loops, such as for, while, and do-while loops. This allows you to exit the loop when a certain condition is met. Let’s take a look at an example where we use the break statement in a for
loop.
public class BreakInLoop {
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
if (i == 5) {
break;
}
System.out.println(i);
}
}
}
Output:
0
1
2
3
4
In this code, we have a simple for
loop that iterates from 0 to 9. The loop checks if the current value of i
is equal to 5. When it is, the break statement is executed, causing the loop to terminate immediately. As a result, the output displays numbers from 0 to 4, illustrating how the break statement effectively stops the loop when the specified condition is met.
Using break statements in loops can greatly enhance the efficiency of your code, especially in scenarios where continuing the loop would be unnecessary or counterproductive. However, it’s essential to use them judiciously to avoid creating hard-to-read code.
Using Break in Switch Statements
Another common application of the break statement is in switch statements. In Java, once a case in a switch statement is executed, the program continues to execute subsequent cases unless a break statement is encountered. Let’s illustrate this with an example.
public class BreakInSwitch {
public static void main(String[] args) {
int day = 3;
switch (day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
default:
System.out.println("Invalid day");
}
}
}
Output:
Wednesday
In this example, we have a switch statement that evaluates the variable day
. When day
is equal to 3, the program prints “Wednesday” and encounters the break statement, which prevents the execution from falling through to the default case. This behavior is crucial for ensuring that only the intended case is executed. Without the break statement, all subsequent cases would execute, leading to unexpected results.
Understanding how to use break statements effectively in switch statements can help you manage complex decision-making scenarios in your code, making it more readable and maintainable.
Best Practices for Using Break Statements
While the break statement is a powerful tool in Java, it’s essential to use it wisely. Here are some best practices to keep in mind:
-
Avoid Overuse: Using break statements excessively can lead to spaghetti code, making it difficult to follow the program’s logic. Try to keep your code structured and clear.
-
Use Descriptive Conditions: When using break statements, ensure that the conditions are descriptive and meaningful. This will help others (and yourself) understand the intent behind the break.
-
Comment When Necessary: If a break statement is used in a complex loop or switch, consider adding a comment explaining why it’s there. This can be helpful for future reference.
-
Limit Scope: Use break statements within the smallest scope necessary. This will help maintain clarity and reduce the chances of unintended consequences.
By following these practices, you can make your code more efficient and easier to understand, ultimately leading to better programming habits.
Conclusion
The break statement in Java is an essential control flow tool that allows developers to exit loops and switch statements efficiently. By understanding how and when to use break statements, you can write cleaner, more effective code. Whether it’s to stop a loop early or to prevent fall-through behavior in switch statements, mastering the break statement will enhance your programming skills and improve your overall code quality. As you continue to practice and explore Java, keep the break statement in mind as a powerful ally in your coding toolkit.
FAQ
-
What does the break statement do in Java?
The break statement in Java is used to exit a loop or switch statement prematurely, allowing for more efficient code execution. -
Can I use break in nested loops?
Yes, you can use break in nested loops. It will only exit the innermost loop where it is called. -
What happens if I forget to use break in a switch statement?
If you forget to use break in a switch statement, the program will continue executing the following cases until it encounters a break or reaches the end of the switch. -
Are there alternatives to using break in loops?
Yes, alternatives include using return statements in methods or restructuring your code logic to avoid the need for a break. -
Is it considered bad practice to use break statements?
While break statements are not inherently bad, overusing them can lead to unclear code. It’s best to use them judiciously.