Java Block Comments
- Importance of Comments in Java Programming
- Single-Line Block Comments
- Multiline Block Comments
- Javadoc Comments
- Conclusion
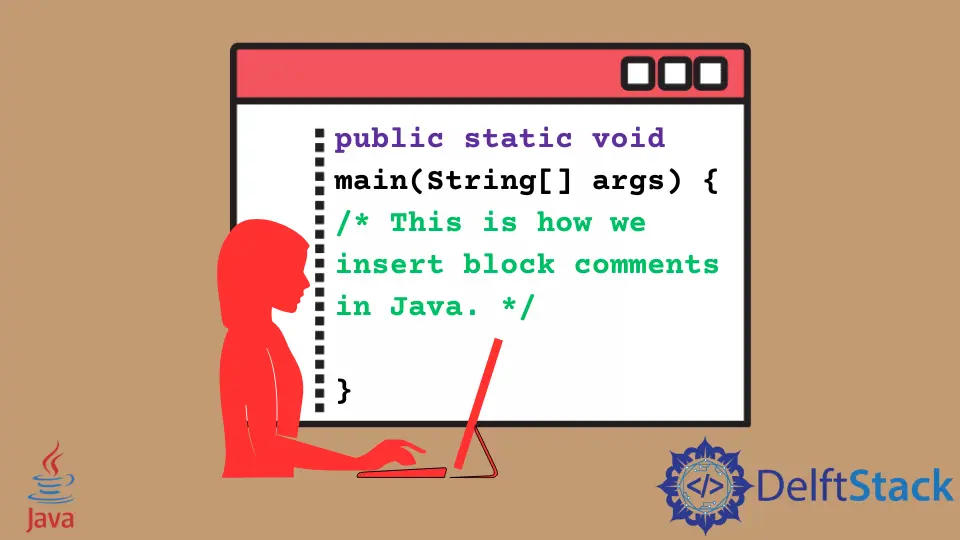
In Java, we use comments to improve the readability of Java code by providing additional explanations and information about the code, thereby helping maintain the code and find bugs and errors easily. While compiling the Java code, the compiler completely excludes the text and statements within the comments.
This article explores the pivotal role of various block comment methods, including single-line, multiline, and Javadoc, in fostering code clarity, documentation, and collaborative development in Java.
Importance of Comments in Java Programming
Comments in Java provide crucial explanations within code, aiding comprehension. They clarify complex logic, highlight key points, and enhance code readability.
Comments also facilitate collaboration among developers by conveying intent and purpose. Effective commenting simplifies maintenance, making it easier to debug, modify, and extend code, contributing to efficient and sustainable Java programming.
Single-Line Block Comments
Single-line comments in Java provide a quick and concise way to annotate code, making it readable and aiding in debugging. Unlike block comments, they are suitable for brief explanations on a single line, maintaining code clarity without introducing extra characters.
This simplicity makes single-line comments ideal for short, focused remarks, enhancing code comprehension and efficiency.
Let’s consider a simple Java program that calculates the sum of two numbers and utilizes single-line block comments for explanation:
public class SumCalculator {
public static void main(String[] args) {
// Declare and initialize two numbers
int num1 = 5;
int num2 = 10;
// Calculate the sum of num1 and num2
int sum = num1 + num2;
// Display the result
System.out.println("The sum of " + num1 + " and " + num2 + " is: " + sum);
}
}
In this example, we begin by defining a Java class named SumCalculator
. Inside the main
method, we declare and initialize two integers, num1
and num2
.
The subsequent line calculates the sum of num1
and num2
and stores the result in the variable sum
. Here, the single-line block comment clarifies the purpose of this line.
Finally, we utilize another single-line block comment to explain the System.out.println
statement, which displays the calculated sum.
By incorporating these comments, we enhance the code’s readability and provide valuable insights into the logic behind each step.
Upon executing this Java program, the output will be:
Single-line block comments, as demonstrated in this example, play a crucial role in making Java code more transparent and comprehensible. By adopting this practice, developers contribute to the creation of maintainable and collaborative codebases.
The effectiveness of single-line block comments lies in their simplicity and ability to succinctly convey the purpose of individual lines or blocks of code.
Multiline Block Comments
Multiline comments in Java serve as a versatile tool for adding detailed explanations or temporarily disabling code. Unlike single-line comments, they can span multiple lines, offering a comprehensive way to document code sections or provide extensive context.
This flexibility makes multiline comments valuable for in-depth explanations and annotations, contributing to code maintainability and collaboration among developers. These comments, enclosed within /* */
, are instrumental in providing comprehensive explanations and documentation for larger code segments.
Consider a Java program that calculates the area of a rectangle, utilizing multiline block comments for thorough documentation:
public class RectangleAreaCalculator {
public static void main(String[] args) {
// Define the dimensions of the rectangle
int length = 8;
int width = 5;
/*
Calculate the area of the rectangle
by multiplying length and width
*/
int area = length * width;
// Display the calculated area
System.out.println("The area of the rectangle is: " + area);
}
}
Let’s dive into the code and understand how multiline block comments enhance its readability.
In the RectangleAreaCalculator
class, we start by declaring two integers, length
and width
, to represent the dimensions of the rectangle.
The subsequent lines are enveloped in a multiline block comment, providing a comprehensive explanation of the logic behind calculating the area. It highlights that the area is determined by multiplying the length and width of the rectangle.
The final section of code uses a single-line block comment to clarify the System.out.println
statement, which displays the calculated area.
Upon executing this Java program, the output will be:
Multiline block comments are indispensable for elucidating complex code sections, such as mathematical calculations or intricate algorithms. This example illustrates how these comments contribute to code documentation, fostering better understanding and collaboration among developers.
By adopting a judicious use of multiline block comments, programmers can significantly improve the maintainability and comprehensibility of their Java code.
Javadoc Comments
Javadoc comments in Java are essential for generating automated documentation, enhancing code readability, and facilitating collaboration. Unlike regular comments, Javadoc comments follow a structured format, providing method, parameter, and return type details.
This standardized approach allows for the automatic creation of comprehensive documentation, aiding developers in understanding and utilizing code effectively. These comments, denoted by /** */
, are not just annotations but serve as a structured way to generate documentation.
Consider a Java program that implements a simple utility class for calculating the factorial of a number, showcasing the use of Javadoc comments:
/**
* The FactorialCalculator class provides methods for calculating the factorial of a number.
*/
public class FactorialCalculator {
/**
* Calculates the factorial of a given number.
*
* @param n The number for which to calculate the factorial.
* @return The factorial of the input number.
*/
public static int calculateFactorial(int n) {
if (n == 0 || n == 1) {
return 1; // Base case: factorial of 0 and 1 is 1
} else {
/*
Recursive case: Multiply the current number (n)
with the factorial of the previous number (n - 1)
*/
return n * calculateFactorial(n - 1);
}
}
/**
* Main method to demonstrate the usage of the FactorialCalculator class.
*/
public static void main(String[] args) {
int number = 5;
// Calculate and display the factorial of the number
System.out.println("The factorial of " + number + " is: " + calculateFactorial(number));
}
}
Let’s break down the code and understand how Javadoc comments contribute to clear documentation.
The FactorialCalculator
class is introduced with a Javadoc comment providing an overview of the class’s purpose - calculating the factorial of a number.
The calculateFactorial
method is then documented using Javadoc comments. The @param
tag explains the purpose of the n
parameter, and the @return
tag describes the method’s return value.
Within the method, a base case is established with a single-line comment. The recursive case is explained using a multiline block comment.
The main
method, responsible for demonstrating the class’s functionality, is also accompanied by a Javadoc comment.
Upon executing this Java program, the output will be:
Javadoc comments not only serve as documentation but also enable the automatic generation of comprehensive documentation for your Java code. By adopting this practice, developers enhance collaboration and understanding, making their codebases more maintainable and accessible.
This example showcases how Javadoc comments bring clarity to the purpose and usage of methods, contributing to effective communication within the development team.
Conclusion
Employing block comments in Java, whether single-line, multiline, or Javadoc, is paramount for code clarity, documentation, and collaboration.
Single-line comments offer succinct annotations, while multiline comments provide versatility for in-depth explanations. Javadoc comments, with their structured format, not only enhance readability but also enable automated documentation generation.
By judiciously using these block comment methods, developers foster a more comprehensible and maintainable codebase, promoting effective communication and streamlined development processes.