How to Accessor Methods in Java
- What Are Accessor Methods?
- Creating Accessor Methods in Java
- Benefits of Using Accessor Methods
- Conclusion
- FAQ
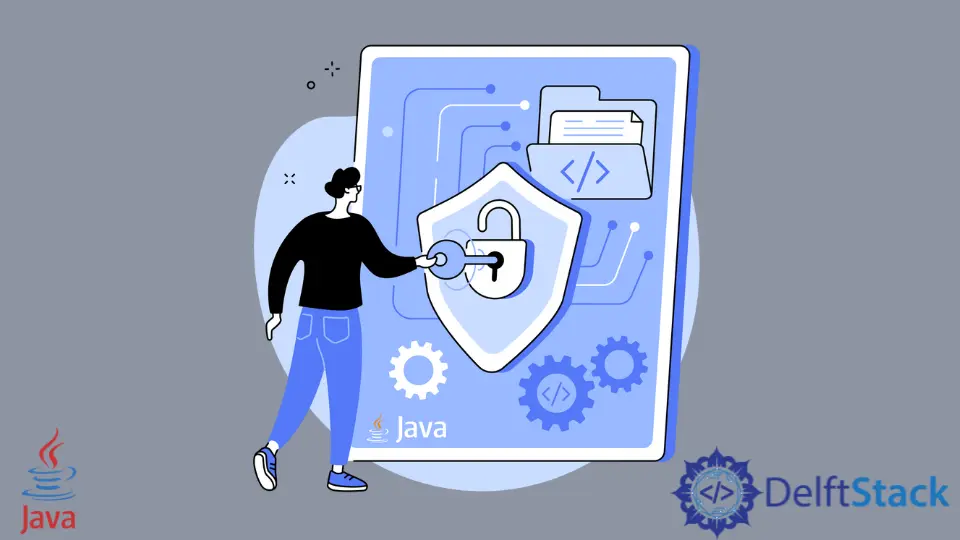
Accessor methods, often referred to as getter methods, are an essential part of object-oriented programming in Java. These methods allow you to retrieve the values of private variables from a class while maintaining encapsulation. Encapsulation is a fundamental principle of object-oriented programming that helps protect the internal state of an object.
In this tutorial, we will explore how to create and use accessor methods in Java, demonstrating their importance in maintaining clean and maintainable code. By the end of this guide, you’ll understand how to implement these methods effectively, ensuring your Java applications are robust and secure.
What Are Accessor Methods?
Accessor methods are functions defined in a class that allow you to access the values of private instance variables. In Java, it is a common practice to declare class variables as private to restrict direct access from outside the class. This encapsulation ensures that the internal state of an object can only be changed through methods, promoting better control over data.
The typical naming convention for accessor methods is to prefix the method name with “get” followed by the variable name, capitalizing the first letter. For example, if you have a private variable named name
, the accessor method would be getName()
. This method returns the value of the name
variable.
Here’s a simple example to illustrate this concept:
public class Person {
private String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
In this example, the Person
class has a private variable name
and an accessor method getName()
. This method allows you to retrieve the value of name
from an instance of the Person
class.
Accessor methods are crucial for maintaining encapsulation, as they allow controlled access to private data. They help ensure that the internal state of an object is not altered unexpectedly, thereby enhancing the reliability of your code.
Creating Accessor Methods in Java
To create accessor methods in Java, follow these steps:
- Define a Class: Start by defining a class with private instance variables.
- Add Constructor: Create a constructor to initialize the instance variables.
- Implement Accessor Methods: For each private variable, implement a corresponding accessor method.
Let’s look at a more detailed example that includes multiple accessor methods:
public class Car {
private String model;
private int year;
public Car(String model, int year) {
this.model = model;
this.year = year;
}
public String getModel() {
return model;
}
public int getYear() {
return year;
}
}
In this Car
class, we have two private variables: model
and year
. The constructor initializes these variables, and we have two accessor methods, getModel()
and getYear()
, to retrieve their values.
To use this class, you would create an instance of Car
and call the accessor methods like this:
Car myCar = new Car("Toyota Camry", 2020);
System.out.println("Model: " + myCar.getModel());
System.out.println("Year: " + myCar.getYear());
Output:
Model: Toyota Camry
Year: 2020
By using accessor methods, you can easily access the private variables of the Car
class without exposing the internal structure of the class. This encapsulation helps prevent unintended modifications and promotes better software design.
Benefits of Using Accessor Methods
Using accessor methods in Java provides several key benefits that enhance the overall design and functionality of your code.
-
Encapsulation: By restricting direct access to class variables, you can control how they are accessed and modified. This encapsulation helps maintain the integrity of your data.
-
Flexibility: If you need to change the internal representation of a variable, you can do so without affecting external code that uses the accessor methods. This flexibility makes your code easier to maintain.
-
Readability: Accessor methods can improve the readability of your code. When you use descriptive method names, it becomes clear what data is being accessed, making your code more understandable.
-
Validation: You can implement validation logic within accessor methods. For example, if you want to ensure that a variable is not set to an invalid value, you can add checks before returning the value.
Here’s an example that demonstrates the validation feature:
public class Employee {
private int id;
public Employee(int id) {
this.id = id;
}
public int getId() {
if (id < 0) {
throw new IllegalArgumentException("ID cannot be negative");
}
return id;
}
}
In this Employee
class, the getId()
method checks if the ID is negative before returning it. This additional layer of validation helps prevent logical errors in your application.
In summary, accessor methods play a crucial role in Java programming. They not only promote encapsulation and maintainability but also enhance code readability and allow for validation. By incorporating these methods into your classes, you can create a more robust and reliable codebase.
Conclusion
Accessor methods are a fundamental aspect of object-oriented programming in Java. They provide a means to access private variables while maintaining encapsulation, which is vital for creating secure and maintainable code. By following best practices for naming and implementing these methods, you can enhance the readability and flexibility of your Java applications. As you continue to develop your skills, remember the importance of accessor methods in ensuring that your classes are well-structured and your data remains protected.
FAQ
-
What are accessor methods in Java?
Accessor methods, also known as getter methods, are used to retrieve the values of private instance variables in a class, promoting encapsulation. -
Why are accessor methods important?
They help maintain encapsulation, improve code readability, and allow for validation of data before accessing it. -
How do I create an accessor method in Java?
Define a method with the prefix “get” followed by the variable name, ensuring it returns the value of the private variable. -
Can I add validation in accessor methods?
Yes, you can implement validation logic within accessor methods to ensure that the returned values meet specific criteria. -
What is the naming convention for accessor methods?
The convention is to prefix the method name with “get” followed by the variable name, with the first letter of the variable capitalized.