How to Print Page in HTML
-
Hide the Contents With
display:none
Inside@media print
to Print a Specific Content in HTML -
Pass the
id
to theonClick
Event Listener to Print a Specific Content in HTML
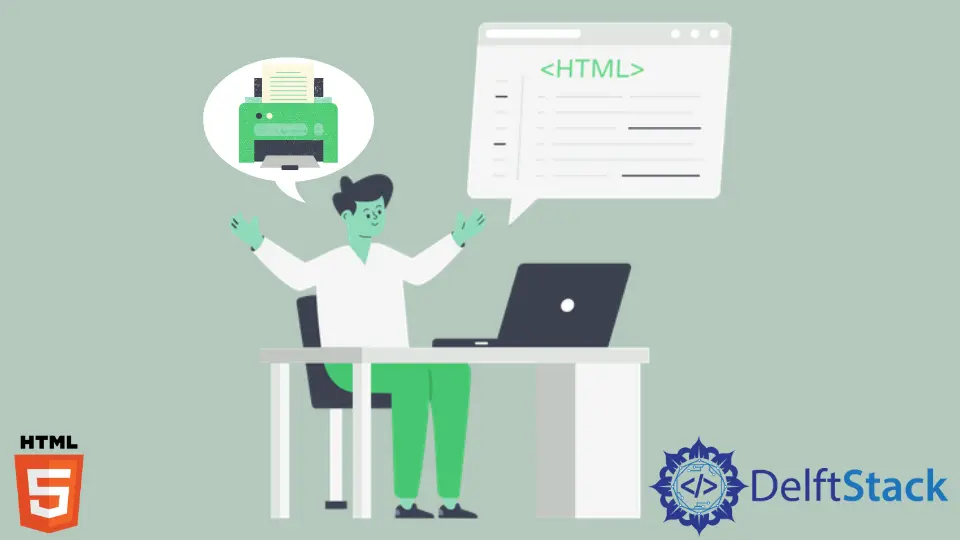
This article will introduce a few methods to print the specific content from a page in HTML.
Hide the Contents With display:none
Inside @media print
to Print a Specific Content in HTML
We can use CSS to change the appearance of the web page when it’s printed on paper. We can set one CSS style on the web and another CSS style while printing the web contents.
The CSS @media print
rule allows you to change the style of HTML contents while printing them.
We need JavaScript to print the HTML contents. The window.print()
method allows you to print the current window.
The print()
method opens the Print
Dialog Box, which lets you select preferred printing options. We can call window.print()
after clicking a button to print the current window.
Using @media print
, we can set the display
property to none
for the HTML contents, which we do not want to print. This will not show the HTML contents inside the printing option despite having those contents on the web.
For example, in HTML, create two headings using the h1
tag. Name the first heading as Need to print this
and the second heading as Don't need to print this
.
Give the class name for the second heading as dontPrint
. Then create a button and name it Print
.
Also, give this button a class name of dontPrint
. Create an onClick
listener for the button and call the window.print()
method when the button is clicked.
In the CSS section, inside @media print
, set the display property of the class named dontPrint
to none
.
The example below shows that the heading and button having the class name dontPrint
is not shown in the print section when the button Print
is clicked.
Example Code:
<h1>
Need to print this
</h1>
<h1 class="dontPrint">
Don't need to print this
</h1>
<button onclick="window.print();" class="dontPrint">
Print
</button>
@media print {
.dontPrint{
display:none;
}
}
Pass the id
to the onClick
Event Listener to Print a Specific Content in HTML
In this method, we can create an id
of the specific content to be printed. Then, we can pass the id
to a JavaScript function with the help of the onClick
event listener.
The JavaScript prints the specified content with the window.print()
method.
The innerHTML
property sets or returns the HTML content of an element. We can use it to select the content printed in JavaScript along with the document.getElementById()
method.
Similarly, we can preserve the original content of the HTML document using the innerHTML
property along with the document.body
property. After printing the content, we can set the original content of the HTML document in the document.body.innerHTML
property.
Let’s see the instructions below to understand it more clearly.
For example, create a div
and give it an id
of printIt
. Inside that div
, create an h1
tag and write Need to print this
inside it.
Outside the div
, create a button
and name it Print
. Create an onclick
listener for the button and call the printOut
function with the printIt
id as the parameter.
In the JavaScript section, create a function named printOut
with an argument divId
. Create a variable named printOutContent
to store innerHTML
of the divId
.
Next, create another variable originalContent
to store the innerHTMl
of the HTML body. The demonstration is shown below.
var printOutContent = document.getElementById(divId).innerHTML;
var originalContent = document.body.innerHTML;
Next, set innerHTML
of the body to the printOutContent
variable.
Then, call the window.print()
method. After calling the method, set innerHTML
of the body to originalContent
variable.
document.body.innerHTML = printOutContent;
window.print();
document.body.innerHTML = originalContent;
Here, when we call the window.print()
method, it will only print the section of the divId
id as document.body.innerHTML
contains only the element to be printed. After the content gets printed, the content of the whole HTML document is set to its original state.
Thus, we printed out a specific content of an HTML document using JavaScript.
Example Code:
<div id="printIt">
<h1>
Need to Print this
</h1>
</div>
<button onClick="printOut('printIt')">
Print
</button>
function printOut(divId) {
var printOutContent = document.getElementById(divId).innerHTML;
var originalContent = document.body.innerHTML;
document.body.innerHTML = printOutContent;
window.print();
document.body.innerHTML = originalContent;
}