How to Center a Form in HTML
-
Use the CSS
margin
Property to Center Form in HTML -
Use the CSS
text-align
Property to Center Form in HTML -
Use the CSS
width
andmargin-left
Properties to Center Form in HTML - Use Flexbox Centering to Center Form in HTML
- Use Grid Centering to Center Form in HTML
- Use Absolute Positioning to Center Form in HTML
- Conclusion
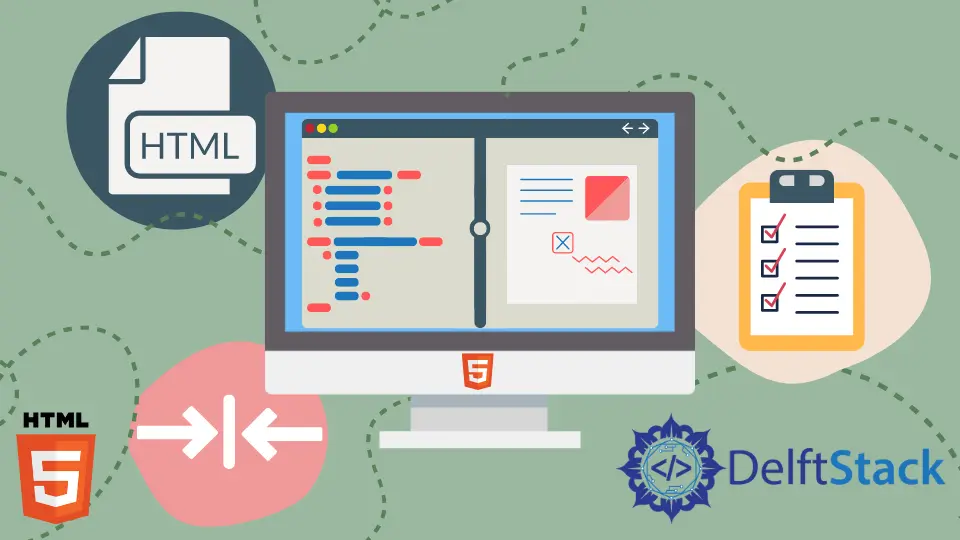
Centering elements in HTML, especially forms, is a crucial aspect of web development and design to create visually appealing and user-friendly interfaces. Achieving a centered form involves utilizing various CSS techniques to position the form element at the center of its container.
In this article, we’ll delve into several methods of how to center a form in HTML, exploring the intricacies of each method. From using classic techniques like margin manipulation to modern solutions involving Flexbox and CSS Grid, you’ll gain insights into the diverse ways of achieving centered forms.
Use the CSS margin
Property to Center Form in HTML
We can use the margin
CSS property to center a form in HTML. The styles can be written as inline CSS in the form
tag. The margin
property defines the space between the container and the adjacent elements.
First, we can provide the value to set a margin for the property. When we use a single value, the value applies to all four directions of the container div
.
Next, we can use the auto
value for the margin
property to center the form.
For this, we should provide a width
of the form for it to be adjusted in the center with the given width. The margin auto
value will split the remaining distance equally from the left and the right.
For example, create a form
tag and write the style
attribute in it. In the style attribute, set the margin
property to auto
and the width
property to 220px
.
Next, create an input
tag of text
and another input
tag of submit
.
The example below will create a center form. The form has a width of 220px
, and the remaining space is divided into left and right margins.
In this way, we can create a form with center alignment with margin:auto
using CSS in HTML.
<form style="margin: auto; width: 220px;">
Enter name:<input type="text" />
<input type="submit" value="Submit"/>
</form>
Output:
Use the CSS text-align
Property to Center Form in HTML
We use the text-align
property to specify the alignment of the text in CSS. We can use this property as the inline style of the form
tag to set the alignment of the form.
The text-align
property takes the values like left
, right
, center
, justify
, etc. We can set the value to center
to center the form.
For example, apply the text-align
property to the form
tag in the style
attribute and set the property to center
. Next, create input
tags with the type text
and then submit
.
The example below will center align all the contents of the form. However, the form occupies the whole block as no margin has been specified. In this way, we can center align the form in HTML.
<form style="text-align: center; ">
Enter name:<input type="text"/> <br><br>
<input type="submit" value="Submit"/>
</form>
Output:
Use the CSS width
and margin-left
Properties to Center Form in HTML
We can set the form’s width as 50% of the viewport width, and then we can provide 25% of the width as a margin to the left. We can use the width
and margin-left
CSS properties to set the width and add margin and achieve our goal.
Finally, we can use the vw
unit to specify the max width and the length. For example, set max width
to 50vw
and margin-left
to 25vw
as the style
attribute of the form
tag.
Here, the value 50vw
will occupy 50% of the viewport width, then add the margin, and the value 25vw
will give 25% of the viewport width as the left margin. The remaining 25% space will be in the right of the form.
In this way, we can center a form in HTML.
<form style=" width: 50vw; margin-left : 25vw;">
Enter name:<input type="text"/> <br><br>
<input type="submit" value="Submit"/>
</form>
Output:
Use Flexbox Centering to Center Form in HTML
Flexbox provides two primary properties, justify-content
and align-items
, that enable both horizontal and vertical alignment of elements within a container. To center a form element using Flexbox, we’ll employ these properties to position the form element precisely at the center of its parent container.
In the code below, the div
container utilizes Flexbox to center the contained <form>
element both horizontally and vertically within the viewport. The <form>
contains input elements for username, password, and a submit button, which will be centered within the container due to the Flexbox settings.
<style>
.container {
display: flex; /* Use flexbox */
justify-content: center; /* Horizontal centering */
align-items: center; /* Vertical centering */
height: 100vh; /* Set container height */
}
form {
width: 300px; /* Set a width for the form */
}
</style>
<div class="container">
<form>
<label for="username">Username:</label>
<input type="text" id="username" name="username"><br><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password"><br><br>
<input type="submit" value="Submit">
</form>
</div>
Output:
This approach simplifies the process to center align the form within the webpage and ensures the form remains responsive across different screen sizes due to the use of Flexbox properties for alignment and the defined width for the form.
Using Flexbox With Margin Auto
By combining Flexbox with the margin: auto;
property for the form, you achieve precise horizontal centering within the container.
<style>
.container {
display: flex; /* Use flexbox */
justify-content: center; /* Horizontal centering */
align-items: center; /* Vertical centering */
height: 100vh; /* Set container height */
}
form {
width: 300px; /* Set a width for the form */
margin: auto; /* Set margins to 'auto' */
}
</style>
<div class="container">
<form>
<label for="username">Username:</label>
<input type="text" id="username" name="username"><br><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password"><br><br>
<input type="submit" value="Submit">
</form>
</div>
Output:
Use Grid Centering to Center Form in HTML
CSS Grid is a two-dimensional layout system that enables precise control over rows and columns, offering an intuitive way to create complex web layouts. Leveraging its capabilities allows for both simple and sophisticated arrangements of content within a designated grid container.
In the code below, the div
container uses CSS Grid to create and display a grid-based layout. The place-items: center;
property centers the contained <form>
element both horizontally and vertically within the grid container.
The <form>
contains input elements for username, password, and a submit button, which will be centered within the container due to the CSS Grid settings.
<style>
.container {
display: grid; /* Use CSS Grid */
place-items: center; /* Center items both horizontally and vertically */
height: 100vh; /* Set container height */
}
form {
width: 300px; /* Set a width for the form */
}
</style>
<div class="container">
<form>
<label for="username">Username:</label>
<input type="text" id="username" name="username"><br><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password"><br><br>
<input type="submit" value="Submit">
</form>
</div>
Output:
This method offers a concise way to center align the form using CSS Grid’s powerful grid-based layout capabilities, ensuring the form remains centered within the viewport regardless of screen size.
Use Absolute Positioning to Center Form in HTML
Absolute positioning allows elements to be placed at specific coordinates within their containing elements, disregarding the document flow. This technique positions an element relative to its closest positioned ancestor, typically the nearest parent container with a defined position
property other than static
.
In the code below, the position: absolute;
and transform: translate(-50%, -50%);
combination allows the form to be precisely centered within the div
container, both horizontally and vertically, irrespective of the container’s size or the form’s dimensions.
This method using absolute positioning and transform
provides a straightforward way to center the form within its container while maintaining its responsiveness across different viewport sizes.
<style>
.container {
position: relative; /* Set parent container position to relative */
height: 100vh; /* Set container height */
}
form {
width: 300px; /* Set a width for the form */
position: absolute; /* Set form position to absolute */
top: 50%; /* Move the form down by 50% of its height */
left: 50%; /* Move the form right by 50% of its width */
transform: translate(-50%, -50%); /* Center the form */
}
</style>
<div class="container">
<form>
<label for="username">Username:</label>
<input type="text" id="username" name="username"><br><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password"><br><br>
<input type="submit" value="Submit">
</form>
</div>
Output:
Conclusion
In this article, we learned the following:
- Basic Techniques: We started with simple methods like
margin
manipulation andtext-align
to align form horizontally. - Viewport Units and Percentages: Techniques employing percentages and viewport units allowed flexible and responsive form centering based on the viewport dimensions.
- Advanced Approaches: Moving into Flexbox and CSS Grid, we encountered powerful layout tools. Flexbox streamlined both horizontal and vertical centering, while CSS Grid provided intricate control over form alignment within the viewport.
- Absolute Positioning: Leveraging absolute positioning with
transform
, we achieved precise centering irrespective of container size or form dimensions.