How to Create a New Directory in Go
-
Permission Modes of
os.Mkdir
andos.MkdirAll
-
Use the
os.Mkdir()
Method in Go -
Use the
os.MkdirAll()
Method in Go
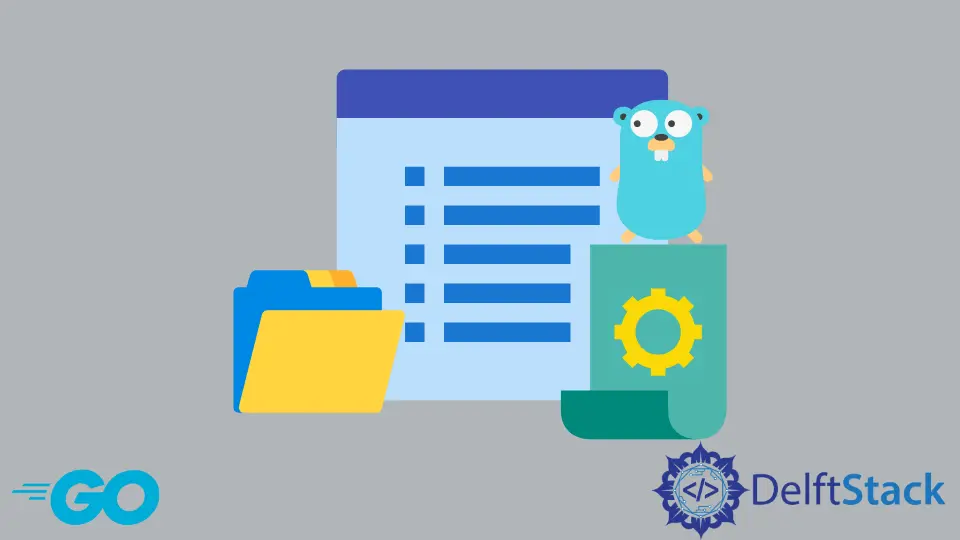
Go has many methods to create directories, one being os.Mkdir
. We can assign the specified name and permission bits to the newly formed directory.
The os.MkdirAll
helps create several directories recursively, including any missing parents.
Permission Modes of os.Mkdir
and os.MkdirAll
Notation | Octal Integer | Functionality |
---|---|---|
rwx |
7 |
Read, write and execute |
rw- |
6 |
Read, write |
r-x |
5 |
Read, and execute |
r-- |
4 |
Read |
-wx |
3 |
Write and execute |
-w- |
2 |
Write |
--x |
1 |
Execute |
--- |
0 |
No permissions |
Users Access
Permission | Octal | Field |
---|---|---|
rwx------ |
0700 |
User |
---rwx--- |
0070 |
Group |
------rwx |
0007 |
Other |
Common Permissions
0664
: Only the owner can read and write into the file. Whereas other users can only read the file.0600
: Only the owner can read and write into the file, and no one else can access it.0777
: All the users have full access to the file.0755
: Only the owner has full access, while other users can only view and execute the file.0666
: Read and write access to all the file users.0750
: The file owner can read, write and execute it, but the users can only read and execute it.
Use the os.Mkdir()
Method in Go
package main
import (
"fmt"
"log"
"os"
)
func main() {
error := os.Mkdir("HomeDir", 0750)
if error != nil && !os.IsExist(error) {
log.Fatal(error)
}
fmt.Print("The directory has been created successfully.")
}
Output:
The directory has been created successfully.
The above code allows us to create a new directory. If there were to be an error while creating the new directory, it would be of type PathError
.
The os.Mkdir
function only makes a single directory. We can create subdirectories using this function.
Use the os.MkdirAll()
Method in Go
package main
import (
"log"
"os"
"fmt"
)
func main() {
DirPath := "Desktop/Documents/MyDirectory"
err := os.MkdirAll(DirPath, os.ModePerm)
if err != nil {
log.Println(err)
}
fmt.Print("The directory has been created successfully.")
}
Output:
The directory has been created successfully.
It is helpful to make a function for creating multiple directories using a single command. The missing parent directories are automatically made, which avoids the redundancy of writing code for making new directories.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn