How to Run Docker Instance From Dockerfile
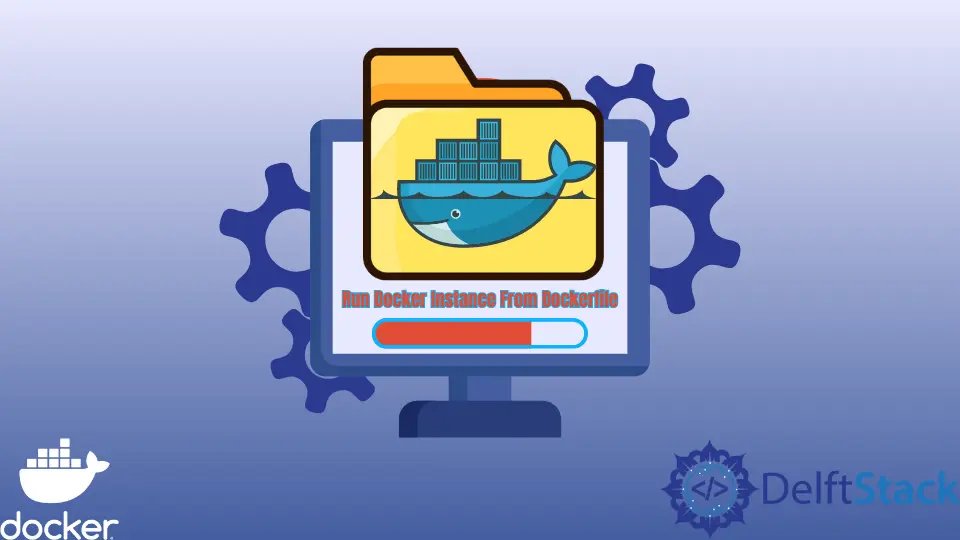
Docker containers have undoubtedly become the standard unit when it comes to the management of software and dependencies in different environments. When working with real applications, you must create a docker file before building your application’s container image.
A Dockerfile is simply a read-only text document with instructions set when assembling a Docker image. On the other hand, a Docker image is a set of instructions, application code, dependencies, tools, and libraries used to build a Docker container.
Therefore, a container is a runnable instance of the docker image assembled from a Dockerfile.
This article will walk you through the steps to create a Dockerfile and run a docker instance from this file.
Steps to Run Docker Instance From Dockerfile
We need to follow the steps below to run a Docker instance from the Dockerfile.
Create a Dockerfile
To create a Dockerfile, we must understand its components. Some of the most common commands include:
FROM
: creates a layer of the parent/base image used.WORKDIR
: allows us to set the working directory.COPY
: enables us to copy current directory contents into a directory in the container.PULL
: adds files from your Docker repository.RUN
: is executed when we want to build the image.CMD
: specifies what command to run when the container starts.ENV
: defines environmental variables used during the build.ENTRYPOINT
: determines what command to run when the container starts.MAINTAINER
: specifies the author of the image.
To create a Dockerfile, we will first begin by creating the main directory that will host, among other files, the Dockerfile. We will be making a simple Flask application that prints a message on the console.
mkdir my-app
Now, we move into this directory and create the main file of your application as app.py
. This file contains the application code for the program.
from flask import Flask
app = Flask(__name__)
def hello():
print("Hello, this is a simple Flask application")
hello()
We can now proceed to create our Dockerfile and populate it with the necessary commands to create a Docker image.
touch Dockerfile
We also created a requirements.txt
file that contains the necessary file to install to run this application. The requirements are shown below.
click==8.0.4
Flask==2.0.3
gunicorn==20.1.0
itsdangerous==2.1.0
Jinja2==3.0.3
MarkupSafe==2.1.0
Werkzeug==2.0.3
We will edit the Dockerfile and add the following commands to create docker images using the docker build
command. In this case, Python is the base image.
We have also set the working directory and copied the necessary files from the current directory to the directory in the Docker container.
# base image
FROM python
# Set your working directory
WORKDIR /var/www/
# Copy the necessary files
COPY ./app.py /var/www/app.py
COPY ./requirements.txt /var/www/requirements.txt
# Install the necessary packages
RUN pip install -r /var/www/requirements.txt
# Run the app
CMD ["echo", "Hello, Developer"]
Create a Docker Image
We proceed to create a Docker image using the docker build
command. However, we have to run this command in the same directory.
Syntax:
$ docker build [OPTIONS] PATH | URL | -
Inside the my-app
directory, we will execute the command below. The -t
flag enables us to tag the name of the image and indicate that the Dockerfile is in the same directory that we are executing this command.
~/my-app$ docker build -t new_image .
Output:
[+] Building 184.4s (10/10) FINISHED
=> [internal] load build definition from Dockerfile 1.5s
=> => transferring dockerfile: 38B 0.0s
=> [internal] load .dockerignore 1.9s
=> => transferring context: 2B 0.0s
=> [internal] load metadata for docker.io/library/python:latest 50.8s
=> [1/5] FROM docker.io/library/python@sha256:3204faabc2f0b5e0939bdb8b29079a2a330c38dee92a22482a9ed449c5649a55 30.4s
=> => resolve docker.io/library/python@sha256:3204faabc2f0b5e0939bdb8b29079a2a330c38dee92a22482a9ed449c5649a55 0.4s
=> => sha256:3204faabc2f0b5e0939bdb8b29079a2a330c38dee92a22482a9ed449c5649a55 2.14kB / 2.14kB 0.0s
=> => sha256:17e2d81e5757980ee40742d77dd5d3e1a69ad0d6dacb13064e1b018a6664ec72 2.22kB / 2.22kB 0.0s
=> => sha256:178dcaa62b393b539abc8b866c39be81e8ade01786880dc5d17ce3fe02426dbb 8.55kB / 8.55kB 0.0s
=> => sha256:38121472aa0128f87b31fde5c07080418cc17b4a8ee224767b59e24c592ff7d3 2.34MB / 2.34MB 10.4s
=> => extracting sha256:38121472aa0128f87b31fde5c07080418cc17b4a8ee224767b59e24c592ff7d3 14.6s
=> [internal] load build context 1.1s
=> => transferring context: 195B 0.0s
=> [2/5] WORKDIR /var/www/ 3.2s
=> [3/5] COPY ./app.py /var/www/app.py 1.9s
=> [4/5] COPY ./requirements.txt /var/www/requirements.txt 2.6s
=> [5/5] RUN pip install -r /var/www/requirements.txt 82.3s
=> exporting to image 8.1s
=> => exporting layers 6.0s
=> => writing image sha256:5811f24b498ae784af32935318a5fddba536e2be27233b19bf08cad81438d114 0.2s
=> => naming to docker.io/library/new_image
We can now list the docker images using the docker images
command below.
~/my-app$ docker images
Output:
REPOSITORY TAG IMAGE ID CREATED SIZE
new_image latest 5811f24b498a 2 minutes ago 929MB
Run Instance From the Dockerfile
To create a runnable instance from this docker image, we will use the docker run
command to create a writable layer over the image we created earlier.
Syntax:
docker run [OPTIONS] IMAGE [COMMAND] [ARG...]
Finally, we can now create a runnable instance using the command above.
~/my-app$ docker run -it new_image
Hello, Developer
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn