How to Recreate Containers From New Images Using Docker-Compose
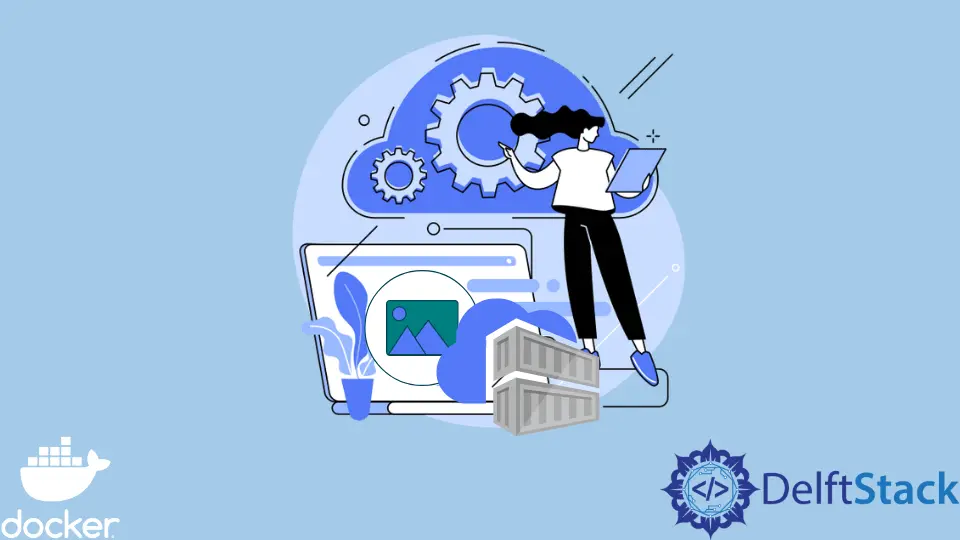
When developing applications, we usually make changes or add more features to make the application more effective for the different users interacting with the applications.
When working with Docker, we need to ensure that the changes or features made are updated to the main application by rebuilding the image and running a new container from this image. This tutorial will teach us how to recreate containers from new images using Docker Compose.
Create a New Project
In WebStorm IDEA
, select File
> New
> Project
to create a new project. Then select Empty Project
and change the project name from untitled
to docker-compose-no-cache
or use any name preferred.
Press the button labeled Create
to generate the project. Create a file named index.html
under the current folder and copy and paste the following code.
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Bootstrap demo</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity="sha384-Zenh87qX5JnK2Jl0vWa8Ck2rdkQ2Bzep5IDxbcnCeuOxjzrPF/et3URy9Bv1WTRi"
crossorigin="anonymous">
</head>
<body>
<div class="card">
<div class="card-header">
Featured
</div>
<div class="card-body">
<h5 class="card-title">Special title treatment</h5>
<p class="card-text">With supporting text below as a natural lead-in to additional content.</p>
<a href="#" class="btn btn-primary">Go somewhere</a>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/js/bootstrap.bundle.min.js"
integrity="sha384-OERcA2EqjJCMA+/3y+gxIOqMEjwtxJY7qPCqsdltbNJuaOe923+mo//f6V8Qbsw3"
crossorigin="anonymous"></script>
</body>
</html>
This file uses Bootstrap to create a web page that displays a card when we run a container from the application’s image. We will use this page to verify whether our container is running.
Define an Image
Create a file named Dockerfile
under the current folder and copy and paste the following instructions into the file.
FROM nginx:1.22.0-alpine
COPY . /usr/share/nginx/html
FROM
- Sets the base image on which to create our custom image using subsequent instructions. In this case, we have set our base image as Nginx
and used alpine
to pull a lightweight version of Nginx
.
COPY
- Copies the files and folders in the current directory to a file system location in our image. In this case, we have copied all the files in the current directory to /src/share/nginx/html
.
Define a Container Using Compose File
Create a file named compose.yaml
under the current folder and copy and paste the following instructions into the file.
services:
web-app:
restart: on-failure
build: ./
hostname: web-app-service
ports:
- '80:80'
This file defines a service named web-app
that exposes port 80
in the host to listen on port 80
in the container. This file provides an easier way to manage containers than the containers on the terminal.
Build an Image and Run a Container
Since this is the first time we’re building an image, our base image will be pulled and used to create our custom image using the instructions defined in the Dockerfile
. The container defined in the compose.yaml
file will also be created and added to a network.
Open a new terminal window using the keyboard shortcut ALT+F12 on your computer and execute the command below to build an image and run a container from it.
~/WebstormProjects/docker-compose-no-cache$ docker compose up -d
This command executes the compose.yaml
file to build an image named docker-compose-no-cache_web-app
and also runs a container with the name docker-compose-no-cache-web-app-1
, as shown below.
=> CACHED [1/2] FROM docker.io/library/nginx:1.22.0-alpine@sha256:addd3bf05ec3c69ef3e8f0021ce1ca98e0eb21117b97ab8b64127e 0.0s
=> [2/2] COPY . /usr/share/nginx/html 0.6s
=> exporting to image 0.8s
=> => exporting layers 0.6s
=> => writing image sha256:d72675b7a3e3a52dd27fe46f298dc30757382d837a5fbf36d8e36d646b5902d6 0.1s
=> => naming to docker.io/library/docker-compose-no-cache_web-app 0.1s
Use 'docker scan' to run Snyk tests against images to find vulnerabilities and learn how to fix them
[+] Running 2/2
⠿ Network docker-compose-no-cache_default Created 0.3s
⠿ Container docker-compose-no-cache-web-app-1 Started
To verify whether our container is running, open the browser and issue a request to localhost:80
(http://localhost/#). The card we defined in the index.html
page will be displayed on the browser.
Using the same terminal window, execute the command below to verify that our custom image was created.
~/WebstormProjects/docker-compose-no-cache$ docker image ls
Output:
REPOSITORY TAG IMAGE ID CREATED SIZE
docker-compose-no-cache_web-app latest d72675b7a3e3 8 minutes ago 23.5MB
Rebuild an Image With Cache
To rebuild the existing image with cache and run containers, we first need to stop and remove the existing containers using the command docker compose down
.
Rebuilding a docker image with the cache means the current container will be reused to create a new container. Using the same terminal window, execute the following command to rebuild the existing image with the cache.
~/WebstormProjects/docker-compose-no-cache$ docker compose build
This command rebuilds a new image using the existing image, and we can use the docker compose up -d
to run a container from it.
To verify that the existing image is reused to create a new container, execute the following command and note that there is no image apart from the image created in the previous section.
~/WebstormProjects/docker-compose-no-cache$ docker image ls
Output:
REPOSITORY TAG IMAGE ID CREATED SIZE
docker-compose-no-cache_web-app latest d72675b7a3e3 8 minutes ago 23.5MB
Rebuild an Image With No Cache
Rebuilding an image with no cache means creating a new image without reusing the previous image. We can achieve this by adding the --no-cache
command when building an image using the docker build
command.
Using the same terminal window, execute the following command to rebuild an image with no cache. Ensure running containers are removed using the docker compose down
command.
~/WebstormProjects/docker-compose-no-cache$ docker compose build --no-cache
This command rebuilds a new image with no cache meaning that the previous image is not reused. To verify that the previous image was not reused to create the current image, execute the following command and note that the previous image has no name as indicated by <none>
.
The new image uses the name of the previous image.
~/WebstormProjects/docker-compose-no-cache$ docker image ls
Output:
docker-compose-no-cache_web-app latest f111dde0e704 3 seconds ago 23.5MB
<none> <none> 1c05a46fe049 13 minutes ago 23.5MB
Since the previous image and the new image cannot have the same names, the current image uses the name of the previous image, and the previous image gets none
to show that it has no name.
Conclusion
We have learned how to recreate containers from new images using Docker Compose. To implement this, we have learned how to rebuild an image with the cache and how to rebuild an image with no cache.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHub