Select_related Method in Django
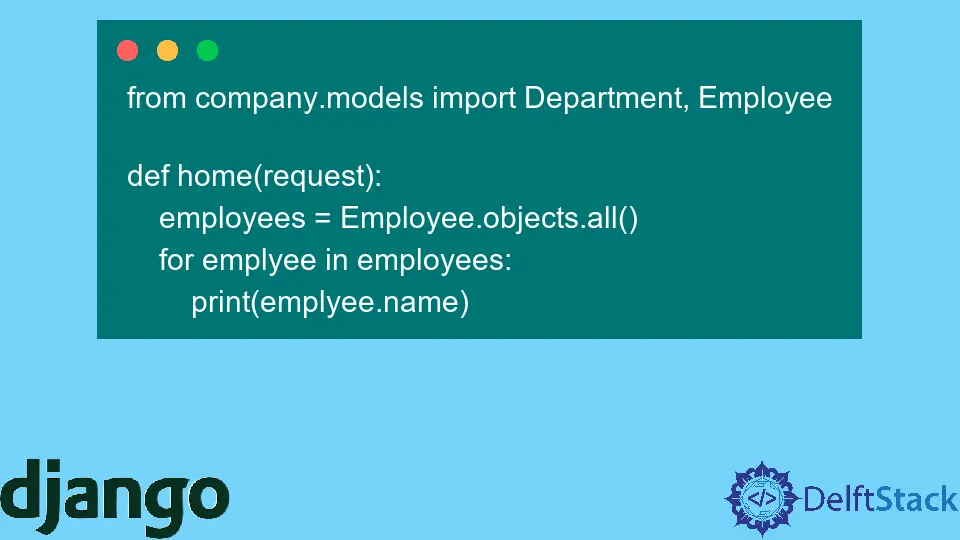
The article tackles query sets, how to work these queries, and how to use the select_related()
method in Django. While using the Django framework, we mostly use the query set
.
Suppose we have a database and we are going to perform a query. Let’s assume that we have a model called the supplier
, and through with this supplier
, we will grab all the suppliers, so we are going to have a list of suppliers, and that list will be called a query set
.
Use the select_related()
Method to Filter the Related Query of Model in Django
We’ll look at the views.py
file, where we will write logic and create two models. We’ll start to write some code explaining the use case of the select_related()
method.
First, we fetched employees using objects.all()
. We need to loop through that employee’s list and print employee names using emplyee.name
.
Code:
from company.models import Department, Employee
def home(request):
employees = Employee.objects.all()
for emplyee in employees:
print(emplyee.name)
Output:
Jane Doe
John Doe
Simpson
John Simpson
If we use the debug tool and go to our browser, we will see that it fetches all employees and corresponding departments and their details. Since the objects.all()
select all employees and gets all details.
The issue starts when we access the related model. For example, we look into our models.py
file, and the employee
model that is a related model is the department
itself.
We’ll print the employee name plus their department name using the following code.
Code:
from company.models import Department, Employee
def home(request):
employees = Employee.objects.all()
for emplyee in employees:
print(emplyee.name, emplyee.department.name)
We use the emplyee.department
in the above code, and the department is a foreign key. It will give us that department
object where is our department names.
Output:
Jane Doe HR
John Doe Accounts
Simpson Purchase
John Simpson Purchase
We can see multiple queries if we go into the browser while running our Django application with the help of the Django debug tool. We can see in the first query that it selects all the employees, and the second one selects the department, which has an id of 1
.
If we have the same id of the employee
even though it is the same underlying, the ORM will go twice into that table and fetches the duplicates. Suppose we have a hundred employees in our company, then it will execute 101
queries, so 100
queries from the department
and one query from the employee
.
This is also known as a classical endless one problem in ORM. It will fetch those employees and their corresponding departments using the select_related()
method, and we need to pass the field of our model in the method.
So the field makes sense to be related, and in our case, we use the field as a department
.
Code:
from company.models import Department, Employee
def home(request):
employees = Employee.objects.all().select_related("department")
for emplyee in employees:
print(emplyee.name, emplyee.department.name)
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn