How to Slug in Django
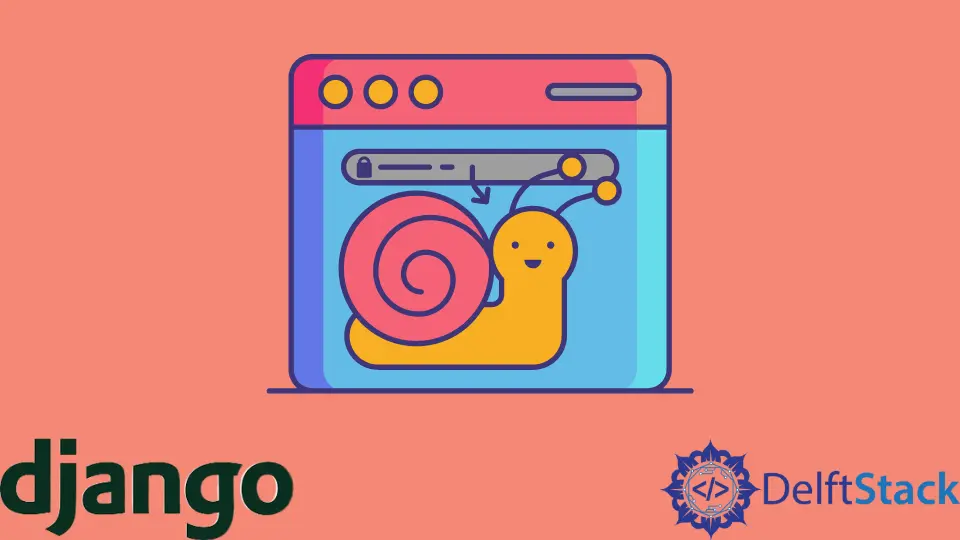
This demonstration aims to define a slug and how we can use the slug field to get a unique post with Django in Python.
Use Slug to Get Unique Post in Django
A slug would be something that is a little bit more shareable for our actual post itself.
For example, if we trigger http://127.0.0.1:8000/post/pa-roate-10
, we can go like this, but this does not look good. That is why it introduced the concept of slug in Django.
Instead of the one above, we can make it as http://127.0.0.1:8000/post/Djangoslug
if we have the Django Slug
title. We can then trigger our post using its post title.
Another thing that we can do is, instead of space, we can use -
and convert this into lowercase. Suppose this is our title Django Slug
, and if we convert this into lowercase with -
, we have django-slug
.
Use the Slug Field in Django
Now, let’s see how to use the slug field in Django. We can pass the slug to the URL between the greater (>
) and lesser (<
) symbols.
We can specify it with specific data types. views.categoryview()
calls a function from the views.py
file, then that function will render the HTML content.
urlpatterns = [
path("category/<str:cats>/", views.categoryview(), name="category"),
]
Let’s jump into our model.py
file and call a slug with models.SlugField()
. Then we pass True
to the unique
parameter.
This is a required field. Since we have set unique=True
, we set the post title with their unique names in a database.
slug = models.SlugField(max_length=100, unique=True)
So inside our models.py
file, we can create a signal that will do something before this is saved. We need to import the signal using the following code.
from django.db.models.signals import pre_save
To turn our title into a slug, we need to import the slugify
class. So our title, Django Slug
, will then turn into django-slug
.
from django.template.defaultfilters import slugify
Let’s define a function to be a signal receiver. We need to pass the sender, an instance, arguments, and keyword arguments.
In this defined function, first, we get the slug and make sure that the slug does not already exist. We will check to see if it exists by doing a filter.
We write the slug equal to a string like this "%s-%s"
. This will be the instance ID, so we can get the original slug by calling that.
def pre_save_post_reciever(sender, instance, *args, **kwargs):
slug = slugify(instance.title)
exists = post.objects.filter().exists()
if exists:
skug = "%s-%s" % (slug, instance.id)
instance.slug = slug
pre_save.connect(pre_save_post_reciever, sender=post)
It executes this before being saved every time a method of pre_save()
is called. The pre_save_post_reciever()
function will update our slug accordingly.
from django.db.models.signals import pre_save
from django.dispatch import receiver
from django.template.defaultfilters import slugify
def pre_save_post_reciever(sender, instance, *args, **kwargs):
slug = slugify(instance.title)
exists = post.objects.filter().exists()
if exists:
skug = "%s-%s" % (slug, instance.id)
instance.slug = slug
pre_save.connect(pre_save_post_reciever, sender=post)
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn