How to Log Messages to the Console in Django
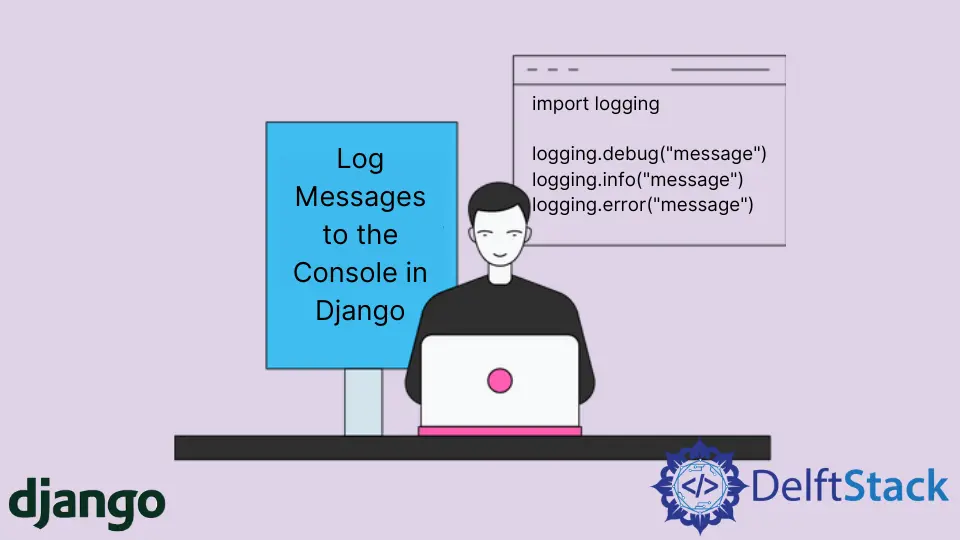
When we install a set of software on our machine, we often find a log file or see some messages being printed inside the console of that application. These are log messages that provide information about the events occurring in the application, tasks that the software is completing, errors encountered, warnings, etc. These messages help software developers improve their application and debug it if users allow the application to share the error reports.
When working with Django or any other framework for a project, we generally use print statements to debug our applications. Print statements are grand; they are built-in and very effortless to use. But we can take this thing to the next level if we start using packages or libraries dedicated to this purpose. These libraries let us define levels for our log messages and effortlessly print additional information like line numbers and timestamps. Some libraries even let us add colors to our log statements or messages.
In this article, we’ll learn how to log messages to the console in Django projects.
Log Messages Using Build-In Python Library logging
Python comes with a built-in library logging
, whose sole purpose is to help implement a flexible event logging system. In this article, we won’t go over everything but cover only the basics and a few essential things.
When working with logging
, we use a logger
to log messages to the console. As mentioned above, the log messages have some levels that indicate the purpose of a log message. This library has five such levels, and each level has a seniority number attached to it. For example, the CRITICAL
level has the highest seniority of 50. DEBUG
has 10. INFO
, WARNING
, and ERROR
have 20, 30, and 40, respectively.
DEBUG
- Used to print messages to debug an application.INFO
- Used to print general messages such as affirmations, confirmations, and success messages.WARNING
- Used to report warnings, unexpected events due to inputs, indications for some problems that might arise in the future, etc.ERROR
- Used to alert about serious problems that most probably will not stop the application from running.CRITICAL
- Used to alert about critical issues that can harm the user data, expose the user data, hinder security, stop some service, or maybe end the application itself.
The logging
library has functions we can use to log messages. To demonstrate, check the code below:
import logging
logging.debug("Log message goes here.")
logging.info("Log message goes here.")
logging.warning("Log message goes here.")
logging.error("Log message goes here.")
logging.critical("Log message goes here.")
By default, the logger doesn’t log the debug
and the info
level log messages. To enable them, we have to define a level for the logger. The following code does the same:
import logging
logging.basicConfig(level=logging.NOTSET) # Here
logging.debug("Log message goes here.")
logging.info("Log message goes here.")
logging.warning("Log message goes here.")
logging.error("Log message goes here.")
logging.critical("Log message goes here.")
If the level is set to INFO
, all the levels with seniority numbers greater than and equal to that of INFO
will only be printed. The following program depicts this process:
import logging
logging.basicConfig(level=logging.INFO) # Here
logging.debug("Log message goes here.")
logging.info("Log message goes here.")
logging.warning("Log message goes here.")
logging.error("Log message goes here.")
logging.critical("Log message goes here.")
The basicConfig()
method can be used to define the level. In total, the level
parameter can accept six values.
logging.DEBUG
# 10logging.INFO
# 20logging.WARNING
#30logging.ERROR
# 40logging.CRITICAL
# 50logging.NOTSET
# 0
There are more parameters that you can configure; you can read more about them here.
To learn about a few more, refer to this list:
filename
- ThebasicConfig()
method has afilename
parameter that can be used to set a file name, to which all the messages should be logged. When a file name is specified, the log messages are logged into this file instead of the console.filemode
- ThebasicConfig()
method has afilemode
parameter used to define the opening mode for the mentioned file. By default, it is set to"a"
, which meansappend
. So, every time you’ll run the program, new log messages will be appended to the old messages.format
- ThebasicConfig()
method has aformat
parameter that can be used to format the log messages structure. The default format, which looks like this:<Level>:root:<Message>
, will be overwritten by the new format.
If you want to read more about this library, refer here.