How to Use Post Request to Send Data to a Django Server
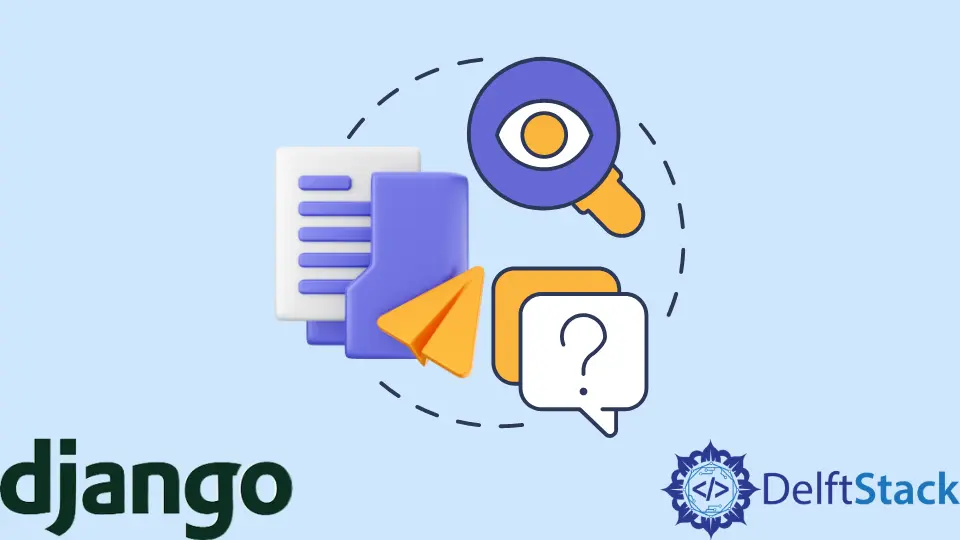
In this explanation about Django, we briefly introduce the post
and get
requests and how to implement the CSRF token with the post
in Django.
Use post
Request to Send Data to a Django Server
We use the HTTP protocol whenever we send a request or get a response. The HTTP has multiple methods like get
, post
, put
, delete
, etc., but we will focus on the two methods, get
and post
here.
When you visit a link to get the image, that is a get
request because you expect something from a server. If you want to send something to the server, maybe you are filling out a form and submitting your data; that is the post
method.
When we search the data using the search bar, the get
method will work behind the scene, and we can see the data on the address bar.
When we send data that could be passwords or other secret information, we will not use the get
method. We will be using the post
method on the backend.
The second reason to use the post
method is when we send multiple data of the entire form with lots of fields, and we do not want to show everything on the address bar. Technically, you should always use the post
method for submitting data.
The first step is to go to your form and set the method attribute as a post
. By default, the form uses the get
method.
<form action='add' method='post'>
Enter 1st number<input type="text" name='num1'><br>
Enter 2nd number<input type="text" name='num2'><br>
</form>
Let’s see if the post
method is working correctly. We expect you to try to use this code with a Django project, so run the server and go to your browser.
Output:
When we submit a form, we catch an error, and at least in the address bar, we do not have any data, but what is wrong with that? This error says CSRF verification failed.
The error is raised because several hacking techniques are available around the internet. The CSRF is a tag that will help us securely send our data.
But, we will not be talking much about this attack. You can search about CSRF.
Django has a built-in feature to avoid this error. If we go to the settings.py
file, we will find the MIDDLEWARE
list, and in this list, we can see there is already a given CSRF technique to use while sending data.
Using the Jinja code, we need to use that CSRF token in our form.
<form action='add' method='post'>
{% csrf_token %}
Enter 1st number<input type="text" name='num1'><br>
Enter 2nd number<input type="text" name='num2'><br>
</form>
We need to open the views.py
file and write this demo code.
def add(request):
val1 = int(request.POST["num1"])
val2 = int(request.POST["num2"])
res = val1 + val2
return render(request, "request.html", {"result": res})
So in this code, we are just summing two integers and sending the result on request.html
without getting an error.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn